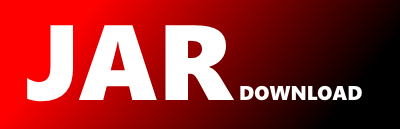
org.refcodes.checkerboard.AbstractPlayer Maven / Gradle / Ivy
Show all versions of refcodes-checkerboard Show documentation
package org.refcodes.checkerboard;
import org.refcodes.controlflow.ExecutionStrategy;
import org.refcodes.exception.VetoException;
import org.refcodes.exception.VetoException.VetoRuntimeException;
import org.refcodes.graphical.Position;
import org.refcodes.observer.AbstractObservable;
/**
* The Class AbstractPlayer.
*
* @param the generic type
* @param the generic type
*/
public abstract class AbstractPlayer
, S> extends AbstractObservable, PlayerEvent> implements Player
{
// /////////////////////////////////////////////////////////////////////////
// STATICS:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// CONSTANTS:
// /////////////////////////////////////////////////////////////////////////
private static final ExecutionStrategy STRATEGY = ExecutionStrategy.SEQUENTIAL;
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private int _posX;
private int _posY;
private S _state;
private boolean _isVisible = true;
private boolean _isDraggable = true;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Empty constructor. Make sure you set the coordinates e.g. by calling
* {@link #setPosition(int, int)} or the like after construction. You may
* also directly construct with coordinates by using
* {@link #AbstractPlayer(Position)} or {@link #AbstractPlayer(int, int)}.
*/
public AbstractPlayer() {}
/**
* Instantiates a new abstract player.
*
* @param aPosX the pos X
* @param aPosY the pos Y
*/
public AbstractPlayer( int aPosX, int aPosY ) {
_posX = aPosX;
_posY = aPosY;
}
/**
* Instantiates a new abstract player.
*
* @param aPosition the position
*/
public AbstractPlayer( Position aPosition ) {
_posX = aPosition.getPositionX();
_posY = aPosition.getPositionY();
}
// /////////////////////////////////////////////////////////////////////////
// INJECTION:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// HANDLERS:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@SuppressWarnings("unchecked")
@Override
public void click() {
try {
ClickedEvent
theEvent = new ClickedEvent
( (P) this );
fireEvent( theEvent, STRATEGY );
}
catch ( VetoException ignore ) {}
}
/**
* With position.
*
* @param aPosX the pos X
* @param aPosY the pos Y
*
* @return the p
*
* @throws VetoRuntimeException the veto runtime exception
*/
@SuppressWarnings("unchecked")
@Override
public P withPosition( int aPosX, int aPosY ) throws VetoRuntimeException {
setPosition( aPosX, aPosY );
return (P) this;
}
/**
* With position.
*
* @param aPosition the position
*
* @return the p
*
* @throws VetoRuntimeException the veto runtime exception
*/
@SuppressWarnings("unchecked")
@Override
public P withPosition( Position aPosition ) throws VetoRuntimeException {
setPosition( aPosition );
return (P) this;
}
/**
* Sets the position.
*
* @param aPosX the pos X
* @param aPosY the pos Y
*
* @throws VetoRuntimeException the veto runtime exception
*/
@SuppressWarnings("unchecked")
@Override
public void setPosition( int aPosX, int aPosY ) throws VetoRuntimeException {
if ( aPosX != _posX || aPosY != _posY ) {
ChangePositionEvent
theVetoable = new ChangePositionEvent
( aPosX, aPosY, _posX, _posY, (P) this );
try {
fireEvent( theVetoable, STRATEGY );
}
catch ( VetoException e ) {
throw new VetoRuntimeException( e );
}
PositionChangedEvent
theEvent = new PositionChangedEvent<>( aPosX, aPosY, _posX, _posY, (P) this );
_posX = aPosX;
_posY = aPosY;
try {
fireEvent( theEvent, STRATEGY );
}
catch ( VetoException ignore ) {}
}
}
/**
* {@inheritDoc}
*/
@Override
public void setPosition( Position aPosition ) throws VetoRuntimeException {
setPosition( aPosition.getPositionX(), aPosition.getPositionY() );
}
/**
* With position Y.
*
* @param aPosY the pos Y
*
* @return the p
*
* @throws VetoRuntimeException the veto runtime exception
*/
@SuppressWarnings("unchecked")
@Override
public P withPositionY( int aPosY ) throws VetoRuntimeException {
setPositionY( aPosY );
return (P) this;
}
/**
* Sets the position Y.
*
* @param aPosY the new position Y
*
* @throws VetoRuntimeException the veto runtime exception
*/
@SuppressWarnings("unchecked")
@Override
public void setPositionY( int aPosY ) throws VetoRuntimeException {
if ( aPosY != _posY ) {
PositionChangedEvent
theEvent = new PositionChangedEvent<>( _posX, aPosY, _posX, _posY, (P) this );
_posY = aPosY;
try {
fireEvent( theEvent, STRATEGY );
}
catch ( VetoException ignore ) {}
}
}
/**
* With position X.
*
* @param aPosX the pos X
*
* @return the p
*
* @throws VetoRuntimeException the veto runtime exception
*/
@SuppressWarnings("unchecked")
@Override
public P withPositionX( int aPosX ) throws VetoRuntimeException {
setPositionX( aPosX );
return (P) this;
}
/**
* Sets the state.
*
* @param aState the new state
*/
@SuppressWarnings("unchecked")
@Override
public void setStatus( S aState ) {
if ( aState != _state ) {
StateChangedEvent
theEvent = new StateChangedEvent<>( aState, _state, (P) this );
_state = aState;
try {
fireEvent( theEvent, STRATEGY );
}
catch ( VetoException ignore ) {}
}
}
/**
* With state.
*
* @param aState the state
*
* @return the p
*/
@SuppressWarnings("unchecked")
@Override
public P withStatus( S aState ) {
setStatus( aState );
return (P) this;
}
/**
* Sets the position X.
*
* @param aPosX the new position X
*/
@SuppressWarnings("unchecked")
@Override
public void setPositionX( int aPosX ) {
if ( aPosX != _posX ) {
PositionChangedEvent
theEvent = new PositionChangedEvent
( aPosX, _posY, _posX, _posY, (P) this );
_posX = aPosX;
try {
fireEvent( theEvent, STRATEGY );
}
catch ( VetoException ignore ) {}
}
}
/**
* Sets the visible.
*
* @param isVisible the new visible
*/
@SuppressWarnings("unchecked")
@Override
public void setVisible( boolean isVisible ) {
if ( isVisible != _isVisible ) {
VisibilityChangedEvent
theEvent = new VisibilityChangedEvent
( (P) this );
_isVisible = isVisible;
try {
fireEvent( theEvent, STRATEGY );
}
catch ( VetoException ignore ) {}
}
}
/**
* With show.
*
* @return the p
*/
@SuppressWarnings("unchecked")
@Override
public P withShow() {
setVisible( true );
return (P) this;
}
/**
* With hide.
*
* @return the p
*/
@SuppressWarnings("unchecked")
@Override
public P withHide() {
setVisible( false );
return (P) this;
}
/**
* With visible.
*
* @param isVisible the is visible
*
* @return the p
*/
@SuppressWarnings("unchecked")
@Override
public P withVisible( boolean isVisible ) {
setVisible( isVisible );
return (P) this;
}
/**
* {@inheritDoc}
*/
@Override
public void show() {
setVisible( true );
}
/**
* {@inheritDoc}
*/
@Override
public void hide() {
setVisible( false );
}
/**
* {@inheritDoc}
*/
@Override
public boolean isVisible() {
return _isVisible;
}
/**
* Sets the draggable.
*
* @param isDraggable the new draggable
*/
@SuppressWarnings("unchecked")
@Override
public void setDraggable( boolean isDraggable ) {
if ( isDraggable != _isDraggable ) {
DraggabilityChangedEvent
theEvent = new DraggabilityChangedEvent
( (P) this );
_isDraggable = isDraggable;
try {
fireEvent( theEvent, STRATEGY );
}
catch ( VetoException ignore ) {}
}
}
/**
* With draggable.
*
* @return the p
*/
@SuppressWarnings("unchecked")
@Override
public P withDraggable() {
setDraggable( true );
return (P) this;
}
/**
* With stationary.
*
* @return the p
*/
@SuppressWarnings("unchecked")
@Override
public P withStationary() {
setDraggable( false );
return (P) this;
}
/**
* With draggable.
*
* @param isDraggable the is draggable
*
* @return the p
*/
@SuppressWarnings("unchecked")
@Override
public P withDraggable( boolean isDraggable ) {
setDraggable( isDraggable );
return (P) this;
}
/**
* {@inheritDoc}
*/
@Override
public void draggable() {
setDraggable( true );
}
/**
* {@inheritDoc}
*/
@Override
public void stationary() {
setDraggable( false );
}
/**
* {@inheritDoc}
*/
@Override
public boolean isDraggable() {
return _isDraggable;
}
/**
* {@inheritDoc}
*/
@Override
public int getPositionX() {
return _posX;
}
/**
* {@inheritDoc}
*/
@Override
public int getPositionY() {
return _posY;
}
/**
* {@inheritDoc}
*/
@Override
public S getStatus() {
return _state;
}
/**
* {@inheritDoc}
*/
@Override
public String toString() {
return getClass().getSimpleName() + "(" + _posX + ":" + _posY + ", " + getStatus() + ")@" + hashCode();
}
// /////////////////////////////////////////////////////////////////////////
// HOOKS:
// /////////////////////////////////////////////////////////////////////////
/**
* Fire event.
*
* @param aEvent the event
* @param aObserver the observer
* @param aExecutionStrategy the execution strategy
*
* @return true, if successful
*
* @throws VetoException the veto exception
*/
@SuppressWarnings("unchecked")
@Override
protected boolean fireEvent( PlayerEvent
aEvent, PlayerObserver
aObserver, ExecutionStrategy aExecutionStrategy ) throws VetoException {
aObserver.onPlayerEvent( aEvent );
if ( aEvent instanceof ChangePositionEvent ) {
aObserver.onChangePositionEvent( (ChangePositionEvent
) aEvent );
}
if ( aEvent instanceof PositionChangedEvent ) {
aObserver.onPositionChangedEvent( (PositionChangedEvent
) aEvent );
}
if ( aEvent instanceof ClickedEvent ) {
aObserver.onClickedEvent( (ClickedEvent
) aEvent );
}
if ( aEvent instanceof StateChangedEvent ) {
aObserver.onStateChangedEvent( (StateChangedEvent
) aEvent );
}
if ( aEvent instanceof VisibilityChangedEvent ) {
aObserver.onVisibilityChangedEvent( (VisibilityChangedEvent
) aEvent );
}
if ( aEvent instanceof DraggabilityChangedEvent ) {
aObserver.onDraggabilityChangedEvent( (DraggabilityChangedEvent
) aEvent );
}
return true;
}
// /////////////////////////////////////////////////////////////////////////
// HELPER:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// INNER CLASSES:
// /////////////////////////////////////////////////////////////////////////
}