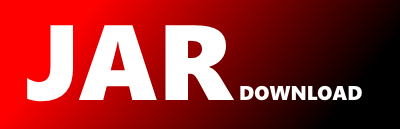
org.refcodes.checkerboard.AbstractCheckerboard Maven / Gradle / Ivy
Show all versions of refcodes-checkerboard Show documentation
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.checkerboard;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.function.Consumer;
import org.refcodes.controlflow.ExecutionStrategy;
import org.refcodes.exception.VetoException;
import org.refcodes.graphical.Dimension;
import org.refcodes.graphical.GridDimension;
import org.refcodes.graphical.GridMode;
import org.refcodes.graphical.Position;
import org.refcodes.graphical.PositionImpl;
import org.refcodes.observer.AbstractObservable;
import org.refcodes.observer.GenericEvent;
import org.refcodes.observer.SubscribeEvent;
import org.refcodes.observer.SubscribeEventImpl;
import org.refcodes.observer.UnsubscribeEvent;
import org.refcodes.observer.UnsubscribeEventImpl;
/**
* The Class CheckerboardImpl.
*
* @param the generic type
* @param the generic type
*/
public abstract class AbstractCheckerboard, P extends Player, S> extends AbstractObservable, GenericEvent>> implements Checkerboard {
// /////////////////////////////////////////////////////////////////////////
// STATICS:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// CONSTANTS:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private List
_players = new ArrayList<>();
private Map> _rowToColumn = new HashMap<>();
private Map> _columnToRow = new HashMap<>();
private int _gridWidth = -1;
private int _gridHeight = -1;
private GridMode _gridMode = GridMode.NONE;
private CheckerboardPlayerObserverImpl _observer = new CheckerboardPlayerObserverImpl();
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// BUILDER:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@SuppressWarnings("unchecked")
@Override
public T withGridMode( GridMode aGridMode ) {
setGridMode( aGridMode );
return (T) this;
}
/**
* {@inheritDoc}
*/
@SuppressWarnings("unchecked")
@Override
public T withGridDimension( int aGridWidth, int aGridHeight ) {
setGridDimension( aGridWidth, aGridHeight );
return (T) this;
}
/**
* {@inheritDoc}
*/
@SuppressWarnings("unchecked")
@Override
public T withGridDimension( GridDimension aDimension ) {
setGridDimension( aDimension );
return (T) this;
}
/**
* {@inheritDoc}
*/
@SuppressWarnings("unchecked")
@Override
public T withGridDimension( Dimension aDimension ) {
setGridDimension( aDimension );
return (T) this;
}
/**
* {@inheritDoc}
*/
@SuppressWarnings("unchecked")
@Override
public T withGridWidth( int aWidth ) {
setGridWidth( aWidth );
return (T) this;
}
/**
* {@inheritDoc}
*/
@SuppressWarnings("unchecked")
@Override
public T withGridHeight( int aHeight ) {
setGridHeight( aHeight );
return (T) this;
}
// /////////////////////////////////////////////////////////////////////////
// INJECTION:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public void forEach( Consumer aConsumer ) {
new ArrayList<>( _players ).forEach( aConsumer );
}
/**
* {@inheritDoc}
*/
@Override
public boolean hasAtPosition( Position aPos ) throws IndexOutOfBoundsException {
return hasAtPosition( aPos.getPositionX(), aPos.getPositionY() );
}
/**
* {@inheritDoc}
*/
@Override
public boolean hasAtPosition( int aPosX, int aPosY ) throws IndexOutOfBoundsException {
Position thePos = toAbsPos( aPosX, aPosY );
Map theColumn = _rowToColumn.get( thePos.getPositionX() );
if ( theColumn != null ) {
return theColumn.containsKey( thePos.getPositionY() );
}
return false;
}
/**
* {@inheritDoc}
*/
@Override
public P atPosition( Position aPos ) throws IndexOutOfBoundsException {
return atPosition( aPos.getPositionX(), aPos.getPositionY() );
}
/**
* {@inheritDoc}
*/
@Override
public P atPosition( int aPosX, int aPosY ) throws IndexOutOfBoundsException {
Position thePos = toAbsPos( aPosX, aPosY );
Map theColumn = _rowToColumn.get( thePos.getPositionX() );
if ( theColumn != null ) {
return theColumn.get( thePos.getPositionY() );
}
return null;
}
/**
* {@inheritDoc}
*/
@Override
public boolean hasAtTopOf( Position aPos ) throws IndexOutOfBoundsException {
int x = aPos.getPositionX();
int y = toTopYPosition( aPos );
return hasAtPosition( x, y );
}
/**
* {@inheritDoc}
*/
@Override
public P atTopOf( Position aPos ) throws IndexOutOfBoundsException {
int x = aPos.getPositionX();
int y = toTopYPosition( aPos );
return atPosition( x, y );
}
/**
* {@inheritDoc}
*/
@Override
public boolean hasAtTopRightOf( Position aPos ) throws IndexOutOfBoundsException {
int y = toTopYPosition( aPos );
int x = toRightXPosition( aPos );
return hasAtPosition( x, y );
}
/**
* {@inheritDoc}
*/
@Override
public P atTopRightOf( Position aPos ) throws IndexOutOfBoundsException {
int y = toTopYPosition( aPos );
int x = toRightXPosition( aPos );
return atPosition( x, y );
}
/**
* {@inheritDoc}
*/
@Override
public boolean hasAtRightOf( Position aPos ) throws IndexOutOfBoundsException {
int y = aPos.getPositionY();
int x = toRightXPosition( aPos );
return hasAtPosition( x, y );
}
/**
* {@inheritDoc}
*/
@Override
public P atRightOf( Position aPos ) throws IndexOutOfBoundsException {
int y = aPos.getPositionY();
int x = toRightXPosition( aPos );
return atPosition( x, y );
}
/**
* {@inheritDoc}
*/
@Override
public boolean hasAtBottomRightOf( Position aPos ) throws IndexOutOfBoundsException {
int y = toBottomYPosition( aPos );
int x = toRightXPosition( aPos );
return hasAtPosition( x, y );
}
/**
* {@inheritDoc}
*/
@Override
public P atBottomRightOf( Position aPos ) throws IndexOutOfBoundsException {
int y = toBottomYPosition( aPos );
int x = toRightXPosition( aPos );
return atPosition( x, y );
}
/**
* {@inheritDoc}
*/
@Override
public boolean hasAtBottomOf( Position aPos ) throws IndexOutOfBoundsException {
int x = aPos.getPositionX();
int y = toBottomYPosition( aPos );
return hasAtPosition( x, y );
}
/**
* {@inheritDoc}
*/
@Override
public P atBottomOf( Position aPos ) throws IndexOutOfBoundsException {
int x = aPos.getPositionX();
int y = toBottomYPosition( aPos );
return atPosition( x, y );
}
/**
* {@inheritDoc}
*/
@Override
public boolean hasAtBottomLeftOf( Position aPos ) throws IndexOutOfBoundsException {
int y = toBottomYPosition( aPos );
int x = toLeftXPosition( aPos );
return hasAtPosition( x, y );
}
/**
* {@inheritDoc}
*/
@Override
public P atBottomLeftOf( Position aPos ) throws IndexOutOfBoundsException {
int y = toBottomYPosition( aPos );
int x = toLeftXPosition( aPos );
return atPosition( x, y );
}
/**
* {@inheritDoc}
*/
@Override
public boolean hasAtLeftOf( Position aPos ) throws IndexOutOfBoundsException {
int y = aPos.getPositionY();
int x = toLeftXPosition( aPos );
return hasAtPosition( x, y );
}
/**
* {@inheritDoc}
*/
@Override
public P atLeftOf( Position aPos ) throws IndexOutOfBoundsException {
int y = aPos.getPositionY();
int x = toLeftXPosition( aPos );
return atPosition( x, y );
}
/**
* {@inheritDoc}
*/
@Override
public boolean hasAtTopLeftOf( Position aPos ) throws IndexOutOfBoundsException {
int y = toTopYPosition( aPos );
int x = toLeftXPosition( aPos );
return hasAtPosition( x, y );
}
/**
* {@inheritDoc}
*/
@Override
public P atTopLeftOf( Position aPos ) throws IndexOutOfBoundsException {
int y = toTopYPosition( aPos );
int x = toLeftXPosition( aPos );
return atPosition( x, y );
}
/**
* {@inheritDoc}
*/
@Override
public Map getRow( int aRow ) throws IndexOutOfBoundsException {
return _rowToColumn.get( aRow );
}
/**
* {@inheritDoc}
*/
@Override
public Map getColumn( int aColumn ) throws IndexOutOfBoundsException {
return _columnToRow.get( aColumn );
}
/**
* {@inheritDoc}
*/
@Override
public GridMode getGridMode() {
return _gridMode;
}
/**
* {@inheritDoc}
*/
@Override
public void setGridMode( GridMode aGridMode ) {
if ( aGridMode != _gridMode ) {
GridModeChangedEvent theEvent = new GridModeChangedEvent<>( aGridMode, _gridMode, this );
_gridMode = aGridMode;
try {
fireEvent( theEvent, ExecutionStrategy.SEQUENTIAL );
}
catch ( VetoException ignore ) {}
}
}
/**
* {@inheritDoc}
*/
@Override
public List
getPlayers() {
return _players;
}
/**
* {@inheritDoc}
*/
@Override
public P putPlayer( P aPlayer ) {
_players.add( aPlayer );
P thePrevPlayer = atPosition( aPlayer );
if ( thePrevPlayer != null ) {
if ( !removePlayer( thePrevPlayer ) ) {
throw new IllegalStateException( "Illegal state detected while replacing player \"" + thePrevPlayer + "> from position (" + thePrevPlayer.getPositionX() + ":" + aPlayer.getPositionY() + ") with player player \"" + aPlayer + "> from position (" + aPlayer.getPositionX() + ":" + aPlayer.getPositionY() + "). Probably the player's coordinates changed while removing." );
}
}
if ( addToLookup( aPlayer ) != null ) {
throw new IllegalStateException( "Illegal state detected while removing player \"" + aPlayer + "> from position (" + aPlayer.getPositionX() + ":" + aPlayer.getPositionY() + "). Probably the player's coordinates changed while removing." );
}
PlayerAddedEvent
theEvent = new PlayerAddedEvent<>( aPlayer, this );
aPlayer.subscribeObserver( _observer );
try {
fireEvent( theEvent, ExecutionStrategy.SEQUENTIAL );
}
catch ( VetoException ignore ) {}
return thePrevPlayer;
}
/**
* {@inheritDoc}
*/
@Override
public boolean removePlayer( P aPlayer ) {
if ( _players.remove( aPlayer ) ) {
if ( removeFromLookup( aPlayer ) != aPlayer ) {
throw new IllegalStateException( "Illegal state detected while removing player \"" + aPlayer + "> from position (" + aPlayer.getPositionX() + ":" + aPlayer.getPositionY() + "). Probably the player's coordinates changed while removing." );
}
PlayerRemovedEvent
theEvent = new PlayerRemovedEvent<>( aPlayer, this );
aPlayer.unsubscribeObserver( _observer );
aPlayer.subscribeObserver( _observer );
try {
fireEvent( theEvent, ExecutionStrategy.SEQUENTIAL );
}
catch ( VetoException ignore ) {}
return true;
}
return false;
}
/**
* {@inheritDoc}
*/
@Override
public void clearPlayers() {
_players.clear();
}
/**
* {@inheritDoc}
*/
@Override
public int playerCount() {
return _players.size();
}
/**
* {@inheritDoc}
*/
@Override
public boolean hasPlayers() {
return !_players.isEmpty();
}
/**
* {@inheritDoc}
*/
@Override
public Iterator
players() {
return _players.iterator();
}
/**
* {@inheritDoc}
*/
@Override
public boolean hasPlayer( P aPlayer ) {
return _players.contains( aPlayer );
}
/**
* {@inheritDoc}
*/
@Override
public int getGridWidth() {
return _gridWidth;
}
/**
* {@inheritDoc}
*/
@Override
public void setGridDimension( int aWidth, int aHeight ) {
if ( aWidth != _gridWidth || aHeight != _gridHeight ) {
GridDimensionChangedEvent
theEvent = new GridDimensionChangedEvent<>( aWidth, aHeight, _gridWidth, _gridHeight, this );
_gridWidth = aWidth;
_gridHeight = aHeight;
try {
fireEvent( theEvent, ExecutionStrategy.SEQUENTIAL );
}
catch ( VetoException ignore ) {}
}
}
/**
* {@inheritDoc}
*/
@Override
public void setGridDimension( GridDimension aDimension ) {
setGridDimension( aDimension.getGridWidth(), aDimension.getGridHeight() );
}
/**
* {@inheritDoc}
*/
@Override
public void setGridDimension( Dimension aDimension ) {
setGridDimension( aDimension.getWidth(), aDimension.getHeight() );
}
/**
* {@inheritDoc}
*/
@Override
public void setGridWidth( int aWidth ) {
setGridDimension( aWidth, _gridHeight );
}
/**
* {@inheritDoc}
*/
@Override
public void setGridHeight( int aHeight ) {
setGridDimension( _gridWidth, aHeight );
}
/**
* {@inheritDoc}
*/
@Override
public int getGridHeight() {
return _gridHeight;
}
// /////////////////////////////////////////////////////////////////////////
