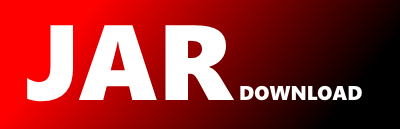
org.refcodes.checkerboard.AbstractCheckerboardViewer Maven / Gradle / Ivy
Show all versions of refcodes-checkerboard Show documentation
package org.refcodes.checkerboard;
import org.refcodes.component.InitializeException;
import org.refcodes.graphical.Dimension;
import org.refcodes.graphical.GridMode;
import org.refcodes.graphical.Offset;
import org.refcodes.graphical.Position;
import org.refcodes.graphical.ViewportDimension;
import org.refcodes.graphical.ViewportDimensionImpl.ViewportDimensionPropertyBuilderImpl;
import org.refcodes.graphical.ViewportOffset;
import org.refcodes.observer.SubscribeEvent;
import org.refcodes.observer.UnsubscribeEvent;
/**
* In order to provide a {@link Checkerboard}, register an observer by invoking
* {@link Checkerboard#subscribeObserver(Object)}. The default
* {@link CheckerboardImpl#subscribeObserver(org.refcodes.checkerboard.CheckerboardObserver)}
* method will invoke this {@link #onSubscribe(SubscribeEvent)} method which in
* turn sets the {@link Checkerboard} instance. Retrieve it by calling
* {@link #getCheckerboard()}
*
* @param the generic type
* @param The type which's instances represent a {@link Player} state.
* @param
The type for the state's representation ("image").
* @param the generic type
* @param The {@link CheckerboardViewer}'s type implementing this
* interface.
*/
public abstract class AbstractCheckerboardViewer, S, IMG, SF extends SpriteFactory
, CBV extends CheckerboardViewer
> implements CheckerboardViewer
{
// /////////////////////////////////////////////////////////////////////////
// STATICS:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// CONSTANTS:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private int _viewportWidth = -1;
private int _viewportHeight = -1;
private int _viewportOffsetX = 0;
private int _viewportOffsetY = 0;
private ViewportDimensionPropertyBuilderBuilder _minViewportDimension = new ViewportDimensionPropertyBuilderImpl( -1, -1 );
private Checkerboard
_checkerboard;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// INJECTION:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* With initialize.
*
* @return the cbv
* @throws InitializeException the initialize exception
*/
@SuppressWarnings("unchecked")
@Override
public CBV withInitialize() throws InitializeException {
initialize();
return (CBV) this;
}
/**
* With viewport offset Y.
*
* @param aPosY the pos Y
* @return the cbv
*/
@SuppressWarnings("unchecked")
@Override
public CBV withViewportOffsetY( int aPosY ) {
setViewportOffsetY( aPosY );
return (CBV) this;
}
/**
* With viewport height.
*
* @param aGridHeight the grid height
* @return the cbv
*/
@SuppressWarnings("unchecked")
@Override
public CBV withViewportHeight( int aGridHeight ) {
setViewportHeight( aGridHeight );
return (CBV) this;
}
/**
* With viewport width.
*
* @param aGridWidth the grid width
* @return the cbv
*/
@SuppressWarnings("unchecked")
@Override
public CBV withViewportWidth( int aGridWidth ) {
setViewportWidth( aGridWidth );
return (CBV) this;
}
/**
* With viewport dimension.
*
* @param aWidth the width
* @param aHeight the height
* @return the cbv
*/
@SuppressWarnings("unchecked")
@Override
public CBV withViewportDimension( int aWidth, int aHeight ) {
setViewportDimension( aWidth, aHeight );
return (CBV) this;
}
/**
* With viewport dimension.
*
* @param aGridDimension the grid dimension
* @return the cbv
*/
@SuppressWarnings("unchecked")
@Override
public CBV withViewportDimension( ViewportDimension aGridDimension ) {
setViewportDimension( aGridDimension );
return (CBV) this;
}
/**
* With viewport dimension.
*
* @param aDimension the dimension
* @return the cbv
*/
@SuppressWarnings("unchecked")
@Override
public CBV withViewportDimension( Dimension aDimension ) {
setViewportDimension( aDimension );
return (CBV) this;
}
/**
* With viewport offset.
*
* @param aPosX the pos X
* @param aPosY the pos Y
* @return the cbv
*/
@SuppressWarnings("unchecked")
@Override
public CBV withViewportOffset( int aPosX, int aPosY ) {
setViewportOffset( aPosX, aPosY );
return (CBV) this;
}
/**
* With viewport offset.
*
* @param aOffset the offset
* @return the cbv
*/
@SuppressWarnings("unchecked")
@Override
public CBV withViewportOffset( Position aOffset ) {
setViewportOffset( aOffset );
return (CBV) this;
}
/**
* With viewport offset.
*
* @param aOffset the offset
* @return the cbv
*/
@SuppressWarnings("unchecked")
@Override
public CBV withViewportOffset( ViewportOffset aOffset ) {
setViewportOffset( aOffset );
return (CBV) this;
}
/**
* With viewport offset.
*
* @param aOffset the offset
* @return the cbv
*/
@SuppressWarnings("unchecked")
@Override
public CBV withViewportOffset( Offset aOffset ) {
setViewportOffset( aOffset );
return (CBV) this;
}
/**
* With viewport offset X.
*
* @param aPosX the pos X
* @return the cbv
*/
@SuppressWarnings("unchecked")
@Override
public CBV withViewportOffsetX( int aPosX ) {
setViewportOffsetX( aPosX );
return (CBV) this;
}
/**
* With min viewport dimension.
*
* @param aDimension the dimension
* @return the cbv
*/
@SuppressWarnings("unchecked")
@Override
public CBV withMinViewportDimension( ViewportDimension aDimension ) {
setMinViewportDimension( aDimension );
return (CBV) this;
}
/**
* With min viewport dimension.
*
* @param aWidth the width
* @param aHeight the height
* @return the cbv
*/
@SuppressWarnings("unchecked")
@Override
public CBV withMinViewportDimension( int aWidth, int aHeight ) {
setMinViewportDimension( aWidth, aHeight );
return (CBV) this;
}
/**
* {@inheritDoc}
*/
@Override
public void setViewportDimension( int aWidth, int aHeight ) {
if ( _minViewportDimension.getViewportWidth() != -1 && aWidth < _minViewportDimension.getViewportWidth() ) {
throw new IllegalArgumentException( "The provided grid width <" + aWidth + "> is less than the min grid width (<" + _minViewportDimension.getViewportWidth() + ">)." );
}
if ( _minViewportDimension.getViewportHeight() != -1 && aHeight < _minViewportDimension.getViewportHeight() ) {
throw new IllegalArgumentException( "The provided grid height <" + aHeight + "> is less than the min grid height (<" + _minViewportDimension.getViewportHeight() + ">)." );
}
_viewportWidth = aWidth;
_viewportHeight = aHeight;
}
/**
* {@inheritDoc}
*/
@Override
public void setViewportDimension( ViewportDimension aGridDimension ) {
setViewportDimension( aGridDimension.getViewportWidth(), aGridDimension.getViewportHeight() );
}
/**
* {@inheritDoc}
*/
@Override
public void setViewportDimension( Dimension aDimension ) {
setViewportDimension( aDimension.getWidth(), aDimension.getHeight() );
}
/**
* {@inheritDoc}
*/
@Override
public void setViewportWidth( int aGridWidth ) {
setViewportDimension( aGridWidth, _viewportHeight );
}
/**
* {@inheritDoc}
*/
@Override
public void setViewportHeight( int aGridHeight ) {
setViewportDimension( _viewportWidth, aGridHeight );
}
/**
* {@inheritDoc}
*/
@Override
public int getViewportWidth() {
return _viewportWidth;
}
/**
* {@inheritDoc}
*/
@Override
public int getViewportHeight() {
return _viewportHeight;
}
/**
* {@inheritDoc}
*/
@Override
public void setViewportOffset( int aPosX, int aPosY ) {
if ( aPosX != _viewportOffsetX || aPosY != _viewportOffsetY ) {
ViewportOffsetChangedEvent
theEvent = new ViewportOffsetChangedEventImpl
( aPosX, aPosY, _viewportOffsetX, _viewportOffsetY, this );
_viewportOffsetX = aPosX;
_viewportOffsetY = aPosY;
onViewportOffsetChangedEvent( theEvent );
}
}
/**
* {@inheritDoc}
*/
@Override
public void setViewportOffset( Position aOffset ) {
setViewportOffset( aOffset.getPositionX(), aOffset.getPositionY() );
}
/**
* {@inheritDoc}
*/
@Override
public void setViewportOffset( ViewportOffset aOffset ) {
setViewportOffset( aOffset.getViewportOffsetX(), aOffset.getViewportOffsetY() );
}
/**
* {@inheritDoc}
*/
@Override
public void setViewportOffset( Offset aOffset ) {
setViewportOffset( aOffset.getOffsetX(), aOffset.getOffsetY() );
}
/**
* {@inheritDoc}
*/
@Override
public void setViewportOffsetX( int aPosX ) {
setViewportOffset( aPosX, _viewportOffsetY );
}
/**
* {@inheritDoc}
*/
@Override
public int getViewportOffsetX() {
return _viewportOffsetX;
}
/**
* {@inheritDoc}
*/
@Override
public int getViewportOffsetY() {
return _viewportOffsetY;
}
/**
* {@inheritDoc}
*/
@Override
public void setViewportOffsetY( int aPosY ) {
setViewportOffset( _viewportOffsetX, aPosY );
}
/**
* {@inheritDoc}
*/
@Override
public void setMinViewportDimension( ViewportDimension aDimension ) {
_minViewportDimension.setViewportDimension( aDimension );
}
/**
* {@inheritDoc}
*/
@Override
public ViewportDimension getMinViewportDimension() {
return _minViewportDimension;
}
/**
* {@inheritDoc}
*/
@Override
public void setMinViewportDimension( int aWidth, int aHeight ) {
_minViewportDimension.setViewportDimension( aWidth, aHeight );
}
/**
* {@inheritDoc}
*/
@Override
public GridMode getGridMode() {
return getCheckerboard().getGridMode();
}
/**
* {@inheritDoc}
*/
@Override
public int getGridWidth() {
return getCheckerboard().getGridWidth();
}
/**
* {@inheritDoc}
*/
@Override
public int getGridHeight() {
return getCheckerboard().getGridHeight();
}
// /////////////////////////////////////////////////////////////////////////
// LIFECYCLE:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public void destroy() {}
// /////////////////////////////////////////////////////////////////////////
// LIFECYCLE:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public void onSubscribe( SubscribeEvent> aSubscribeEvent ) {
_checkerboard = aSubscribeEvent.getSource();
}
/**
* {@inheritDoc}
*/
@Override
public void onUnsubscribe( UnsubscribeEvent> aUnsubscribeEvent ) {}
// /////////////////////////////////////////////////////////////////////////
// HOOKS:
// /////////////////////////////////////////////////////////////////////////
/**
* Gets the checkerboard.
*
* @return the checkerboard
*/
protected Checkerboard getCheckerboard() {
if ( _checkerboard == null ) {
throw new IllegalStateException( "Register your checkerboard viewer to a checkerboard via \"Checkerboard#subscribeObserver( yourCheckerboardViewer)\". The viewer's \"#onSubscribe( ... )} method will be invoked, retrieving (from the event) and setting the checkerboard object to be returnd by this \"getCheckerboard()\" method." );
}
return _checkerboard;
}
// /////////////////////////////////////////////////////////////////////////
// HELPER:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// INNER CLASSES:
// /////////////////////////////////////////////////////////////////////////
}