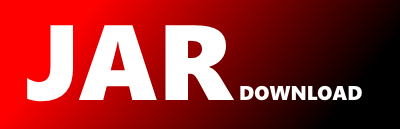
org.refcodes.checkerboard.Players Maven / Gradle / Ivy
Show all versions of refcodes-checkerboard Show documentation
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.checkerboard;
import java.lang.reflect.Array;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
/**
* The Interface Players.
*
* @author steiner
*
* @param the generic type
*/
public interface Players
> {
// /////////////////////////////////////////////////////////////////////////
// CONSTANTS:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* Gets the players.
*
* @return the players
*/
List
getPlayers();
/**
* Players.
*
* @return the iterator
*/
Iterator
players();
/**
* Checks for player.
*
* @param aElement the element
*
* @return true, if successful
*/
boolean hasPlayer( P aElement );
/**
* Player count.
*
* @return the int
*/
int playerCount();
/**
* Checks for players.
*
* @return true, if successful
*/
boolean hasPlayers();
/**
* Clear players.
*/
void clearPlayers();
/**
* Put player.
*
* @param aElement the element
*
* @return the p
*/
P putPlayer( P aElement );
/**
* Removes the player.
*
* @param aElement the element
*
* @return true, if successful
*/
boolean removePlayer( P aElement );
/**
* Returns an array containing all the players being of the given type.
*
* @param The type of the player to seek for.
* @param aType The type of the player to find.
*
* @return The according array, if no players were found then the array is
* empty.
*/
@SuppressWarnings("unchecked")
default PLAYER[] players( Class aType ) {
List thePlayers = new ArrayList<>();
Iterator
e = players();
P ePlayer;
while ( e.hasNext() ) {
ePlayer = e.next();
if ( aType.isAssignableFrom( ePlayer.getClass() ) && ePlayer.getClass().isAssignableFrom( aType ) ) {
thePlayers.add( ePlayer );
}
}
PLAYER[] theResult = (PLAYER[]) Array.newInstance( aType, thePlayers.size() );
theResult = thePlayers.toArray( theResult );
return theResult;
}
/**
* Returns the first player found being of the given type.
*
* @param The type of the player to seek for.
* @param aType The type of the player to find.
*
* @return The according player or null if no players were found matching
* the given type.
*/
@SuppressWarnings("unchecked")
default PLAYER firstPlayer( Class aType ) {
Iterator e = players();
P ePlayer;
while ( e.hasNext() ) {
ePlayer = e.next();
if ( aType.isAssignableFrom( ePlayer.getClass() ) && ePlayer.getClass().isAssignableFrom( aType ) ) {
return (PLAYER) ePlayer;
}
}
return null;
}
}