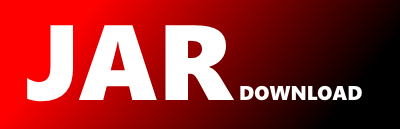
org.refcodes.component.ComponentUtility Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-component Show documentation
Show all versions of refcodes-component Show documentation
Artifact with common component definitions and functionality.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// =============================================================================
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// =============================================================================
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// together with the GPL linking exception applied; as being applied by the GNU
// Classpath ("http://www.gnu.org/software/classpath/license.html")
// =============================================================================
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// =============================================================================
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.component;
import java.io.Flushable;
import java.io.IOException;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashSet;
import java.util.Set;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Future;
import org.refcodes.controlflow.ControlFlowUtility;
import org.refcodes.controlflow.ExecutionStrategy;
import org.refcodes.exception.HiddenException;
import org.refcodes.mixin.Disposable.DisposeSupport;
public final class ComponentUtility {
// /////////////////////////////////////////////////////////////////////////
// CONSTANTS:
// /////////////////////////////////////////////////////////////////////////
private static CallableFactory _initializeCallableFactoryImpl = new CallableFactory() {
@Override
public Callable toCallable( Initializable aComponent, Object... aArguments ) {
return new InitializeCallableImpl( aComponent );
}
@Override
public Class getType() {
return Initializable.class;
}
};
@SuppressWarnings("rawtypes")
private static CallableFactory _configureCallableFactoryImpl = new CallableFactory() {
@SuppressWarnings({
"unchecked"
})
@Override
public Callable toCallable( Configurable aComponent, Object... aArguments ) {
return new ConfigureCallableImpl( aComponent, aArguments[0] );
}
@Override
public Class getType() {
return Configurable.class;
}
};
private static CallableFactory _startCallableFactoryImpl = new CallableFactory() {
@Override
public Callable toCallable( Startable aComponent, Object... aArguments ) {
return new StartCallableImpl( aComponent );
}
@Override
public Class getType() {
return Startable.class;
}
};
private static CallableFactory _pauseCallableFactoryImpl = new CallableFactory() {
@Override
public Callable toCallable( Pausable aComponent, Object... aArguments ) {
return new PauseCallableImpl( aComponent );
}
@Override
public Class getType() {
return Pausable.class;
}
};
private static CallableFactory _resumeCallableFactoryImpl = new CallableFactory() {
@Override
public Callable toCallable( Resumable aComponent, Object... aArguments ) {
return new ResumeCallableImpl( aComponent );
}
@Override
public Class getType() {
return Resumable.class;
}
};
private static CallableFactory _stopCallableFactoryImpl = new CallableFactory() {
@Override
public Callable toCallable( Stoppable aComponent, Object... aArguments ) {
return new StopCallableImpl( aComponent );
}
@Override
public Class getType() {
return Stoppable.class;
}
};
private static CallableFactory _destroyCallableFactoryImpl = new CallableFactory() {
@Override
public Callable toCallable( Destroyable aComponent, Object... aArguments ) {
return new DestroyCallableImpl( aComponent );
}
@Override
public Class getType() {
return Destroyable.class;
}
};
private static CallableFactory _decomposeCallableFactoryImpl = new CallableFactory() {
@Override
public Callable toCallable( Decomposeable aComponent, Object... aArguments ) {
return new DecomposeCallableImpl( aComponent );
}
@Override
public Class getType() {
return Decomposeable.class;
}
};
private static CallableFactory _disposeCallableFactoryImpl = new CallableFactory() {
@Override
public Callable toCallable( DisposeSupport aComponent, Object... aArguments ) {
return new DisposeCallableImpl( aComponent );
}
@Override
public Class getType() {
return DisposeSupport.class;
}
};
private static CallableFactory _openCallableFactoryImpl = new CallableFactory() {
@Override
public Callable toCallable( Openable aComponent, Object... aArguments ) {
return new OpenCallableImpl( aComponent );
}
@Override
public Class getType() {
return Openable.class;
}
};
@SuppressWarnings("rawtypes")
private static CallableFactory _connectCallableFactoryImpl = new CallableFactory() {
@SuppressWarnings("unchecked")
@Override
public Callable toCallable( ConnectionOpenable aComponent, Object... aArguments ) {
return new ConnectCallableImpl( aComponent, aArguments[0] );
}
@Override
public Class getType() {
return ConnectionOpenable.class;
}
};
@SuppressWarnings("rawtypes")
private static CallableFactory _ioConnectCallableFactoryImpl = new CallableFactory() {
