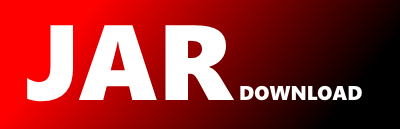
org.refcodes.audio.WavMonoSampleWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-audio Show documentation
Show all versions of refcodes-audio Show documentation
Artifact providing audio provessing functionality such as generating
sine waves or writing raw audio samples to WAV files.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.audio;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.OutputStream;
import org.refcodes.numerical.NumericalUtility;
/**
* The {@link WavMonoSampleWriter} provides means to write sound samples to a
* WAV file. Information on the WAV file format has been taken from the
* following article:
* "https://web.archive.org/web/20120113025807/http://technology.niagarac.on.ca:80/courses/ctec1631/WavFileFormat.html".
*/
public class WavMonoSampleWriter extends AbstractWavSampleWriter implements MonoSampleWriter {
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private final MonoSampleBuilder _soundSample = new MonoSampleBuilderImpl( 0, SamplingRate.AUDIO_CD.getSamplesPerSecond() );
private boolean _hasHeader = false;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Constructs the {@link WavMonoSampleWriter} for writing sound samples to a
* WAV file or stream.
*
* @param aFile The {@link File} where to write the CSV records to.
*
* @throws FileNotFoundException If the given file object does not denote an
* existing, writable regular file and a new regular file of that
* name cannot be created, or if some other error occurs while
* opening or creating the file.
*/
public WavMonoSampleWriter( File aFile ) throws FileNotFoundException {
super( aFile );
}
/**
* Constructs the {@link WavMonoSampleWriter} for writing sound samples to a
* WAV file or stream.
*
* @param aOutputStream The {@link OutputStream} where to write the CSV
* records to.
*/
public WavMonoSampleWriter( OutputStream aOutputStream ) {
super( aOutputStream );
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public WavMonoSampleWriter withBitsPerSample( BitsPerSample aBitsPerSample ) {
setBitsPerSample( aBitsPerSample );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public void writeNext( double aSampleData ) throws IOException {
_soundSample.setMonoData( aSampleData );
_soundSample.updateTimeStamp();
writeNext( _soundSample );
}
/**
* {@inheritDoc}
*/
@Override
public void writeNext( MonoSample aSample ) throws IOException {
// Header |-->
if ( !_hasHeader ) {
synchronized ( this ) {
if ( !_hasHeader ) {
if ( aSample.getSamplingRate() > 0 ) {
setSamplingRate( aSample.getSamplingRate() );
}
writeHeader( getSamplingRate(), 1 );
_hasHeader = true;
}
}
}
// Header <--|
final double theValue = toWavSample( aSample.getMonoData() );
final byte[] eBytes = NumericalUtility.toLittleEndianBytes( (long) theValue, getBitsPerSample().getByteCount() );
_outputStream.write( eBytes );
_soundSample.increaseIndex();
}
/**
* {@inheritDoc}
*/
@Override
public int getSamplingRate() {
return _soundSample.getSamplingRate();
}
/**
* {@inheritDoc}
*/
@Override
public void setSamplingRate( int aSamplingRate ) {
if ( aSamplingRate != -1 && aSamplingRate != _soundSample.getSamplingRate() ) {
_soundSample.setSamplingRate( aSamplingRate );
}
}
/**
* {@inheritDoc}
*/
@Override
public WavMonoSampleWriter withSamplingRate( int aSamplingRate ) {
setSamplingRate( aSamplingRate );
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy