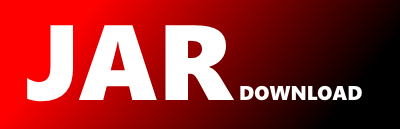
org.refcodes.audio.SoundSampleBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-audio Show documentation
Show all versions of refcodes-audio Show documentation
Artifact providing audio provessing functionality such as generating
sine waves or writing raw audio samples to WAV files.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.audio;
/**
* The {@link SoundSampleBuilder} extends the {@link SoundSample} with builder
* functionality.
*/
public interface SoundSampleBuilder extends SoundSample, SampleBuilder {
/**
* Sets the sample's data for the according time positioning. A sample is a
* value or set of values at a point in time. In case of mono audio, a
* sample represents a single value, in case of stereo audio, a sample
* represents a set of values, one value represents one channel (e.g. two
* channels when we have a left and a right speaker).
*
* @param aSampleData The sample's data for the according time positioning.
*/
void setSampleData( double[] aSampleData );
/**
* Sets the sample's data for the sample property.
*
* @param aSampleData The sample's data to be stored by the sample property.
*
* @return The builder for applying multiple build operations.
*/
default SoundSampleBuilder withSampleData( double[] aSampleData ) {
setSampleData( aSampleData );
return this;
}
/**
* Sets the position in time of this sound sample in ms.
*
* @param aTimeMillis The sound sample's time positioning in ms.
*/
@Override
void setTimeStamp( double aTimeMillis );
/**
* Sets the position in time of this sound sample in ms.
*
* @param aTimeMillis The sound sample's time positioning in ms.
*
* @return The builder for applying multiple build operations.
*/
@Override
default SoundSampleBuilder withTimeStamp( double aTimeMillis ) {
setTimeStamp( aTimeMillis );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default SoundSampleBuilder withIndex( long aIndex ) {
setIndex( aIndex );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default SoundSampleBuilder withIncreaseIndex() {
increaseIndex();
return this;
}
/**
* {@inheritDoc}
*/
@Override
default SoundSampleBuilder withDecreaseIndex() {
decreaseIndex();
return this;
}
/**
* {@inheritDoc}
*/
@Override
default SoundSampleBuilder withSamplingRate( int aSamplingRate ) {
setSamplingRate( aSamplingRate );
return this;
}
/**
* This is a convenience method for easily instantiating the according
* builder.
*
* @param aSample The sample (set of values representing the channels, one
* channel per value) for the related time positioning.
*
* @return The according {@link SoundSampleBuilder}.
*/
static SoundSampleBuilder build( double[] aSample ) {
return new SoundSampleBuilderImpl( aSample );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy