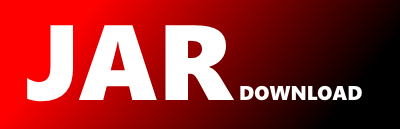
org.refcodes.audio.SamplingRate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-audio Show documentation
Show all versions of refcodes-audio Show documentation
Artifact providing audio provessing functionality such as generating
sine waves or writing raw audio samples to WAV files.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.audio;
/**
* The {@link SamplingRate} enumeration defines some common sampling rates. Use
* {@link #getLowestSamplingRate()} for the lowest sampling rate and
* {@link #getHigestSamplingRate()} for the highest sampling rate. On a
* {@link SamplingRate} instance, use {@link #getNextHigherSamplingRate()} for
* the next higher sampling rate and {@link #getPreviousLowerSamplingRate()} for
* the previous lower sampling rate. See
* "https://github.com/audiojs/sample-rate"
*/
public enum SamplingRate {
/**
* 8,000 Hz: Adequate for human speech but without sibilance. Used in
* telephone/walkie-talkie.
*/
TELEPHONE(8000),
/**
* 11,025 Hz Used for lower-quality PCM, MPEG audio and for audio analysis
* of subwoofer bandpasses.
*/
LOWEST_PCM(11025),
/**
* 16,000 Hz: Used in most VoIP and VVoIP, extension of telephone
* narrowband.
*/
VOICE_OVER_IP(16000),
/**
* 22,050 Hz: Used for lower-quality PCM and MPEG audio and for audio
* analysis of low frequency energy.
*/
LOW_PCM(22050),
/**
* 44,100 Hz: Audio CD, most commonly used rate with MPEG-1 audio (VCD,
* SVCD, MP3). Covers the 20 kHz bandwidth.
*/
AUDIO_CD(44100),
/**
* 48,000 Hz: Standard sampling rate used by professional digital video
* equipment, could reconstruct frequencies up to 22 kHz.
*/
PROFESSIONAL(48000),
/**
* 88,200 Hz: Used by some professional recording equipment when the
* destination is CD, such as mixers, EQs, compressors, reverb, crossovers
* and recording devices.
*/
HIGHER_PROFESSIONAL(88200),
/**
* 96,000 Hz: DVD-Audio, LPCM DVD tracks, Blu-ray audio tracks, HD DVD audio
* tracks.
*/
DVD_AUDIO(96000),
/**
* 176,400 Hz: Used in HDCD recorders and other professional applications
* for CD production.
*/
HDC(176400),
/**
* 192,000 Hz: Used with audio on professional video equipment. DVD-Audio,
* LPCM DVD tracks, Blu-ray audio tracks, HD DVD audio tracks.
*/
HIGH_PROFESSIONAL(192000),
/**
* 352,800 Hz Digital eXtreme Definition. Used for recording and editing
* Super Audio CDs.
*/
EXTREME(352800),
/**
* 384,000 Hz: Highest sample rate available for common software. Allows for
* precise peak detection.
*/
HIGHEST(384800);
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private int _samplingRate;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
private SamplingRate( int aSamplingRate ) {
_samplingRate = aSamplingRate;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* Returns the sampling rate in samples per second.
*
* @return The samples per second for this {@link SamplingRate}.
*/
public int getSamplesPerSecond() {
return _samplingRate;
}
/**
* Retrieves the lowest sampling rate.
*
* @return The lowest sampling rate.
*/
public static SamplingRate getLowestSamplingRate() {
return TELEPHONE;
}
/**
* Retrieves the highest sampling rate.
*
* @return The highest sampling rate.
*/
public static SamplingRate getHigestSamplingRate() {
return HIGHEST;
}
/**
* Retrieves the next higher sampling rate or null if it is already the
* highest sampling rate.
*
* @return The next higher sampling rate or null if already the highest
* sampling rate.
*/
public SamplingRate getNextHigherSamplingRate() {
return toNextHigherSamplingRate( this );
}
/**
* Retrieves the previous lower sampling rate or null if it is already the
* lowest sampling rate.
*
* @return The previous lower sampling rate or null if already the lowest
* sampling rate.
*/
public SamplingRate getPreviousLowerSamplingRate() {
return toPreviousLowerSamplingRate( this );
}
// /////////////////////////////////////////////////////////////////////////
// HELPER:
// /////////////////////////////////////////////////////////////////////////
/**
* Retrieves the next higher sampling rate or null if it is already the
* highest sampling rate.
*
* @param aSamplingRate The sampling rate for which to get the next higher
* one.
*
* @return The next higher sampling rate or null if already the highest
* sampling rate.
*/
private static SamplingRate toNextHigherSamplingRate( SamplingRate aSamplingRate ) {
for ( int i = 0; i < values().length - 1; i++ ) {
if ( aSamplingRate == values()[i] ) {
return values()[i + 1];
}
}
return null;
}
/**
* Retrieves the previous lower sampling rate or null if it is already the
* lowest sampling rate.
*
* @param aSamplingRate The sampling rate for which to get the previous
* lower one.
*
* @return The previous lower sampling rate or null if already the lowest
* sampling rate.
*/
private static SamplingRate toPreviousLowerSamplingRate( SamplingRate aSamplingRate ) {
for ( int i = 1; i < values().length; i++ ) {
if ( aSamplingRate == values()[i] ) {
return values()[i - 1];
}
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy