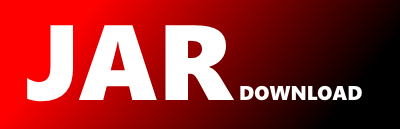
org.redisson.api.RListRx Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of redisson Show documentation
Show all versions of redisson Show documentation
Redis Java client with features of In-Memory Data Grid
/**
* Copyright (c) 2013-2024 Nikita Koksharov
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.redisson.api;
import java.util.Collection;
import java.util.List;
import io.reactivex.rxjava3.core.Completable;
import io.reactivex.rxjava3.core.Flowable;
import io.reactivex.rxjava3.core.Maybe;
import io.reactivex.rxjava3.core.Single;
/**
* list functions
*
* @author Nikita Koksharov
*
* @param the type of elements held in this collection
*/
// TODO add sublist support
public interface RListRx extends RCollectionRx, RSortableRx> {
/**
* Loads elements by specified indexes
*
* @param indexes of elements
* @return elements
*/
Single> get(int... indexes);
/**
* Add element
after elementToFind
*
* @param elementToFind - object to find
* @param element - object to add
* @return new list size
*/
Single addAfter(V elementToFind, V element);
/**
* Add element
before elementToFind
*
* @param elementToFind - object to find
* @param element - object to add
* @return new list size
*/
Single addBefore(V elementToFind, V element);
Flowable descendingIterator();
Flowable descendingIterator(int startIndex);
Flowable iterator(int startIndex);
/**
* Returns last index of element
or
* -1 if element isn't found
*
* @param element to find
* @return index of -1 if element isn't found
*/
Single lastIndexOf(Object element);
/**
* Returns last index of element
or
* -1 if element isn't found
*
* @param element to find
* @return index of -1 if element isn't found
*/
Single indexOf(Object element);
/**
* Inserts element
at index
.
* Subsequent elements are shifted.
*
* @param index - index number
* @param element - element to insert
* @return {@code true} if list was changed
*/
Completable add(int index, V element);
/**
* Inserts elements
at index
.
* Subsequent elements are shifted.
*
* @param index - index number
* @param elements - elements to insert
* @return {@code true} if list changed
* or {@code false} if element isn't found
*/
Single addAll(int index, Collection extends V> elements);
/**
* Set element
at index
.
* Works faster than {@link #set(int, Object)} but
* doesn't return previous element.
*
* @param index - index of object
* @param element - object
* @return void
*/
Completable fastSet(int index, V element);
/**
* Set element
at index
and returns previous element.
*
* @param index - index of object
* @param element - object
* @return previous element or null
if element wasn't set.
*/
Maybe set(int index, V element);
/**
* Get element at index
*
* @param index - index of object
* @return element
*/
Maybe get(int index);
/**
* Removes element at index
.
*
* @param index - index of object
* @return element or null
if element wasn't set.
*/
Maybe remove(int index);
/**
* Read all elements at once
*
* @return list of values
*/
Single> readAll();
/**
* Trim list and remains elements only in specified range
* fromIndex
, inclusive, and toIndex
, inclusive.
*
* @param fromIndex - from index
* @param toIndex - to index
* @return void
*/
Completable trim(int fromIndex, int toIndex);
/**
* Remove object by specified index
*
* @param index - index of object
* @return void
*/
Completable fastRemove(int index);
/**
* Returns range of values from 0 index to toIndex
. Indexes are zero based.
* -1
means the last element, -2
means penultimate and so on.
*
* @param toIndex - end index
* @return elements
*/
Single> range(int toIndex);
/**
* Returns range of values from fromIndex
to toIndex
index including.
* Indexes are zero based. -1
means the last element, -2
means penultimate and so on.
*
* @param fromIndex - start index
* @param toIndex - end index
* @return elements
*/
Single> range(int fromIndex, int toIndex);
/**
* Adds object event listener
*
* @see org.redisson.api.listener.TrackingListener
* @see org.redisson.api.ExpiredObjectListener
* @see org.redisson.api.DeletedObjectListener
* @see org.redisson.api.listener.ListAddListener
* @see org.redisson.api.listener.ListInsertListener
* @see org.redisson.api.listener.ListSetListener
* @see org.redisson.api.listener.ListRemoveListener
* @see org.redisson.api.listener.ListTrimListener
*
* @param listener - object event listener
* @return listener id
*/
Single addListener(ObjectListener listener);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy