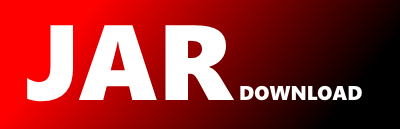
org.refcodes.component.ClosableHandle Maven / Gradle / Ivy
package org.refcodes.component;
import org.refcodes.component.ConnectionOpenable.ConnectionOpenAutomaton;
/**
* The {@link ClosableHandle} interface defines those methods related to the
* handle based close life-cycle.
*
* The handle reference requires the {@link Closable} interface to be
* implemented.
*
* @param The type of the handle.
*/
public interface ClosableHandle {
/**
* Determines whether the handle reference is closable by implementing the
* {@link Closable} interface.
*
* @param aHandle The handle to test whether the reference provides the
* according functionality.
*
* @return True in case the reference provides the according functionality.
*
* @throws UnknownHandleRuntimeException in case the handle is unknown.
*/
boolean hasClosable( H aHandle ) throws UnknownHandleRuntimeException;
/**
* Closes or pre-closes (flush) the component identified by the given
* handle. Throws a {@link CloseException} as upon close we may have to do
* things like flushing buffers which can fail (and would otherwise fail
* unhandled or even worse unnoticed).
*
*
* @param aHandle The handle identifying the component.
*
* @throws CloseException Thrown in case closing or pre-closing (flushing)
* fails.
*
* @throws UnsupportedHandleOperationRuntimeException in case the reference
* of the handle does not support the requested operation.
*
* @throws UnknownHandleRuntimeException in case the given handle is
* unknown.
*
* @throws IllegaleHandleStateChangeRuntimeException Thrown in case a state
* change is not possible due to the current state the referenced
* component is in.
*/
void close( H aHandle ) throws CloseException, UnknownHandleRuntimeException, UnsupportedHandleOperationRuntimeException, IllegaleHandleStateChangeRuntimeException;
/**
* The {@link CloseAutomatonHandle} interface defines those methods related
* to the handle based close life-cycle.
*
* The handle reference requires the {@link ConnectionOpenAutomaton}
* interface to be implemented.
*
* @param The type of the handle.
*/
public interface CloseAutomatonHandle extends ClosableHandle {
/**
* Determines whether the handle reference is closable by implementing
* the {@link ConnectionOpenAutomaton} interface.
*
* @param aHandle The handle to test whether the reference provides the
* according functionality.
*
* @return True in case the reference provides the according
* functionality.
*
* @throws UnknownHandleRuntimeException in case the handle is unknown.
*/
boolean hasCloseAutomaton( H aHandle ) throws UnknownHandleRuntimeException;
/**
* Determines whether the component identified by the given handle may
* get closed/disconnected.
*
* @param aHandle The handle identifying the component.
*
* @return True if {@link #close(Object)} is possible.
*
* @throws UnsupportedHandleOperationRuntimeException in case the
* reference of the handle does not support the requested
* operation.
*
* @throws UnknownHandleRuntimeException in case the given handle is
* unknown.
*/
boolean isClosable( H aHandle ) throws UnknownHandleRuntimeException, UnsupportedHandleOperationRuntimeException;
/**
* Determines whether the component (its connection) identified by the
* given handle is closed (disconnected).
*
* @param aHandle The handle identifying the component.
*
* @return True in case of being closed, else false.
*
* @throws UnsupportedHandleOperationRuntimeException in case the
* reference of the handle does not support the requested
* operation.
*
* @throws UnknownHandleRuntimeException in case the given handle is
* unknown.
*/
boolean isClosed( H aHandle ) throws UnknownHandleRuntimeException, UnsupportedHandleOperationRuntimeException;
}
}