@SuppressWarnings("unchecked")
@Override
public Callable toCallable( BidirectionalConnectionOpenable aComponent, Object... aArguments ) {
return new IoConnectCallableImpl( aComponent, aArguments[0], aArguments[1] );
}
@Override
public Class getType() {
return BidirectionalConnectionOpenable.class;
}
};
private static CallableFactory _closeCallableFactoryImpl = new CallableFactory() {
@Override
public Callable toCallable( Closable aComponent, Object... aArguments ) {
return new CloseCallableImpl( aComponent );
}
@Override
public Class getType() {
return Closable.class;
}
};
private static CallableFactory _flushCallableFactoryImpl = new CallableFactory() {
@Override
public Callable toCallable( Flushable aComponent, Object... aArguments ) {
return new FlushCallableImpl( aComponent );
}
@Override
public Class getType() {
return Flushable.class;
}
};
private static CallableFactory _resetCallableFactoryImpl = new CallableFactory() {
@Override
public Callable toCallable( Resetable aComponent, Object... aArguments ) {
return new ResetCallableImpl( aComponent );
}
@Override
public Class getType() {
return Resetable.class;
}
};
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Private empty constructor to prevent instantiation as of being a utility
* with just static public methods.
*/
private ComponentUtility() {}
// /////////////////////////////////////////////////////////////////////////
// INITIALIZE:
// /////////////////////////////////////////////////////////////////////////
/**
* Helper method, initializes the given {@link Component} in case it is
* {@link Initializable}.
*
* @param aComponent The {@link Component} to initialize.
*
* @throws InitializeException Thrown in case initialization failed.
*/
public static void initialize( Object aComponent ) throws InitializeException {
if ( aComponent instanceof Initializable ) {
((Initializable) aComponent).initialize();
}
}
/**
* Helper method for initializing all {@link Initializable}
* {@link Component} instances found in the provided {@link Collection}. The
* strategy with which the {@link Component} instances are processed is
* defined with the provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when initializing
* the {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to initialize (in case them implement
* the {@link Initializable} interface).
*
* @throws InitializeException in case initialization of at least one
* {@link Initializable} instance failed.
*/
public static void initialize( ExecutionStrategy aComponentExecutionStrategy, Collection> aComponents ) throws InitializeException {
initialize( aComponentExecutionStrategy, ControlFlowUtility.createDaemonExecutorService(), aComponents );
}
/**
* Helper method for initializing all {@link Initializable}
* {@link Component} instances found in the provided {@link Collection}. The
* strategy with which the {@link Component} instances are processed is
* defined with the provided {@link ExecutionStrategy}. An
* {@link ExecutorService} can be provided in case some EJB container is to
* use a managed {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when initializing
* the {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws InitializeException in case initialization of at least one
* {@link Initializable} instance failed.
*/
public static void initialize( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, Collection> aComponents ) throws InitializeException {
try {
execute( aComponentExecutionStrategy, aExecutorService, _initializeCallableFactoryImpl, aComponents, (Object[]) null );
}
catch ( InitializeException e ) {
throw e;
}
catch ( Exception e ) {
throw new InitializeException( e );
}
}
/**
* Helper method for initializing all {@link Initializable}
* {@link Component} instances found in the provided {@link Collection}. The
* strategy with which the {@link Component} instances are processed is
* defined with the provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when initializing
* the {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to initialize (in case them implement
* the {@link Initializable} interface).
*
* @throws InitializeException in case initialization of at least one
* {@link Initializable} instance failed.
*/
@SafeVarargs
public static void initialize( ExecutionStrategy aComponentExecutionStrategy, Object... aComponents ) throws InitializeException {
initialize( aComponentExecutionStrategy, Arrays.asList( aComponents ) );
}
/**
* Helper method for initializing all {@link Initializable}
* {@link Component} instances found in the provided {@link Collection}. The
* strategy with which the {@link Component} instances are processed is
* defined with the provided {@link ExecutionStrategy}. An
* {@link ExecutorService} can be provided in case some EJB container is to
* use a managed {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when initializing
* the {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws InitializeException in case initialization of at least one
* {@link Initializable} instance failed.
