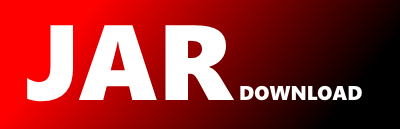
org.redisson.transaction.RedissonTransactionalMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of redisson Show documentation
Show all versions of redisson Show documentation
Redis Java client with features of In-Memory Data Grid
/**
* Copyright (c) 2013-2024 Nikita Koksharov
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.redisson.transaction;
import org.redisson.RedissonMap;
import org.redisson.ScanResult;
import org.redisson.api.*;
import org.redisson.api.mapreduce.RMapReduce;
import org.redisson.client.RedisClient;
import org.redisson.client.codec.Codec;
import org.redisson.command.CommandAsyncExecutor;
import org.redisson.transaction.operation.TransactionalOperation;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.atomic.AtomicBoolean;
/**
*
* @author Nikita Koksharov
*
* @param key type
* @param value type
*/
public class RedissonTransactionalMap extends RedissonMap {
private final BaseTransactionalMap transactionalMap;
private final AtomicBoolean executed;
public RedissonTransactionalMap(CommandAsyncExecutor commandExecutor,
List operations, long timeout, AtomicBoolean executed, RMap innerMap, String transactionId) {
super(innerMap.getCodec(), commandExecutor, innerMap.getName(), null, null, null);
this.executed = executed;
this.transactionalMap = new BaseTransactionalMap(commandExecutor, timeout, operations, innerMap, transactionId);
}
public RedissonTransactionalMap(CommandAsyncExecutor commandExecutor, String name,
List operations, long timeout, AtomicBoolean executed, String transactionId) {
super(commandExecutor, name, null, null, null);
this.executed = executed;
RedissonMap innerMap = new RedissonMap(commandExecutor, name, null, null, null);
this.transactionalMap = new BaseTransactionalMap(commandExecutor, timeout, operations, innerMap, transactionId);
}
public RedissonTransactionalMap(Codec codec, CommandAsyncExecutor commandExecutor, String name,
List operations, long timeout, AtomicBoolean executed, String transactionId) {
super(codec, commandExecutor, name, null, null, null);
this.executed = executed;
RedissonMap innerMap = new RedissonMap(codec, commandExecutor, name, null, null, null);
this.transactionalMap = new BaseTransactionalMap(commandExecutor, timeout, operations, innerMap, transactionId);
}
@Override
public RFuture expireAsync(long timeToLive, TimeUnit timeUnit, String param, String... keys) {
return transactionalMap.expireAsync(timeToLive, timeUnit, param, keys);
}
@Override
protected RFuture expireAtAsync(long timestamp, String param, String... keys) {
return transactionalMap.expireAtAsync(timestamp, param, keys);
}
@Override
public RFuture clearExpireAsync() {
return transactionalMap.clearExpireAsync();
}
@Override
public RFuture moveAsync(int database) {
throw new UnsupportedOperationException("move method is not supported in transaction");
}
@Override
public RFuture migrateAsync(String host, int port, int database, long timeout) {
throw new UnsupportedOperationException("migrate method is not supported in transaction");
}
@Override
public RMapReduce mapReduce() {
throw new UnsupportedOperationException("mapReduce method is not supported in transaction");
}
@Override
public ScanResult> scanIterator(String name, RedisClient client,
String startPos, String pattern, int count) {
checkState();
return transactionalMap.scanIterator(name, client, startPos, pattern, count);
}
@Override
public RFuture containsKeyAsync(Object key) {
checkState();
return transactionalMap.containsKeyAsync(key);
}
@Override
public RFuture containsValueAsync(Object value) {
checkState();
return transactionalMap.containsValueAsync(value);
}
@Override
protected RFuture addAndGetOperationAsync(K key, Number value) {
checkState();
return transactionalMap.addAndGetOperationAsync(key, value);
}
@Override
protected RFuture putIfExistsOperationAsync(K key, V value) {
checkState();
return transactionalMap.putIfExistsOperationAsync(key, value);
}
@Override
protected RFuture putIfAbsentOperationAsync(K key, V value) {
checkState();
return transactionalMap.putIfAbsentOperationAsync(key, value);
}
@Override
protected RFuture putOperationAsync(K key, V value) {
checkState();
return transactionalMap.putOperationAsync(key, value);
}
@Override
protected RFuture fastPutIfExistsOperationAsync(K key, V value) {
checkState();
return transactionalMap.fastPutIfExistsOperationAsync(key, value);
}
@Override
protected RFuture fastPutIfAbsentOperationAsync(K key, V value) {
checkState();
return transactionalMap.fastPutIfAbsentOperationAsync(key, value);
}
@Override
protected RFuture fastPutOperationAsync(K key, V value) {
checkState();
return transactionalMap.fastPutOperationAsync(key, value);
}
@Override
@SuppressWarnings("unchecked")
protected RFuture fastRemoveOperationAsync(K... keys) {
checkState();
return transactionalMap.fastRemoveOperationAsync(keys);
}
@Override
public RFuture valueSizeAsync(K key) {
checkState();
return transactionalMap.valueSizeAsync(key);
}
@Override
public RFuture getOperationAsync(K key) {
checkState();
return transactionalMap.getOperationAsync(key);
}
@Override
public RFuture> readAllKeySetAsync() {
checkState();
return transactionalMap.readAllKeySetAsync();
}
@Override
public RFuture>> readAllEntrySetAsync() {
checkState();
return transactionalMap.readAllEntrySetAsync();
}
@Override
public RFuture> readAllValuesAsync() {
checkState();
return transactionalMap.readAllValuesAsync();
}
@Override
public RFuture
© 2015 - 2025 Weber Informatics LLC | Privacy Policy