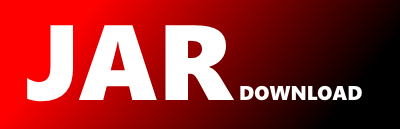
org.redkalex.convert.protobuf.ProtobufEnumSimpledCoder Maven / Gradle / Ivy
Show all versions of redkale-plugins Show documentation
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package org.redkalex.convert.protobuf;
import java.lang.reflect.Method;
import java.util.*;
import org.redkale.convert.*;
/**
* 枚举 的SimpledCoder实现
*
*
* 详情见: https://redkale.org
*
* @author zhangjx
* @param Reader输入的子类型
* @param Writer输出的子类型
* @param Enum的子类
*/
public class ProtobufEnumSimpledCoder extends SimpledCoder {
private final Map values1 = new HashMap<>();
private final Map values2 = new HashMap<>();
private final boolean enumtostring;
public ProtobufEnumSimpledCoder(Class type, boolean enumtostring) {
this.type = type;
this.enumtostring = enumtostring;
try {
final Method method = type.getMethod("values");
int index = -1;
for (E item : (E[]) method.invoke(null)) {
values1.put(item, ++index);
values2.put(index, item);
}
} catch (Exception e) {
throw new RuntimeException(e);
}
}
@Override
public void convertTo(final W out, final E value) {
if (value == null) {
out.writeNull();
} else if (enumtostring) {
out.writeSmallString(value.toString());
} else {
((ProtobufWriter) out).writeUInt32(values1.getOrDefault(value, -1));
}
}
@Override
@SuppressWarnings("unchecked")
public E convertFrom(final R in) {
if (enumtostring) {
String value = in.readSmallString();
if (value == null) return null;
return (E) Enum.valueOf((Class) type, value);
}
int value = ((ProtobufReader) in).readRawVarint32();
if (value == -1) return null;
return values2.get(value);
}
@Override
public Class getType() {
return (Class) type;
}
}