*/
@SafeVarargs
public static void initialize( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, Object... aComponents ) throws InitializeException {
initialize( aComponentExecutionStrategy, aExecutorService, Arrays.asList( aComponents ) );
}
// /////////////////////////////////////////////////////////////////////////
// CONFIGURE:
// /////////////////////////////////////////////////////////////////////////
/**
* Helper method, configuring the given {@link Component} in case it is
* {@link Configurable}.
*
* @param aComponent The {@link Component} to configure.
*
* @throws InitializeException Thrown in case initialization failed.
*/
@SuppressWarnings({
"unchecked", "rawtypes"
})
public static void initialize( Object aComponent, CTX aContext ) throws ConfigureException {
if ( aComponent instanceof Configurable> ) {
((Configurable) aComponent).initialize( aContext );
}
}
/**
* Helper method for configuring all {@link Configurable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when initializing
* the {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to initialize (in case them implement
* the {@link Configurable} interface).
*
* @throws InitializeException in case initialization of at least one
* {@link Configurable} instance failed.
*/
public static void initialize( ExecutionStrategy aComponentExecutionStrategy, CTX aContext, Collection> aComponents ) throws ConfigureException {
initialize( aComponentExecutionStrategy, ControlFlowUtility.createDaemonExecutorService(), aContext, aComponents );
}
/**
* Helper method for configuring all {@link Configurable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}. An {@link ExecutorService} can be
* provided in case some EJB container is to use a managed
* {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when initializing
* the {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws InitializeException in case initialization of at least one
* {@link Configurable} instance failed.
*/
public static void initialize( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, CTX aContext, Collection> aComponents ) throws ConfigureException {
try {
execute( aComponentExecutionStrategy, aExecutorService, _configureCallableFactoryImpl, aComponents, aContext );
}
catch ( ConfigureException e ) {
throw e;
}
catch ( Exception e ) {
throw new ConfigureException( aContext, e );
}
}
/**
* Helper method for configuring all {@link ConfigureException}
* {@link Component} instances found in the provided {@link Collection}. The
* strategy with which the {@link Component} instances are processed is
* defined with the provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when initializing
* the {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to initialize (in case them implement
* the {@link ConfigureException} interface).
*
* @throws InitializeException in case initialization of at least one
* {@link ConfigureException} instance failed.
*/
@SafeVarargs
public static void initialize( ExecutionStrategy aComponentExecutionStrategy, CTX aContext, Object... aComponents ) throws ConfigureException {
initialize( aComponentExecutionStrategy, aContext, Arrays.asList( aComponents ) );
}
/**
* Helper method for configuring all {@link ConfigureException}
* {@link Component} instances found in the provided {@link Collection}. The
* strategy with which the {@link Component} instances are processed is
* defined with the provided {@link ExecutionStrategy}. An
* {@link ExecutorService} can be provided in case some EJB container is to
* use a managed {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when initializing
* the {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws InitializeException in case initialization of at least one
* {@link ConfigureException} instance failed.
*/
@SafeVarargs
public static void initialize( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, CTX aContext, Object... aComponents ) throws ConfigureException {
initialize( aComponentExecutionStrategy, aExecutorService, aContext, Arrays.asList( aComponents ) );
}
// /////////////////////////////////////////////////////////////////////////
// START:
// /////////////////////////////////////////////////////////////////////////
/**
* Helper method, starts the given {@link Component} in case it is
* {@link Startable}.
*
* @param aComponent The {@link Component} to start.
*
* @throws StartException Thrown in case initialization failed.
*/
public static void start( Object aComponent ) throws StartException {
if ( aComponent instanceof Startable ) {
((Startable) aComponent).start();
}
}
/**
* Helper method for starting all {@link Startable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when starting the
* {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to start (in case them implement the
* {@link Startable} interface).
*
* @throws StartException in case initialization of at least one
* {@link Startable} instance failed.
*/
public static void start( ExecutionStrategy aComponentExecutionStrategy, Collection> aComponents ) throws StartException {
start( aComponentExecutionStrategy, ControlFlowUtility.createDaemonExecutorService(), aComponents );
}
/**
* Helper method for starting all {@link Startable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}. An {@link ExecutorService} can be
* provided in case some EJB container is to use a managed
* {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when starting the
* {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws StartException in case initialization of at least one
* {@link Startable} instance failed.
*/
public static void start( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, Collection> aComponents ) throws StartException {
try {
execute( aComponentExecutionStrategy, aExecutorService, _startCallableFactoryImpl, aComponents, (Object[]) null );
}
catch ( StartException e ) {
throw e;
}
catch ( Exception e ) {
throw new StartException( e );
}
}
/**
* Helper method for starting all {@link Startable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when starting the
* {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to start (in case them implement the
* {@link Startable} interface).
*
* @throws StartException in case initialization of at least one
* {@link Startable} instance failed.
*/
@SafeVarargs
public static void start( ExecutionStrategy aComponentExecutionStrategy, Object... aComponents ) throws StartException {
start( aComponentExecutionStrategy, Arrays.asList( aComponents ) );
}
/**
* Helper method for starting all {@link Startable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}. An {@link ExecutorService} can be
* provided in case some EJB container is to use a managed
* {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when starting the
* {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws StartException in case initialization of at least one
* {@link Startable} instance failed.
*/
@SafeVarargs
public static void start( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, Object... aComponents ) throws StartException {
start( aComponentExecutionStrategy, aExecutorService, Arrays.asList( aComponents ) );
}
// /////////////////////////////////////////////////////////////////////////
// PAUSE:
// /////////////////////////////////////////////////////////////////////////
/**
* Helper method, pauses the given {@link Component} in case it is
* {@link Pausable}.
*
* @param aComponent The {@link Component} to pause.
*
* @throws PauseException Thrown in case initialization failed.
*/
public static void pause( Object aComponent ) throws PauseException {
if ( aComponent instanceof Pausable ) {
((Pausable) aComponent).pause();
}
}
/**
* Helper method for pausing all {@link Pausable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when pausing the
* {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to pause (in case them implement the
* {@link Pausable} interface).
*
* @throws PauseException in case initialization of at least one
* {@link Pausable} instance failed.
*/
public static void pause( ExecutionStrategy aComponentExecutionStrategy, Collection> aComponents ) throws PauseException {
pause( aComponentExecutionStrategy, ControlFlowUtility.createDaemonExecutorService(), aComponents );
}
/**
* Helper method for pausing all {@link Pausable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}. An {@link ExecutorService} can be
* provided in case some EJB container is to use a managed
* {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when pausing the
* {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws PauseException in case initialization of at least one
* {@link Pausable} instance failed.
*/
public static void pause( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, Collection> aComponents ) throws PauseException {
try {
execute( aComponentExecutionStrategy, aExecutorService, _pauseCallableFactoryImpl, aComponents, (Object[]) null );
}
catch ( PauseException e ) {
throw e;
}
catch ( Exception e ) {
throw new PauseException( e );
}
}
/**
* Helper method for pausing all {@link Pausable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when pausing the
* {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to pause (in case them implement the
* {@link Pausable} interface).
*
* @throws PauseException in case initialization of at least one
* {@link Pausable} instance failed.
*/
@SafeVarargs
public static void pause( ExecutionStrategy aComponentExecutionStrategy, Object... aComponents ) throws PauseException {
pause( aComponentExecutionStrategy, Arrays.asList( aComponents ) );
}
/**
* Helper method for pausing all {@link Pausable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}. An {@link ExecutorService} can be
* provided in case some EJB container is to use a managed
* {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when pausing the
* {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws PauseException in case initialization of at least one
* {@link Pausable} instance failed.
*/
@SafeVarargs
public static void pause( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, Object... aComponents ) throws PauseException {
pause( aComponentExecutionStrategy, aExecutorService, Arrays.asList( aComponents ) );
}
// /////////////////////////////////////////////////////////////////////////
// RESUME:
// /////////////////////////////////////////////////////////////////////////
/**
* Helper method, resumes the given {@link Component} in case it is
* {@link Resumable}.
*
* @param aComponent The {@link Component} to resume.
*
* @throws ResumeException Thrown in case initialization failed.
*/
public static void resume( Object aComponent ) throws ResumeException {
if ( aComponent instanceof Resumable ) {
((Resumable) aComponent).resume();
}
}
/**
* Helper method for resuming all {@link Resumable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when resuming the
* {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to resume (in case them implement the
* {@link Resumable} interface).
*
* @throws ResumeException in case initialization of at least one
* {@link Resumable} instance failed.
*/
public static void resume( ExecutionStrategy aComponentExecutionStrategy, Collection> aComponents ) throws ResumeException {
resume( aComponentExecutionStrategy, ControlFlowUtility.createDaemonExecutorService(), aComponents );
}
/**
* Helper method for resuming all {@link Resumable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}. An {@link ExecutorService} can be
* provided in case some EJB container is to use a managed
* {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when resuming the
* {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws ResumeException in case initialization of at least one
* {@link Resumable} instance failed.
*/
public static void resume( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, Collection> aComponents ) throws ResumeException {
try {
execute( aComponentExecutionStrategy, aExecutorService, _resumeCallableFactoryImpl, aComponents, (Object[]) null );
}
catch ( ResumeException e ) {
throw e;
}
catch ( Exception e ) {
throw new ResumeException( e );
}
}
/**
* Helper method for resuming all {@link Resumable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when resuming the
* {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to resume (in case them implement the
* {@link Resumable} interface).
*
* @throws ResumeException in case initialization of at least one
* {@link Resumable} instance failed.
*/
@SafeVarargs
public static void resume( ExecutionStrategy aComponentExecutionStrategy, Object... aComponents ) throws ResumeException {
resume( aComponentExecutionStrategy, Arrays.asList( aComponents ) );
}
/**
* Helper method for resuming all {@link Resumable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}. An {@link ExecutorService} can be
* provided in case some EJB container is to use a managed
* {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when resuming the
* {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws ResumeException in case initialization of at least one
* {@link Resumable} instance failed.
*/
@SafeVarargs
public static void resume( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, Object... aComponents ) throws ResumeException {
resume( aComponentExecutionStrategy, aExecutorService, Arrays.asList( aComponents ) );
}
// /////////////////////////////////////////////////////////////////////////
// STOP:
// /////////////////////////////////////////////////////////////////////////
/**
* Helper method, stops the given {@link Component} in case it is
* {@link Stoppable}.
*
* @param aComponent The {@link Component} to stop.
*
* @throws StopException Thrown in case initialization failed.
*/
public static void stop( Object aComponent ) throws StopException {
if ( aComponent instanceof Stoppable ) {
((Stoppable) aComponent).stop();
}
}
/**
* Helper method for stopping all {@link Stoppable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when stoping the
* {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to stop (in case them implement the
* {@link Stoppable} interface).
*
* @throws StopException in case initialization of at least one
* {@link Stoppable} instance failed.
*/
public static void stop( ExecutionStrategy aComponentExecutionStrategy, Collection> aComponents ) throws StopException {
stop( aComponentExecutionStrategy, ControlFlowUtility.createDaemonExecutorService(), aComponents );
}
/**
* Helper method for stopping all {@link Stoppable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}. An {@link ExecutorService} can be
* provided in case some EJB container is to use a managed
* {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when stoping the
* {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws StopException in case initialization of at least one
* {@link Stoppable} instance failed.
*/
public static void stop( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, Collection> aComponents ) throws StopException {
try {
execute( aComponentExecutionStrategy, aExecutorService, _stopCallableFactoryImpl, aComponents, (Object[]) null );
}
catch ( StopException e ) {
throw e;
}
catch ( Exception e ) {
throw new StopException( e );
}
}
/**
* Helper method for stoping all {@link Stoppable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when stoping the
* {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to stop (in case them implement the
* {@link Stoppable} interface).
*
* @throws StopException in case initialization of at least one
* {@link Stoppable} instance failed.
*/
@SafeVarargs
public static void stop( ExecutionStrategy aComponentExecutionStrategy, Object... aComponents ) throws StopException {
stop( aComponentExecutionStrategy, Arrays.asList( aComponents ) );
}
/**
* Helper method for stoping all {@link Stoppable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}. An {@link ExecutorService} can be
* provided in case some EJB container is to use a managed
* {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when stoping the
* {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws StopException in case initialization of at least one
* {@link Stoppable} instance failed.
*/
@SafeVarargs
public static void stop( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, Object... aComponents ) throws StopException {
stop( aComponentExecutionStrategy, aExecutorService, Arrays.asList( aComponents ) );
}
// /////////////////////////////////////////////////////////////////////////
// DESTROY:
// /////////////////////////////////////////////////////////////////////////
/**
* Helper method, destroys the given {@link Component} in case it is
* {@link Destroyable}.