// LIFECYCLE:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public boolean subscribeObserver( CheckerboardObserver
aObserver ) {
if ( super.subscribeObserver( aObserver ) ) {
try {
fireEvent( new SubscribeEventImpl>( this ), ExecutionStrategy.SEQUENTIAL );
}
catch ( VetoException ignore ) {}
return true;
}
return false;
}
/**
* {@inheritDoc}
*/
@Override
public boolean unsubscribeObserver( CheckerboardObserver aObserver ) {
try {
fireEvent( new UnsubscribeEventImpl>( this ), ExecutionStrategy.SEQUENTIAL );
}
catch ( VetoException ignore ) {}
return super.unsubscribeObserver( aObserver );
}
/**
* {@inheritDoc}
*/
@Override
public void destroy() {}
/**
* {@inheritDoc}
*/
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((_players == null) ? 0 : _players.hashCode());
return result;
}
/**
* {@inheritDoc}
*/
@Override
public boolean equals( Object obj ) {
if ( this == obj ) return true;
if ( obj == null ) return false;
if ( getClass() != obj.getClass() ) return false;
AbstractCheckerboard, ?, ?> other = (AbstractCheckerboard, ?, ?>) obj;
if ( _players == null ) {
if ( other._players != null ) return false;
}
else if ( !_players.equals( other._players ) ) return false;
return true;
}
// /////////////////////////////////////////////////////////////////////////
// HOOKS:
// /////////////////////////////////////////////////////////////////////////
/**
* Fire event.
*
* @param aEvent the event
* @param aObserver the observer
* @param aExecutionStrategy the execution strategy
*
* @return true, if successful
*
* @throws VetoException the veto exception
*/
@SuppressWarnings("unchecked")
@Override
protected boolean fireEvent( GenericEvent> aEvent, CheckerboardObserver aObserver, ExecutionStrategy aExecutionStrategy ) throws VetoException {
if ( aEvent instanceof SubscribeEvent ) {
aObserver.onSubscribe( (SubscribeEvent>) aEvent );
}
if ( aEvent instanceof UnsubscribeEvent ) {
aObserver.onUnsubscribe( (UnsubscribeEvent>) aEvent );
}
if ( aEvent instanceof CheckerboardEvent, ?> ) {
aObserver.onCheckerboardEvent( (CheckerboardEvent) aEvent );
if ( aEvent instanceof PlayerAddedEvent ) {
aObserver.onPlayerAddedEvent( (PlayerAddedEvent
) aEvent );
}
if ( aEvent instanceof PlayerRemovedEvent ) {
aObserver.onPlayerRemovedEvent( (PlayerRemovedEvent
) aEvent );
}
if ( aEvent instanceof GridModeChangedEvent ) {
aObserver.onGridModeChangedEvent( (GridModeChangedEvent
) aEvent );
}
if ( aEvent instanceof ViewportDimensionChangedEvent ) {
aObserver.onViewportDimensionChangedEvent( (ViewportDimensionChangedEvent
) aEvent );
}
if ( aEvent instanceof ViewportOffsetChangedEvent ) {
aObserver.onViewportOffsetChangedEvent( (ViewportOffsetChangedEvent
) aEvent );
}
}
else if ( aEvent instanceof PlayerEvent> ) {
aObserver.onPlayerEvent( (PlayerEvent
) aEvent, this );
if ( aEvent instanceof ChangePositionEvent ) {
aObserver.onChangePositionEvent( (ChangePositionEvent
) aEvent, this );
}
if ( aEvent instanceof PositionChangedEvent ) {
aObserver.onPositionChangedEvent( (PositionChangedEvent
) aEvent, this );
}
if ( aEvent instanceof StateChangedEvent ) {
aObserver.onStateChangedEvent( (StateChangedEvent
) aEvent, this );
}
if ( aEvent instanceof VisibilityChangedEvent ) {
aObserver.onVisibilityChangedEvent( (VisibilityChangedEvent
) aEvent, this );
}
if ( aEvent instanceof DraggabilityChangedEvent ) {
aObserver.onDraggabilityChangedEvent( (DraggabilityChangedEvent
) aEvent, this );
}
}
return true;
}
// /////////////////////////////////////////////////////////////////////////
// HELPER:
// /////////////////////////////////////////////////////////////////////////
/**
* Calculates the absolute position as of the {@link GridMode}. In case the
* {@link GridMode} is set to {@link GridMode#PERIODIC} and the positions
* exceed the grid dimension, then the positions are reckoned to be a
* multiple of the grid dimensions and relocated accordingly.
*
* @param aPosX The x-position.
* @param aPosX The y-position.
*
* @return The position as of the {@link GridMode}.
*/
private Position toAbsPos( int aPosX, int aPosY ) {
if ( getGridMode() == GridMode.PERIODIC ) {
while ( aPosX < 0 ) { // Modulo?
aPosX = getGridWidth() + aPosX;
}
while ( aPosY < 0 ) { // Modulo?
aPosY = getGridHeight() + aPosY;
}
while ( aPosX >= getGridWidth() ) { // Modulo?
aPosX = aPosX - getGridWidth();
}
while ( aPosY >= getGridHeight() ) { // Modulo?
aPosY = aPosY - getGridHeight();
}
}
Position thePos = new PositionImpl( aPosX, aPosY );
return thePos;
}
/**
* Removes the from lookup.
*
* @param aPosition the position
*
* @return the p
*/
private synchronized P removeFromLookup( Position aPosition ) {
P removeFromRow = null;
Map theColumn = _rowToColumn.get( aPosition.getPositionX() );
if ( theColumn != null ) {
removeFromRow = theColumn.remove( aPosition.getPositionY() );
}
P removeFromColumn = null;
Map theRow = _columnToRow.get( aPosition.getPositionY() );
if ( theRow != null ) {
removeFromColumn = theRow.remove( aPosition.getPositionX() );
}
if ( removeFromColumn != removeFromRow ) {
throw new IllegalStateException( "Illegal state detected while removing player \"" + aPosition + "> from position (" + aPosition.getPositionX() + ":" + aPosition.getPositionY() + "). Probably the player's coordinates changed while removing." );
}
return removeFromColumn;
}
/**
* Adds the to lookup.
*
* @param aPlayer the player
*
* @return the p
*/
private synchronized P addToLookup( P aPlayer ) {
P theAddFromRow = null;
Map theColumn = _rowToColumn.get( aPlayer.getPositionX() );
if ( theColumn == null ) {
theColumn = new HashMap<>();
_rowToColumn.put( aPlayer.getPositionX(), theColumn );
}
theAddFromRow = theColumn.put( aPlayer.getPositionY(), aPlayer );
P theAddFromColumn = null;
Map theRow = _columnToRow.get( aPlayer.getPositionY() );
if ( theRow == null ) {
theRow = new HashMap<>();
_columnToRow.put( aPlayer.getPositionY(), theRow );
}
theAddFromColumn = theRow.put( aPlayer.getPositionX(), aPlayer );
if ( theAddFromColumn != theAddFromRow ) {
throw new IllegalStateException( "Illegal state detected while removing player \"" + aPlayer + "> from position (" + aPlayer.getPositionX() + ":" + aPlayer.getPositionY() + "). Probably the player's coordinates changed while removing." );
}
return theAddFromColumn;
}
/**
* Update player.
*
* @param aPlayer the the player
* @param aPrecedingPosition the the preceding position
*
* @return the p
*/
private P updatePlayer( P aPlayer, Position aPrecedingPosition ) {
P theReplacedPlayer = atPosition( aPlayer );
removePlayer( theReplacedPlayer );
P theTmpPlayer = addToLookup( aPlayer );
if ( theTmpPlayer != null ) {
throw new IllegalStateException( "Illegal state detected while moving player \"" + aPlayer + "> from position (" + aPrecedingPosition.toString() + "): The target position (although being cleared before) now contains another player \"" + theTmpPlayer + ">. Probably some thread race condition and insufficient synchronization." );
}
P thePrevPlayerPos = removeFromLookup( aPrecedingPosition );
if ( thePrevPlayerPos != aPlayer ) {
throw new IllegalStateException( "Illegal state detected while moving player \"" + aPlayer + "> from position (" + aPrecedingPosition.toString() + "): The previous position contains the wrong player \"" + thePrevPlayerPos + "> instead of the expected player \"" + aPlayer + "\". Probably some thread race condition and insufficient synchronization." );
}
return theReplacedPlayer;
}
/**
* To top Y position.
*
* @param aPos the pos
*
* @return the int
*/
private int toTopYPosition( Position aPos ) {
int y = aPos.getPositionY() - 1;
if ( y < 0 ) {
if ( getGridMode() == GridMode.PERIODIC ) {
y = getGridHeight() - 1;
}
else {
throw new IndexOutOfBoundsException( "As the grid is in mode <" + getGridMode() + "> an index of <" + aPos.toString() + "> is out of bounds!" );
}
}
return y;
}
/**
* To right X position.
*
* @param aPos the pos
*
* @return the int
*/
private int toRightXPosition( Position aPos ) {
int x = aPos.getPositionX() + 1;
if ( x > getGridWidth() - 1 ) {
if ( getGridMode() == GridMode.PERIODIC ) {
x = 0;
}
else {
throw new IndexOutOfBoundsException( "As the grid is in mode <" + getGridMode() + "> an index of <" + aPos.toString() + "> is out of bounds!" );
}
}
return x;
}
/**
* To left Y position.
*
* @param aPos the pos
*
* @return the int
*/
private int toBottomYPosition( Position aPos ) {
int y = aPos.getPositionY() + 1;
if ( y > getGridHeight() - 1 ) {
if ( getGridMode() == GridMode.PERIODIC ) {
y = 0;
}
else {
throw new IndexOutOfBoundsException( "As the grid is in mode <" + getGridMode() + "> an index of <" + aPos.toString() + "> is out of bounds!" );
}
}
return y;
}
/**
* To left X position.
*
* @param aPos the pos
*
* @return the int
*/
private int toLeftXPosition( Position aPos ) {
int x = aPos.getPositionX() - 1;
if ( x < 0 ) {
if ( getGridMode() == GridMode.PERIODIC ) {
x = getGridWidth() - 1;
}
else {
throw new IndexOutOfBoundsException( "As the grid is in mode <" + getGridMode() + "> an index of <" + aPos.toString() + "> is out of bounds!" );
}
}
return x;
}
// /////////////////////////////////////////////////////////////////////////
// INNER CLASSES:
// /////////////////////////////////////////////////////////////////////////
/**
* The Class CheckerboardPlayerObserverImpl.
*/
private class CheckerboardPlayerObserverImpl implements PlayerObserver {
@Override
public void onPlayerEvent( PlayerEvent
aPlayerEvent ) {
try {
fireEvent( aPlayerEvent, ExecutionStrategy.SEQUENTIAL );
}
catch ( VetoException ignore ) {}
}
/**
* {@inheritDoc}
*/
@Override
public void onChangePositionEvent( ChangePositionEvent
aPlayerEvent ) throws VetoException {
try {
fireEvent( aPlayerEvent, ExecutionStrategy.SEQUENTIAL );
}
catch ( VetoException ignore ) {}
}
/**
* {@inheritDoc}
*/
@Override
public void onPositionChangedEvent( PositionChangedEvent
aPlayerEvent ) {
P thePlayer = aPlayerEvent.getSource();
Position thePrecedingPosition = aPlayerEvent.getPrecedingPosition();
updatePlayer( thePlayer, thePrecedingPosition );
try {
fireEvent( aPlayerEvent, ExecutionStrategy.SEQUENTIAL );
}
catch ( VetoException ignore ) {}
}
/**
* {@inheritDoc}
*/
@Override
public void onStateChangedEvent( StateChangedEvent
aPlayerEvent ) {
try {
fireEvent( aPlayerEvent, ExecutionStrategy.SEQUENTIAL );
}
catch ( VetoException ignore ) {}
}
/**
* {@inheritDoc}
*/
@Override
public void onVisibilityChangedEvent( VisibilityChangedEvent
aPlayerEvent ) {
try {
fireEvent( aPlayerEvent, ExecutionStrategy.SEQUENTIAL );
}
catch ( VetoException ignore ) {}
}
/**
* {@inheritDoc}
*/
@Override
public void onDraggabilityChangedEvent( DraggabilityChangedEvent
aPlayerEvent ) {
try {
fireEvent( aPlayerEvent, ExecutionStrategy.SEQUENTIAL );
}
catch ( VetoException ignore ) {}
}
}
}