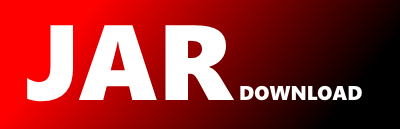
org.refcodes.collection.CollectionUtility Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-collection Show documentation
Show all versions of refcodes-collection Show documentation
Artifact with collection definitions and collections.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// =============================================================================
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// =============================================================================
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// together with the GPL linking exception applied; as being applied by the GNU
// Classpath ("http://www.gnu.org/software/classpath/license.html")
// =============================================================================
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// =============================================================================
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.collection;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
public final class CollectionUtility {
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Private empty constructor to prevent instantiation as of being a utility
* with just static public methods.
*/
private CollectionUtility() {}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* Creates a new list and copies the content of the iterator into the list.
* Caution: The iterator is empty afterwards! You may easily retrieve an
* iterator with the same elements as the provided one by using the method
* 'iterator()' of the returned list.
*
* @param anIterator Description of the Parameter
* @return Description of the Return Value
*/
public static List toArrayList( Iterator extends E> anIterator ) {
List theList = new ArrayList();
if ( anIterator != null ) while ( anIterator.hasNext() )
theList.add( anIterator.next() );
return theList;
}
/**
* Returns the max length of the arrays passed to this method.
*
* @param aObjects The arrays of which the length of the one with the most
* elements is returned.
*
* @return The max length of elements found in the provided arrays or -1 if
* all the arrays were null.
*/
@SafeVarargs
public static int toMaxLength( T[]... aObjects ) {
int theMaxLength = -1;
for ( Object[] eArray : aObjects ) {
if ( eArray != null && eArray.length > theMaxLength ) {
theMaxLength = eArray.length;
}
}
return theMaxLength;
}
/**
* Returns the max size of the collections passed to this method.
*
* @param aCollections The collections of which the size of the one with the
* most elements is returned.
*
* @return The max length of elements found in the provided collections or
* -1 if all the collections were null.
*/
public static int toMaxLength( Collection>... aCollections ) {
int theMaxLength = -1;
for ( Collection> eCollection : aCollections ) {
if ( eCollection != null && eCollection.size() > theMaxLength ) {
theMaxLength = eCollection.size();
}
}
return theMaxLength;
}
// /////////////////////////////////////////////////////////////////////////
// CSV:
// /////////////////////////////////////////////////////////////////////////
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy