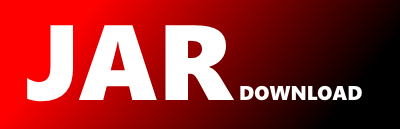
org.refcodes.audio.CsvMonoSampleWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-audio Show documentation
Show all versions of refcodes-audio Show documentation
Artifact providing audio provessing functionality such as generating
sine waves or writing raw audio samples to WAV files.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.audio;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.OutputStream;
import java.io.PrintStream;
/**
* The {@link CsvMonoSampleWriter} provides means to write sound samples to a
* CSV file.
*/
public class CsvMonoSampleWriter extends AbstractCsvSampleWriter implements MonoSampleWriter {
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private final MonoSampleBuilder _soundSample = new MonoSampleBuilderImpl( 0, SamplingRate.AUDIO_CD.getSamplesPerSecond() );
private boolean _hasHeader = false;
private boolean _isSampleDataDirty = true;
private boolean _isSamplingRateDirty = true;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Constructs the {@link CsvMonoSampleWriter} for writing sound samples to a
* CSV file or stream.
*
* @param aFile The {@link File} where to write the CSV records to.
*
* @throws FileNotFoundException If the given file object does not denote an
* existing, writable regular file and a new regular file of that
* name cannot be created, or if some other error occurs while
* opening or creating the file.
*/
public CsvMonoSampleWriter( File aFile ) throws FileNotFoundException {
super( aFile );
}
/**
* Constructs the {@link CsvMonoSampleWriter} for writing sound samples to a
* CSV file or stream.
*
* @param aOutputStream The {@link OutputStream} where to write the CSV
* records to.
*/
public CsvMonoSampleWriter( OutputStream aOutputStream ) {
super( aOutputStream );
}
/**
* Constructs the {@link CsvMonoSampleWriter} for writing sound samples to a
* CSV file or stream.
*
* @param aPrintStream The {@link PrintStream} where to write the CSV
* records to.
*/
public CsvMonoSampleWriter( PrintStream aPrintStream ) {
super( aPrintStream );
}
/**
* Constructs the {@link CsvMonoSampleWriter} for writing sound samples to a
* CSV file or stream.
*
* @param aFile The {@link File} where to write the CSV records to.
* @param aDeltaMode The {@link CsvDeltaMode} to use when writing the CSV
* rows.
*
* @throws FileNotFoundException If the given file object does not denote an
* existing, writable regular file and a new regular file of that
* name cannot be created, or if some other error occurs while
* opening or creating the file.
*/
public CsvMonoSampleWriter( File aFile, CsvDeltaMode aDeltaMode ) throws FileNotFoundException {
super( aFile, aDeltaMode );
}
/**
* Constructs the {@link CsvMonoSampleWriter} for writing sound samples to a
* CSV file or stream.
*
* @param aOutputStream The {@link OutputStream} where to write the CSV
* records to.
* @param aDeltaMode The {@link CsvDeltaMode} to use when writing the CSV
* rows.
*/
public CsvMonoSampleWriter( OutputStream aOutputStream, CsvDeltaMode aDeltaMode ) {
super( aOutputStream, aDeltaMode );
}
/**
* Constructs the {@link CsvMonoSampleWriter} for writing sound samples to a
* CSV file or stream.
*
* @param aPrintStream The {@link PrintStream} where to write the CSV
* records to.
* @param aDeltaMode The {@link CsvDeltaMode} to use when writing the CSV
* rows.
*/
public CsvMonoSampleWriter( PrintStream aPrintStream, CsvDeltaMode aDeltaMode ) {
super( aPrintStream, aDeltaMode );
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public void writeNext( double aSampleData ) {
doUpdateSampleData( aSampleData );
_soundSample.updateTimeStamp();
writeNext( _soundSample );
}
/**
* {@inheritDoc}
*/
@Override
public void writeNext( MonoSample aSample ) {
final double theSample = aSample.getMonoData();
final String[] theRow = new String[1 + 3];
// Header |-->
if ( !_hasHeader ) {
synchronized ( this ) {
if ( !_hasHeader ) {
theRow[0] = HEADER_INDEX;
theRow[1] = HEADER_TIME_STAMP;
theRow[2] = HEADER_SAMPLE_DATA;
theRow[theRow.length - 1] = HEADER_SAMPLING_RATE;
_csvWriter.writeHeader( theRow );
_hasHeader = true;
}
}
}
// Header <--|
if ( aSample != _soundSample ) {
doUpdateSampleData( aSample.getMonoData() );
if ( aSample.getSamplingRate() != -1 && aSample.getSamplingRate() != _soundSample.getSamplingRate() ) {
_soundSample.setSamplingRate( aSample.getSamplingRate() );
_isSamplingRateDirty = true;
}
if ( aSample.getIndex() != -1 ) {
_soundSample.setIndex( aSample.getIndex() );
}
if ( aSample.getTimeStamp() != -1 ) {
_soundSample.setTimeStamp( aSample.getTimeStamp() );
}
}
theRow[0] = Long.toString( _soundSample.getIndex() );
theRow[1] = toString( _soundSample.getTimeStamp() );
if ( !_deltaMode.isSampleDataDelta() || _isSampleDataDirty ) {
theRow[2] = toString( theSample );
}
if ( !_deltaMode.isSamplingRateDelta() || _isSamplingRateDirty ) {
theRow[theRow.length - 1] = Integer.toString( _soundSample.getSamplingRate() );
}
_csvWriter.writeNext( theRow );
_soundSample.increaseIndex();
_isSampleDataDirty = false;
_isSamplingRateDirty = false;
}
/**
* {@inheritDoc}
*/
@Override
public int getSamplingRate() {
return _soundSample.getSamplingRate();
}
/**
* {@inheritDoc}
*/
@Override
public void setSamplingRate( int aSamplingRate ) {
if ( aSamplingRate != -1 && aSamplingRate != _soundSample.getSamplingRate() ) {
_soundSample.setSamplingRate( aSamplingRate );
_isSamplingRateDirty = true;
}
}
/**
* {@inheritDoc}
*/
@Override
public CsvMonoSampleWriter withSamplingRate( int aSamplingRate ) {
setSamplingRate( aSamplingRate );
return this;
}
// /////////////////////////////////////////////////////////////////////////
// HELPER:
// /////////////////////////////////////////////////////////////////////////
/**
* Updates the sample data alongside the according dirty flags.
*
* @param aSampleData The sample data with which to update this instance's
* sample data.
*/
private void doUpdateSampleData( double aSampleData ) {
final double theSampleData = _soundSample.getMonoData();
if ( theSampleData != aSampleData ) {
_isSampleDataDirty = true;
}
_soundSample.setMonoData( aSampleData );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy