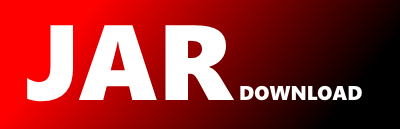
org.refcodes.checkerboard.AbstractGraphicalCheckerboardViewer Maven / Gradle / Ivy
Show all versions of refcodes-checkerboard Show documentation
package org.refcodes.checkerboard;
import org.refcodes.component.InitializeException;
import org.refcodes.graphical.Dimension;
import org.refcodes.graphical.FieldDimension;
import org.refcodes.graphical.GridMode;
import org.refcodes.graphical.MoveMode;
import org.refcodes.graphical.ScaleMode;
import org.refcodes.observer.UnsubscribeEvent;
/**
* The Class AbstractGraphicalCheckerboardViewer.
*
* @param The generic type representing a {@link Player}
* @param The type which's instances represent a {@link Player} state.
* @param
the generic type
* @param the generic type
* @param the generic type
* @param The {@link CheckerboardViewer}'s type implementing this
* interface.
*/
public abstract class AbstractGraphicalCheckerboardViewer, S, IMG, SF extends SpriteFactory
, BF extends BackgroundFactory
, CBV extends GraphicalCheckerboardViewer
> extends AbstractCheckerboardViewer
implements GraphicalCheckerboardViewer
{
// /////////////////////////////////////////////////////////////////////////
// STATICS:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// CONSTANTS:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private int _fieldHeight = -1;
private int _fieldWidth = -1;
private int _fieldGap = 0;
private MoveMode _moveMode = MoveMode.SMOOTH;
private ScaleMode _scaleMode = ScaleMode.SCALE_GRID;
private SF _spriteFactory = null;
private BF _backgroundFactory = null;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// INJECTION:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public int getFieldGap() {
return _fieldGap;
}
/**
* {@inheritDoc}
*/
@Override
public void setFieldGap( int aFieldGap ) {
_fieldGap = aFieldGap;
}
/**
* With field gap.
*
* @param aFieldGap the field gap
* @return the cbv
*/
@SuppressWarnings("unchecked")
@Override
public CBV withFieldGap( int aFieldGap ) {
setFieldGap( aFieldGap );
return (CBV) this;
}
/**
* {@inheritDoc}
*/
@Override
public void show() {
setVisible( true );
}
/**
* {@inheritDoc}
*/
@Override
public void hide() {
setVisible( false );
}
/**
* {@inheritDoc}
*/
@Override
public MoveMode getMoveMode() {
return _moveMode;
}
/**
* {@inheritDoc}
*/
@Override
public void setMoveMode( MoveMode aMode ) {
_moveMode = aMode;
}
/**
* {@inheritDoc}
*/
@Override
public ScaleMode getScaleMode() {
return _scaleMode;
}
/**
* {@inheritDoc}
*/
@Override
public void setScaleMode( ScaleMode aMode ) {
_scaleMode = aMode;
}
/**
* {@inheritDoc}
*/
@Override
public int getFieldHeight() {
return _fieldHeight;
}
/**
* {@inheritDoc}
*/
@Override
public void setFieldDimension( int aFieldWidth, int aFieldHeight ) {
_fieldWidth = aFieldWidth;
_fieldHeight = aFieldHeight;
}
/**
* {@inheritDoc}
*/
@Override
public void setFieldDimension( FieldDimension aField ) {
setFieldDimension( aField.getFieldWidth(), aField.getFieldHeight() );
}
/**
* {@inheritDoc}
*/
@Override
public void setFieldDimension( Dimension aDimension ) {
setFieldDimension( aDimension.getWidth(), aDimension.getHeight() );
}
/**
* {@inheritDoc}
*/
@Override
public void setFieldWidth( int aWidth ) {
setFieldDimension( aWidth, _fieldHeight );
}
/**
* {@inheritDoc}
*/
@Override
public int getFieldWidth() {
return _fieldWidth;
}
/**
* {@inheritDoc}
*/
@Override
public void setFieldHeight( int aHeight ) {
setFieldDimension( _fieldWidth, aHeight );
}
/**
* {@inheritDoc}
*/
@Override
public int getContainerHeight() {
return getFieldHeight() * getViewportHeight() + (getFieldGap() * (getViewportHeight() - 1)) + ((getCheckerboard().getGridMode() == GridMode.CLOSED) ? (getFieldGap() * 2) : 0);
}
/**
* {@inheritDoc}
*/
@Override
public int getContainerWidth() {
return getFieldWidth() * getViewportWidth() + (getFieldGap() * (getViewportWidth() - 1)) + ((getCheckerboard().getGridMode() == GridMode.CLOSED) ? (getFieldGap() * 2) : 0);
}
/**
* With field width.
*
* @param aWidth the width
* @return the cbv
*/
@SuppressWarnings("unchecked")
@Override
public CBV withFieldWidth( int aWidth ) {
setFieldWidth( aWidth );
return (CBV) this;
}
/**
* With field height.
*
* @param aHeight the height
* @return the cbv
*/
@SuppressWarnings("unchecked")
@Override
public CBV withFieldHeight( int aHeight ) {
setFieldHeight( aHeight );
return (CBV) this;
}
/**
* With field dimension.
