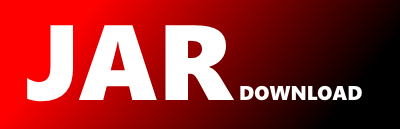
org.redisson.api.RSetCacheRx Maven / Gradle / Ivy
Show all versions of redisson Show documentation
/**
* Copyright (c) 2013-2024 Nikita Koksharov
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.redisson.api;
import java.time.Duration;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.TimeUnit;
import io.reactivex.rxjava3.core.Single;
/**
* RxJava2 interface for RSetCache object
*
* @author Nikita Koksharov
*
* @param value
*/
public interface RSetCacheRx extends RCollectionRx, RDestroyable {
/**
* Returns RPermitExpirableSemaphore
instance associated with value
*
* @param value - set value
* @return RPermitExpirableSemaphore object
*/
RPermitExpirableSemaphoreRx getPermitExpirableSemaphore(V value);
/**
* Returns RSemaphore
instance associated with value
*
* @param value - set value
* @return RSemaphore object
*/
RSemaphoreRx getSemaphore(V value);
/**
* Returns RLock
instance associated with value
*
* @param value - set value
* @return RLock object
*/
RLockRx getFairLock(V value);
/**
* Returns RReadWriteLock
instance associated with value
*
* @param value - set value
* @return RReadWriteLock object
*/
RReadWriteLockRx getReadWriteLock(V value);
/**
* Returns lock instance associated with value
*
* @param value - set value
* @return RLock object
*/
RLockRx getLock(V value);
/**
* Stores value with specified time to live.
* Value expires after specified time to live.
*
* @param value to add
* @param ttl - time to live for key\value entry.
* If 0
then stores infinitely.
* @param unit - time unit
* @return true
if value has been added. false
* if value already been in collection.
*/
Single add(V value, long ttl, TimeUnit unit);
/**
* Returns the number of elements in cache.
* This number can reflects expired elements too
* due to non realtime cleanup process.
*
*/
@Override
Single size();
/**
* Read all elements at once
*
* @return values
*/
Single> readAll();
/**
* Tries to add elements only if none of them in set.
*
* @param values - values to add
* @return true
if elements successfully added,
* otherwise false
.
*/
Single tryAdd(V... values);
/**
* Use {@link #addIfAbsent(Map)} instead
*
* @param values - values to add
* @param ttl - time to live for value.
* If 0
then stores infinitely.
* @param unit - time unit
* @return true
if elements successfully added,
* otherwise false
.
*/
@Deprecated
Single tryAdd(long ttl, TimeUnit unit, V... values);
/**
* Adds elements to this set only if all of them haven't been added before.
*
* Requires Redis 3.0.2 and higher.
*
* @param objects map of elements to add
* @return true
if elements added and false
if not.
*/
Single addIfAbsent(Map objects);
/**
* Adds element to this set only if has not been added before.
*
* Requires Redis 3.0.2 and higher.
*
* @param ttl - object ttl
* @param object - object itself
* @return true
if element added and false
if not.
*/
Single addIfAbsent(Duration ttl, V object);
/**
* Adds element to this set only if it's already exists.
*
* Requires Redis 3.0.2 and higher.
*
* @param ttl - object ttl
* @param object - object itself
* @return true
if element added and false
if not.
*/
Single addIfExists(Duration ttl, V object);
/**
* Adds element to this set only if new ttl less than current ttl of existed element.
*
* Requires Redis 6.2.0 and higher.
*
* @param ttl - object ttl
* @param object - object itself
* @return true
if element added and false
if not.
*/
Single addIfLess(Duration ttl, V object);
/**
* Adds element to this set only if new ttl greater than current ttl of existed element.
*
* Requires Redis 6.2.0 and higher.
*
* @param ttl - object ttl
* @param object - object itself
* @return true
if element added and false
if not.
*/
Single addIfGreater(Duration ttl, V object);
/**
* Adds all elements contained in the specified map to this sorted set.
* Map contains of ttl mapped by object.
*
* @param objects - map of elements to add
* @return amount of added elements, not including already existing in this sorted set
*/
Single addAll(Map objects);
/**
* Adds elements to this set only if they haven't been added before.
*
* Requires Redis 3.0.2 and higher.
*
* @param objects map of elements to add
* @return amount of added elements
*/
Single addAllIfAbsent(Map objects);
/**
* Adds elements to this set only if they already exist.
*
* Requires Redis 3.0.2 and higher.
*
* @param objects map of elements to add
* @return amount of added elements
*/
Single addAllIfExist(Map objects);
/**
* Adds elements to this set only if new ttl greater than current ttl of existed elements.
*
* Requires Redis 6.2.0 and higher.
*
* @param objects map of elements to add
* @return amount of added elements
*/
Single addAllIfGreater(Map objects);
/**
* Adds elements to this set only if new ttl less than current ttl of existed elements.
*
* Requires Redis 6.2.0 and higher.
*
* @param objects map of elements to add
* @return amount of added elements
*/
Single addAllIfLess(Map objects);
/**
* Adds object event listener
*
* @see org.redisson.api.listener.TrackingListener
* @see org.redisson.api.listener.SetAddListener
* @see org.redisson.api.listener.SetRemoveListener
* @see org.redisson.api.ExpiredObjectListener
* @see org.redisson.api.DeletedObjectListener
*
* @param listener - object event listener
* @return listener id
*/
Single addListener(ObjectListener listener);
}