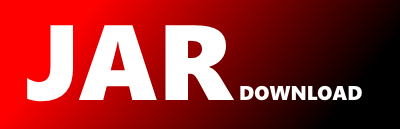
org.refcodes.audio.CurveFunctionSoundSampleBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-audio Show documentation
Show all versions of refcodes-audio Show documentation
Artifact providing audio provessing functionality such as generating
sine waves or writing raw audio samples to WAV files.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.audio;
import java.util.function.Function;
import org.refcodes.audio.SamplingRateAccessor.SamplingRateBuilder;
import org.refcodes.audio.SamplingRateAccessor.SamplingRateProperty;
/**
* The {@link CurveFunctionSoundSampleBuilder} makes it easy to create sound
* samples for some (trigonometric) functions. The provided function should
* return double values between -1 and 1.
*/
public interface CurveFunctionSoundSampleBuilder extends SamplingRateProperty, SamplingRateBuilder {
/**
* Sets the trigonometric function to be used when generating
* {@link MonoSample}.
*
* @param aFunction The {@link Function} to be set.
*/
void setTrigonometricFunction( Function aFunction );
/**
* Returns the trigonometric function being used when generating
* {@link MonoSample} instances.
*
* @return The {@link Function} being set.
*/
Function getTrigonometricFunction();
/**
* Builder method for setting the trigonometric function to be used when
* generating {@link MonoSample} instances.
*
* @param aFunction The {@link Function} to be set.
*
* @return This {@link CurveFunctionSoundSampleBuilder} instance for
* chaining multiple builder operations.
*/
default CurveFunctionSoundSampleBuilder withTrigonometricFunction( Function aFunction ) {
setTrigonometricFunction( aFunction );
return this;
}
/**
* Sets the trigonometric function to be used when generating
* {@link MonoSample}.
*
* @param aFunction The {@link CurveFunctionFunction} to be set.
*/
default void setTrigonometricFunction( CurveFunctionFunction aFunction ) {
setTrigonometricFunction( aFunction.getFunction() );
}
/**
* Builder method for setting the trigonometric function to be used when
* generating {@link MonoSample} instances.
*
* @param aFunction The {@link CurveFunctionFunction} to be set.
*
* @return This {@link CurveFunctionSoundSampleBuilder} instance for
* chaining multiple builder operations.
*/
default CurveFunctionSoundSampleBuilder withTrigonometricFunction( CurveFunctionFunction aFunction ) {
setTrigonometricFunction( aFunction.getFunction() );
return this;
}
/**
* Sets the index to be used when generating {@link MonoSample} instances.
*
* @param aIndex The index to be set.
*/
void setIndex( int aIndex );
/**
* Returns the index being used when generating {@link MonoSample}
* instances.
*
* @return The index being set.
*/
int getIndex();
/**
* Builder method for setting the index to be used when generating
* {@link MonoSample} instances.
*
* @param aIndex The index to be set.
*
* @return This {@link CurveFunctionSoundSampleBuilder} instance for
* chaining multiple builder operations.
*/
default CurveFunctionSoundSampleBuilder withIndex( int aIndex ) {
setIndex( aIndex );
return this;
}
/**
* Sets the frequency in Hz to be used when generating {@link MonoSample}
* instances.
*
* @param aFrequencyInHz The frequency to be set.
*/
void setFrequency( double aFrequencyInHz );
/**
* Returns the frequency in Hz being used when generating {@link MonoSample}
* instances.
*
* @return The frequency in Hz being set.
*/
double getFrequency();
/**
* Builder method for setting the frequency in Hz to be used when generating
* {@link MonoSample} instances.
*
* @param aFrequencyInHz The frequency in Hz to be set.
*
* @return This {@link CurveFunctionSoundSampleBuilder} instance for
* chaining multiple builder operations.
*/
default CurveFunctionSoundSampleBuilder withFrequency( double aFrequencyInHz ) {
setFrequency( aFrequencyInHz );
return this;
}
/**
* Sets the amplitude to be used when generating {@link MonoSample}
* instances.
*
* @param aAmplitude The amplitude to be set.
*/
void setAmplitude( double aAmplitude );
/**
* Returns the amplitude being used when generating {@link MonoSample}
* instances.
*
* @return The amplitude being set.
*/
double getAmplitude();
/**
* Builder method for setting the amplitude to be used when generating
* {@link MonoSample} instances.
*
* @param aAmplitude The amplitude to be set.
*
* @return This {@link CurveFunctionSoundSampleBuilder} instance for
* chaining multiple builder operations.
*/
default CurveFunctionSoundSampleBuilder withAmplitude( double aAmplitude ) {
setAmplitude( aAmplitude );
return this;
}
/**
* Sets the x-offset to be used when generating {@link MonoSample}
* instances.
*
* @param aXOffset The x-offset to be set.
*/
void setXOffset( int aXOffset );
/**
* Returns the x-offset being used when generating {@link MonoSample}
* instances.
*
* @return The x-offset being set.
*/
int getXOffset();
/**
* Builder method for setting the x-offset to be used when generating
* {@link MonoSample} instances.
*
* @param aXOffset The x-offset to be set.
*
* @return This {@link CurveFunctionSoundSampleBuilder} instance for
* chaining multiple builder operations.
*/
default CurveFunctionSoundSampleBuilder withXOffset( int aXOffset ) {
setXOffset( aXOffset );
return this;
}
/**
* Sets the y-offset to be used when generating {@link MonoSample}
* instances.
*
* @param aYOffset The y-offset to be set.
*/
void setYOffset( double aYOffset );
/**
* Returns the yOffset being used when generating {@link MonoSample}
* instances.
*
* @return The yOffset being set.
*/
double getYOffset();
/**
* Builder method for setting the y-offset to be used when generating
* {@link MonoSample} instances.
*
* @param aYOffset The y-offset to be set.
*
* @return This {@link CurveFunctionSoundSampleBuilder} instance for
* chaining multiple builder operations.
*/
default CurveFunctionSoundSampleBuilder withYOffset( double aYOffset ) {
setYOffset( aYOffset );
return this;
}
/**
* Builder method for setting the sampling rate to be used when generating
* {@link MonoSample} instances.
*
* @param aSamplingRate The sampling rate to be set.
*
* @return This {@link CurveFunctionSoundSampleBuilder} instance for
* chaining multiple builder operations.
*/
@Override
default CurveFunctionSoundSampleBuilder withSamplingRate( int aSamplingRate ) {
setSamplingRate( aSamplingRate );
return null;
}
/**
* Returns the next {@link MonoSample} for the current index (as of
* {@link #getIndex()}) as well as for the other builder's settings and
* increases the index by one.
*
* @return The next {@link MonoSample} being set up.
*/
MonoSample next();
/**
* Creates a {@link MonoSample} as of the builder's settings.
*
* @return The sound sample as of the builder's settings.
*/
MonoSample toMonoSample();
/**
* Creates a {@link MonoSample} for the given index and the the builder's
* settings.
*
* @param aIndex The index for which to create the sound sample.
*
* @return The sound sample as of the index and the builder's settings.
*/
MonoSample toMonoSample( int aIndex );
/**
* Creates a {@link MonoSample} for the given arguments and the given
* function. Thanks to willemsenzo, May 18, 2017:
* return Mathf.Sin(2 * Mathf.PI * timeIndex * frequency / sampleRate);
* See "https://forum.unity.com/threads/generating-a-simple-sinewave.471529"
*
* @param aIndex The index of the sample to generate.
* @param aFunction The function to be applied (sine, cosine, ...).
* @param aFrequencyHz The frequency (Hz) to use.
* @param aAmplitude The maximum amplitude to produce.
* @param aXOffset The x-offset (in samples) to be used.
* @param aYOffset The y-offset for the amplitude to be used.
* @param aSamplingRate The samples per second to generate.
*
* @return The {@link MonoSample} generated from the given input values.
*/
static MonoSampleBuilder asMonoSample( double aIndex, Function aFunction, double aFrequencyHz, double aAmplitude, int aXOffset, double aYOffset, int aSamplingRate ) {
final double theTime = aIndex / ( (double) aSamplingRate );
double theSample = aFunction.apply( 2 * Math.PI * ( aIndex - aXOffset ) * aFrequencyHz / aSamplingRate );
theSample *= aAmplitude;
theSample += aYOffset;
return new MonoSampleBuilderImpl( theTime, theSample );
}
/**
* This is a convenience method for easily instantiating the according
* builder.
*
* @return an instance (using a default implementation) of this builder
*/
static CurveFunctionSoundSampleBuilder build() {
return new CurveFunctionSoundSampleBuilderImpl();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy