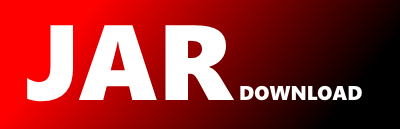
org.redisson.api.RSearchRx Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of redisson Show documentation
Show all versions of redisson Show documentation
Redis Java client with features of In-Memory Data Grid
/**
* Copyright (c) 2013-2024 Nikita Koksharov
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.redisson.api;
import io.reactivex.rxjava3.core.Completable;
import io.reactivex.rxjava3.core.Maybe;
import io.reactivex.rxjava3.core.Single;
import org.redisson.api.search.SpellcheckOptions;
import org.redisson.api.search.aggregate.AggregationOptions;
import org.redisson.api.search.aggregate.AggregationResult;
import org.redisson.api.search.index.FieldIndex;
import org.redisson.api.search.index.IndexInfo;
import org.redisson.api.search.index.IndexOptions;
import org.redisson.api.search.query.QueryOptions;
import org.redisson.api.search.query.SearchResult;
import java.util.List;
import java.util.Map;
/**
* RxJava3 API for RediSearch module
*
* @author Nikita Koksharov
*
*/
public interface RSearchRx {
/**
* Creates an index.
*
* Code example:
*
* search.create("idx", IndexOptions.defaults()
* .on(IndexType.HASH)
* .prefix(Arrays.asList("doc:")),
* FieldIndex.text("t1"),
* FieldIndex.tag("t2").withSuffixTrie());
*
*
* @param indexName index name
* @param options index options
* @param fields fields
*/
Completable createIndex(String indexName, IndexOptions options, FieldIndex... fields);
/**
* Executes search over defined index using defined query.
*
* Code example:
*
* SearchResult r = s.search("idx", "*", QueryOptions.defaults()
* .returnAttributes(new ReturnAttribute("t1"), new ReturnAttribute("t2")));
*
*
* @param indexName index name
* @param query query value
* @param options query options
* @return search result
*/
Single search(String indexName, String query, QueryOptions options);
/**
* Executes aggregation over defined index using defined query.
*
* Code example:
*
* AggregationResult r = s.aggregate("idx", "*", AggregationOptions.defaults()
* .load("t1", "t2"));
*
*
* @param indexName index name
* @param query query value
* @param options aggregation options
* @return aggregation result
*/
Single aggregate(String indexName, String query, AggregationOptions options);
/**
* Adds alias to defined index name
*
* @param alias alias value
* @param indexName index name
*/
Completable addAlias(String alias, String indexName);
/**
* Deletes index alias
*
* @param alias alias value
*/
Completable delAlias(String alias);
/**
* Adds alias to defined index name.
* Re-assigns the alias if it was used before with a different index.
*
* @param alias alias value
* @param indexName index name
*/
Completable updateAlias(String alias, String indexName);
/**
* Adds a new attribute to the index
*
* @param indexName index name
* @param skipInitialScan doesn't scan the index if true
* @param fields field indexes
*/
Completable alter(String indexName, boolean skipInitialScan, FieldIndex... fields);
/**
* Returns configuration map by defined parameter name
*
* @param parameter parameter name
* @return configuration map
*/
Maybe
© 2015 - 2024 Weber Informatics LLC | Privacy Policy