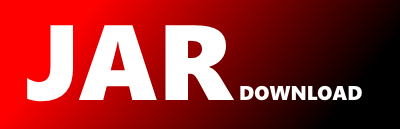
org.redisson.api.RLexSortedSetRx Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of redisson Show documentation
Show all versions of redisson Show documentation
Redis Java client with features of In-Memory Data Grid
/**
* Copyright (c) 2013-2024 Nikita Koksharov
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.redisson.api;
import java.util.Collection;
import io.reactivex.rxjava3.core.Single;
/**
* RxJava2 interface for sorted set contained values of String type.
*
* @author Nikita Koksharov
*
*/
public interface RLexSortedSetRx extends RScoredSortedSetRx, RCollectionRx {
/**
* Removes values range starting with fromElement
and ending with toElement
.
*
* @param fromElement - start element
* @param fromInclusive - start element inclusive
* @param toElement - end element
* @param toInclusive - end element inclusive
* @return number of elements removed
*/
Single removeRange(String fromElement, boolean fromInclusive, String toElement, boolean toInclusive);
/**
* Removes tail values range starting with fromElement
.
*
* @param fromElement - start element
* @param fromInclusive - start element inclusive
* @return number of elements removed
*/
Single removeRangeTail(String fromElement, boolean fromInclusive);
/**
* Removes head values range ending with toElement
.
*
* @param toElement - end element
* @param toInclusive - end element inclusive
* @return number of elements removed
*/
Single removeRangeHead(String toElement, boolean toInclusive);
/**
* Returns the number of tail values starting with fromElement
.
*
* @param fromElement - start element
* @param fromInclusive - start element inclusive
* @return number of elements
*/
Single countTail(String fromElement, boolean fromInclusive);
/**
* Returns the number of head values ending with toElement
.
*
* @param toElement - end element
* @param toInclusive - end element inclusive
* @return number of elements
*/
Single countHead(String toElement, boolean toInclusive);
/**
* Returns tail values range starting with fromElement
.
*
* @param fromElement - start element
* @param fromInclusive - start element inclusive
* @return collection of elements
*/
Single> rangeTail(String fromElement, boolean fromInclusive);
/**
* Returns head values range ending with toElement
.
*
* @param toElement - end element
* @param toInclusive - end element inclusive
* @return collection of elements
*/
Single> rangeHead(String toElement, boolean toInclusive);
/**
* Returns values range starting with fromElement
and ending with toElement
.
*
* @param fromElement - start element
* @param fromInclusive - start element inclusive
* @param toElement - end element
* @param toInclusive - end element inclusive
* @return collection of elements
*/
Single> range(String fromElement, boolean fromInclusive, String toElement, boolean toInclusive);
/**
* Returns tail values range starting with fromElement
.
* Returned collection limited by count
and starts with offset
.
*
* @param fromElement - start element
* @param fromInclusive - start element inclusive
* @param offset - offset of result collection
* @param count - amount of result collection
* @return collection of elements
*/
Single> rangeTail(String fromElement, boolean fromInclusive, int offset, int count);
/**
* Returns head values range ending with toElement
.
* Returned collection limited by count
and starts with offset
.
*
* @param toElement - end element
* @param toInclusive - end element inclusive
* @param offset - offset of result collection
* @param count - amount of result collection
* @return collection of elements
*/
Single> rangeHead(String toElement, boolean toInclusive, int offset, int count);
/**
* Returns values range starting with fromElement
and ending with toElement
.
* Returned collection limited by count
and starts with offset
.
*
* @param fromElement - start element
* @param fromInclusive - start element inclusive
* @param toElement - end element
* @param toInclusive - end element inclusive
* @param offset - offset of result collection
* @param count - amount of result collection
* @return collection of elements
*/
Single> range(String fromElement, boolean fromInclusive, String toElement, boolean toInclusive, int offset, int count);
/**
* Returns the number of elements between fromElement
and toElement
.
*
* @param fromElement - start element
* @param fromInclusive - start element inclusive
* @param toElement - end element
* @param toInclusive - end element inclusive
* @return number of elements
*/
Single count(String fromElement, boolean fromInclusive, String toElement, boolean toInclusive);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy