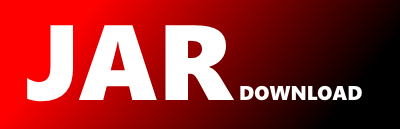
org.redisson.reactive.RedissonMapReactive Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of redisson Show documentation
Show all versions of redisson Show documentation
Redis Java client with features of In-Memory Data Grid
/**
* Copyright 2016 Nikita Koksharov
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.redisson.reactive;
import java.net.InetSocketAddress;
import java.util.Collection;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import java.util.function.BiFunction;
import java.util.function.Function;
import java.util.function.Supplier;
import org.reactivestreams.Publisher;
import org.redisson.RedissonMap;
import org.redisson.api.MapOptions;
import org.redisson.api.RFuture;
import org.redisson.api.RMapAsync;
import org.redisson.api.RMapReactive;
import org.redisson.client.RedisClient;
import org.redisson.client.codec.Codec;
import org.redisson.client.codec.MapScanCodec;
import org.redisson.client.protocol.RedisCommands;
import org.redisson.client.protocol.decoder.MapScanResult;
import org.redisson.client.protocol.decoder.ScanObjectEntry;
import org.redisson.command.CommandReactiveExecutor;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
/**
* Distributed and concurrent implementation of {@link java.util.concurrent.ConcurrentMap}
* and {@link java.util.Map}
*
* @author Nikita Koksharov
*
* @param key
* @param value
*/
public class RedissonMapReactive extends RedissonExpirableReactive implements RMapReactive, MapReactive {
private final RMapAsync instance;
public RedissonMapReactive(CommandReactiveExecutor commandExecutor, String name, MapOptions options) {
super(commandExecutor, name);
instance = new RedissonMap(codec, commandExecutor, name, null, options);
}
public RedissonMapReactive(Codec codec, CommandReactiveExecutor commandExecutor, String name, MapOptions options) {
super(codec, commandExecutor, name);
instance = new RedissonMap(codec, commandExecutor, name, null, options);
}
@Override
public Publisher loadAll(final boolean replaceExistingValues, final int parallelism) {
return reactive(new Supplier>() {
@Override
public RFuture get() {
return instance.loadAllAsync(replaceExistingValues, parallelism);
}
});
}
@Override
public Publisher loadAll(final Set extends K> keys, final boolean replaceExistingValues, final int parallelism) {
return reactive(new Supplier>() {
@Override
public RFuture get() {
return instance.loadAllAsync(keys, replaceExistingValues, parallelism);
}
});
}
@Override
public Publisher fastPutIfAbsent(final K key, final V value) {
return reactive(new Supplier>() {
@Override
public RFuture get() {
return instance.fastPutIfAbsentAsync(key, value);
}
});
}
@Override
public Publisher> readAllKeySet() {
return reactive(new Supplier>>() {
@Override
public RFuture> get() {
return instance.readAllKeySetAsync();
}
});
}
@Override
public Publisher> readAllValues() {
return reactive(new Supplier>>() {
@Override
public RFuture> get() {
return instance.readAllValuesAsync();
}
});
}
@Override
public Publisher>> readAllEntrySet() {
return reactive(new Supplier>>>() {
@Override
public RFuture>> get() {
return instance.readAllEntrySetAsync();
}
});
}
@Override
public Publisher
© 2015 - 2025 Weber Informatics LLC | Privacy Policy