*
* @param aField the field
* @return the cbv
*/
@SuppressWarnings("unchecked")
@Override
public CBV withFieldDimension( FieldDimension aField ) {
setFieldDimension( aField );
return (CBV) this;
}
/**
* With field dimension.
*
* @param aDimension the dimension
* @return the cbv
*/
@SuppressWarnings("unchecked")
@Override
public CBV withFieldDimension( Dimension aDimension ) {
setFieldDimension( aDimension );
return (CBV) this;
}
/**
* With initialize.
*
* @return the cbv
* @throws InitializeException the initialize exception
*/
@SuppressWarnings("unchecked")
@Override
public CBV withInitialize() throws InitializeException {
initialize();
return (CBV) this;
}
/**
* With show.
*
* @return the cbv
*/
@SuppressWarnings("unchecked")
@Override
public CBV withShow() {
setVisible( true );
return (CBV) this;
}
/**
* With hide.
*
* @return the cbv
*/
@SuppressWarnings("unchecked")
@Override
public CBV withHide() {
setVisible( false );
return (CBV) this;
}
/**
* With visible.
*
* @param isVisible the is visible
* @return the cbv
*/
@SuppressWarnings("unchecked")
@Override
public CBV withVisible( boolean isVisible ) {
setVisible( isVisible );
return (CBV) this;
}
/**
* With move mode.
*
* @param aMode the mode
* @return the cbv
*/
@SuppressWarnings("unchecked")
@Override
public CBV withMoveMode( MoveMode aMode ) {
setMoveMode( aMode );
return (CBV) this;
}
/**
* With scale mode.
*
* @param aMode the mode
* @return the cbv
*/
@SuppressWarnings("unchecked")
@Override
public CBV withScaleMode( ScaleMode aMode ) {
setScaleMode( aMode );
return (CBV) this;
}
/**
* {@inheritDoc}
*/
@Override
public int toTotalWidth() {
int theTotalWidth = getFieldWidth() * getViewportWidth() + (getFieldGap() * (getViewportWidth() - 1));
if ( getGridMode() == GridMode.CLOSED ) {
theTotalWidth += (getFieldGap() * 2);
}
return theTotalWidth;
}
/**
* {@inheritDoc}
*/
@Override
public void setFieldDimension( int aFieldWidth, int aFieldHeight, int aGap ) {
setFieldDimension( aFieldWidth, aFieldHeight );
setFieldGap( aGap );
}
/**
* With field dimension.
*
* @param aFieldWidth the field width
* @param aFieldHeight the field height
* @param aGap the gap
* @return the cbv
*/
@SuppressWarnings("unchecked")
@Override
public CBV withFieldDimension( int aFieldWidth, int aFieldHeight, int aGap ) {
setFieldDimension( aFieldWidth, aFieldHeight, aGap );
return (CBV) this;
}
/**
* {@inheritDoc}
*/
@Override
public int toTotalHeight() {
int theTotalHeight = getFieldHeight() * getViewportHeight() + (getFieldGap() * (getViewportHeight() - 1));
if ( getGridMode() == GridMode.CLOSED ) {
theTotalHeight += (getFieldGap() * 2);
}
return theTotalHeight;
}
/**
* With field dimension.
*
* @param aFieldWidth the field width
* @param aFieldHeight the field height
* @return the cbv
*/
@SuppressWarnings("unchecked")
@Override
public CBV withFieldDimension( int aFieldWidth, int aFieldHeight ) {
setFieldDimension( aFieldWidth, aFieldHeight );
return (CBV) this;
}
/**
* {@inheritDoc}
*/
@Override
public void setSpriteFactory( SF aSpriteFactory ) {
_spriteFactory = aSpriteFactory;
}
/**
* {@inheritDoc}
*/
@Override
public SF getSpriteFactory() {
return _spriteFactory;
}
/**
* With sprite factory.
*
* @param aSpriteFactory the sprite factory
* @return the cbv
*/
@SuppressWarnings("unchecked")
@Override
public CBV withSpriteFactory( SF aSpriteFactory ) {
setSpriteFactory( aSpriteFactory );
return (CBV) this;
}
/**
* With background factory.
*
* @param aBackgroundFactory the background factory
* @return the cbv
*/
@SuppressWarnings("unchecked")
@Override
public CBV withBackgroundFactory( BF aBackgroundFactory ) {
setBackgroundFactory( aBackgroundFactory );
return (CBV) this;
}
/**
* {@inheritDoc}
*/
@Override
public void setBackgroundFactory( BF aBackgroundFactory ) {
_backgroundFactory = aBackgroundFactory;
}
/**
* {@inheritDoc}
*/
@Override
public BF getBackgroundFactory() {
return _backgroundFactory;
}
// /////////////////////////////////////////////////////////////////////////
// LIFECYCLE:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public void onUnsubscribe( UnsubscribeEvent> aUnsubscribeEvent ) {}
// /////////////////////////////////////////////////////////////////////////
// HOOKS:
// /////////////////////////////////////////////////////////////////////////
/**
* Gets the checkerboard.
*
* @return the checkerboard
*/
@Override
protected Checkerboard getCheckerboard() {
return super.getCheckerboard();
}
// /////////////////////////////////////////////////////////////////////////
// HELPER:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// INNER CLASSES:
// /////////////////////////////////////////////////////////////////////////
}