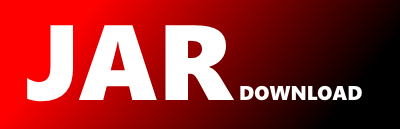
org.redisson.reactive.RedissonListMultimapReactive Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of redisson Show documentation
Show all versions of redisson Show documentation
Redis Java client with features of In-Memory Data Grid
/**
* Copyright 2016 Nikita Koksharov
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.redisson.reactive;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.UUID;
import java.util.concurrent.TimeUnit;
import org.reactivestreams.Publisher;
import org.redisson.RedissonListMultimap;
import org.redisson.api.RListMultimapReactive;
import org.redisson.api.RListReactive;
import org.redisson.client.codec.Codec;
import org.redisson.client.protocol.RedisCommands;
import org.redisson.command.CommandReactiveExecutor;
import io.netty.buffer.ByteBuf;
/**
*
* @author Nikita Koksharov
*
* @param key type
* @param value type
*/
public class RedissonListMultimapReactive extends RedissonBaseMultimapReactive implements RListMultimapReactive {
public RedissonListMultimapReactive(UUID id, CommandReactiveExecutor commandExecutor, String name) {
super(new RedissonListMultimap(id, commandExecutor, name), commandExecutor, name);
}
public RedissonListMultimapReactive(UUID id, Codec codec, CommandReactiveExecutor commandExecutor, String name) {
super(new RedissonListMultimap(id, codec, commandExecutor, name), codec, commandExecutor, name);
}
@Override
public RListReactive get(final K key) {
final ByteBuf keyState = encodeMapKey(key);
final String keyHash = hashAndRelease(keyState);
final String setName = getValuesName(keyHash);
return new RedissonListReactive(codec, commandExecutor, setName) {
@Override
public Publisher delete() {
ByteBuf keyState = encodeMapKey(key);
return RedissonListMultimapReactive.this.fastRemove(Arrays.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy