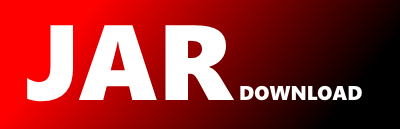
org.refcodes.audio.MonoSampleBuilderImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-audio Show documentation
Show all versions of refcodes-audio Show documentation
Artifact providing audio provessing functionality such as generating
sine waves or writing raw audio samples to WAV files.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.audio;
import java.util.Arrays;
/**
* A {@link MonoSampleBuilderImpl} represents a single amplitude (one for the
* mono channel) assigned to an according time positioning.
*/
public class MonoSampleBuilderImpl implements MonoSampleBuilder {
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private double _timeStamp = -1;
private int _sampligRate = -1;
private double[] _sampleData = new double[1];
private long _index = -1;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Constructs a {@link MonoSample} instance with the according sound
* sample's settings
*
* @param aSample The sample (set of values representing the channels, one
* channel per value) for the related time positioning.
*/
public MonoSampleBuilderImpl( MonoSample aSample ) {
_index = aSample.getIndex();
_timeStamp = aSample.getTimeStamp();
_sampligRate = aSample.getSamplingRate();
if ( aSample.getSampleData() != null ) {
_sampleData = Arrays.copyOf( aSample.getSampleData(), aSample.getChannelCount() );
}
}
/**
* Constructs a {@link MonoSample} instance with the according time and
* sample settings.
*
* @param aSample The sample (set of values representing the channels, one
* channel per value) for the related time positioning.
*/
public MonoSampleBuilderImpl( double aSample ) {
_sampleData[0] = aSample;
}
/**
* Constructs a {@link MonoSample} instance with the according time and
* sample settings.
*
* @param aTimeMillis The time positioning of this sound sample.
* @param aSample The sample (set of values representing the channels, one
* channel per value) for the related time positioning.
*/
public MonoSampleBuilderImpl( double aTimeMillis, double aSample ) {
_timeStamp = aTimeMillis;
_sampleData[0] = aSample;
}
/**
* Constructs a {@link MonoSample} instance with the according time and
* sample settings.
*
* @param aTimeMillis The time positioning of this sound sample.
* @param aSample The sample (set of values representing the channels, one
* channel per value) for the related time positioning.
* @param aSamplingRate The sampling rate for the given sample.
*/
public MonoSampleBuilderImpl( double aTimeMillis, double aSample, int aSamplingRate ) {
_timeStamp = aTimeMillis;
_sampleData[0] = aSample;
_sampligRate = aSamplingRate;
}
/**
* Constructs a {@link MonoSample} instance with the according time and
* sample settings.
*
* @param aSample The sample (set of values representing the channels, one
* channel per value) for the related time positioning.
* @param aSamplingRate The sampling rate for the given sample.
*/
public MonoSampleBuilderImpl( double aSample, int aSamplingRate ) {
_sampleData[0] = aSample;
_sampligRate = aSamplingRate;
}
/**
* Constructs a {@link MonoSample} instance with the according time and
* sample settings.
*
* @param aIndex The index of the sample according to its position in the
* sample sequence (e.g. sound file).
* @param aSample The sample (set of values representing the channels, one
* channel per value) for the related time positioning.
*/
public MonoSampleBuilderImpl( long aIndex, double aSample ) {
_index = aIndex;
_sampleData[0] = aSample;
}
/**
* Constructs a {@link MonoSample} instance with the according time and
* sample settings.
*
* @param aIndex The index of the sample according to its position in the
* sample sequence (e.g. sound file).
* @param aSample The sample (set of values representing the channels, one
* channel per value) for the related time positioning.
* @param aSamplingRate The sampling rate for the given sample.
*/
public MonoSampleBuilderImpl( long aIndex, double aSample, int aSamplingRate ) {
_index = aIndex;
_sampleData[0] = aSample;
_sampligRate = aSamplingRate;
}
/**
* Constructs a {@link MonoSample} instance with the according time and
* sample settings.
*
* @param aIndex The index of the sample according to its position in the
* sample sequence (e.g. sound file).
* @param aTimeMillis The time positioning of this sound sample.
* @param aSample The sample (set of values representing the channels, one
* channel per value) for the related time positioning.
*/
public MonoSampleBuilderImpl( long aIndex, double aTimeMillis, double aSample ) {
_index = aIndex;
_timeStamp = aTimeMillis;
_sampleData[0] = aSample;
}
/**
* Constructs a {@link MonoSample} instance with the according time and
* sample settings.
*
* @param aIndex The index of the sample according to its position in the
* sample sequence (e.g. sound file).
* @param aTimeMillis The time positioning of this sound sample.
* @param aSample The sample (set of values representing the channels, one
* channel per value) for the related time positioning.
* @param aSamplingRate The sampling rate for the given sample.
*/
public MonoSampleBuilderImpl( long aIndex, double aTimeMillis, double aSample, int aSamplingRate ) {
_index = aIndex;
_timeStamp = aTimeMillis;
_sampleData[0] = aSample;
_sampligRate = aSamplingRate;
}
// -------------------------------------------------------------------------
/**
* Constructs a {@link SoundSampleBuilder} instance with the according time
* and sample settings.
*
* @param aIndex The index of the sample according to its position in the
* sample sequence (e.g. sound file).
* @param aSamplingRate The sampling rate for the given sample.
*/
public MonoSampleBuilderImpl( long aIndex, int aSamplingRate ) {
_index = aIndex;
_sampligRate = aSamplingRate;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public void setMonoData( double aSampleData ) {
if ( _sampleData == null || _sampleData.length != 1 ) {
synchronized ( this ) {
if ( _sampleData == null || _sampleData.length != 1 ) {
_sampleData = new double[1];
}
}
}
_sampleData[0] = aSampleData;
}
/**
* {@inheritDoc}
*/
@Override
public double[] getSampleData() {
return _sampleData;
}
/**
* {@inheritDoc}
*/
@Override
public double getTimeStamp() {
return _timeStamp;
}
/**
* {@inheritDoc}
*/
@Override
public int getSamplingRate() {
return _sampligRate;
}
/**
* {@inheritDoc}
*/
@Override
public long getIndex() {
return _index;
}
/**
* {@inheritDoc}
*/
@Override
public void setIndex( long aIndex ) {
_index = aIndex;
}
/**
* {@inheritDoc}
*/
@Override
public void increaseIndex() {
_index++;
}
/**
* {@inheritDoc}
*/
@Override
public void decreaseIndex() {
_index--;
}
/**
* {@inheritDoc}
*/
@Override
public void setTimeStamp( double aTimeMillis ) {
_timeStamp = aTimeMillis;
}
/**
* {@inheritDoc}
*/
@Override
public void setSamplingRate( int aSamplingRate ) {
_sampligRate = aSamplingRate;
}
/**
* {@inheritDoc}
*/
@Override
public String toString() {
return "MonoSampleBuilderImpl [index=" + getIndex() + ", timeStamp=" + getTimeStamp() + ", sampleData=" + getMonoData() + ", sampligRate=" + getSamplingRate();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy