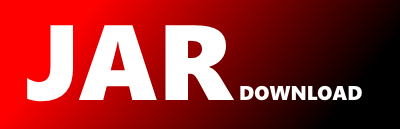
org.refcodes.checkerboard.PlayerObserver Maven / Gradle / Ivy
Show all versions of refcodes-checkerboard Show documentation
package org.refcodes.checkerboard;
import org.refcodes.exception.VetoException;
/**
* An asynchronous update interface for receiving notifications about Player
* information as the Player is constructed.
*
* @param the generic type
* @param the generic type
*/
public interface PlayerObserver
, S> {
/**
* This method is called when information about an Player which was
* previously requested using an asynchronous interface becomes available.
*
* @param aPlayerEvent the player event
*/
void onPlayerEvent( PlayerEvent
aPlayerEvent );
/**
* This method is called when information about an Player which was
* previously requested using an asynchronous interface becomes available.
*
* @param aPlayerEvent the player event
* @throws VetoException the veto exception
*/
void onChangePositionEvent( ChangePositionEvent
aPlayerEvent ) throws VetoException;
/**
* This method is called when information about an Player which was
* previously requested using an asynchronous interface becomes available.
*
* @param aPlayerEvent the player event
*/
void onPositionChangedEvent( PositionChangedEvent
aPlayerEvent );
/**
* This method is called when information about an Player which was
* previously requested using an asynchronous interface becomes available.
*
* @param aPlayerEvent the player event
*/
void onStateChangedEvent( StateChangedEvent
aPlayerEvent );
/**
* This method is called when information about an Player which was
* previously requested using an asynchronous interface becomes available.
*
* @param aPlayerEvent the player event
*/
void onVisibilityChangedEvent( VisibilityChangedEvent
aPlayerEvent );
/**
* This method is called when information about an Player which was
* previously requested using an asynchronous interface becomes available.
*
* @param aPlayerEvent the player event
*/
void onDraggabilityChangedEvent( DraggabilityChangedEvent
aPlayerEvent );
}