*
* @param aComponent The {@link Component} to destroy.
*
* @throws DestroyException Thrown in case initialization failed.
*/
public static void destroy( Object aComponent ) {
if ( aComponent instanceof Destroyable ) {
((Destroyable) aComponent).destroy();
}
}
/**
* Helper method for destroying all {@link Destroyable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when destroying
* the {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to destroy (in case them implement the
* {@link Destroyable} interface).
*
* @throws DestroyException in case initialization of at least one
* {@link Destroyable} instance failed.
*/
public static void destroy( ExecutionStrategy aComponentExecutionStrategy, Collection> aComponents ) {
destroy( aComponentExecutionStrategy, ControlFlowUtility.createDaemonExecutorService(), aComponents );
}
/**
* Helper method for destroying all {@link Destroyable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}. An {@link ExecutorService} can be
* provided in case some EJB container is to use a managed
* {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when destroying
* the {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws DestroyException in case initialization of at least one
* {@link Destroyable} instance failed.
*/
public static void destroy( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, Collection> aComponents ) {
try {
execute( aComponentExecutionStrategy, aExecutorService, _destroyCallableFactoryImpl, aComponents, (Object[]) null );
}
catch ( Exception e ) {
throw new HiddenException( e );
}
}
/**
* Helper method for destroying all {@link Destroyable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when destroying
* the {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to destroy (in case them implement the
* {@link Destroyable} interface).
*
* @throws DestroyException in case initialization of at least one
* {@link Destroyable} instance failed.
*/
@SafeVarargs
public static void destroy( ExecutionStrategy aComponentExecutionStrategy, Object... aComponents ) {
destroy( aComponentExecutionStrategy, Arrays.asList( aComponents ) );
}
/**
* Helper method for destroying all {@link Destroyable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}. An {@link ExecutorService} can be
* provided in case some EJB container is to use a managed
* {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when destroying
* the {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws DestroyException in case initialization of at least one
* {@link Destroyable} instance failed.
*/
@SafeVarargs
public static void destroy( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, Object... aComponents ) {
destroy( aComponentExecutionStrategy, aExecutorService, Arrays.asList( aComponents ) );
}
// /////////////////////////////////////////////////////////////////////////
// DECOMPOSE:
// /////////////////////////////////////////////////////////////////////////
/**
* Helper method, decomposes the given {@link Component} in case it is
* {@link Decomposeable}.
*
* @param aComponent The {@link Component} to decompose.
*
* @throws DecomposeException Thrown in case initialization failed.
*/
public static void decompose( Object aComponent ) {
if ( aComponent instanceof Decomposeable ) {
((Decomposeable) aComponent).decompose();
}
}
/**
* Helper method for decomposing all {@link Decomposeable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when decomposing
* the {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to decompose (in case them implement
* the {@link Decomposeable} interface).
*
* @throws DecomposeException in case initialization of at least one
* {@link Decomposeable} instance failed.
*/
public static void decompose( ExecutionStrategy aComponentExecutionStrategy, Collection> aComponents ) {
decompose( aComponentExecutionStrategy, ControlFlowUtility.createDaemonExecutorService(), aComponents );
}
/**
* Helper method for decomposing all {@link Decomposeable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}. An {@link ExecutorService} can be
* provided in case some EJB container is to use a managed
* {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when decomposing
* the {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws DecomposeException in case initialization of at least one
* {@link Decomposeable} instance failed.
*/
public static void decompose( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, Collection> aComponents ) {
try {
execute( aComponentExecutionStrategy, aExecutorService, _decomposeCallableFactoryImpl, aComponents, (Object[]) null );
}
catch ( Exception e ) {
throw new HiddenException( e );
}
}
/**
* Helper method for decomposing all {@link Decomposeable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when decomposing
* the {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to decompose (in case them implement
* the {@link Decomposeable} interface).
*
* @throws DecomposeException in case initialization of at least one
* {@link Decomposeable} instance failed.
*/
@SafeVarargs
public static void decompose( ExecutionStrategy aComponentExecutionStrategy, Object... aComponents ) {
decompose( aComponentExecutionStrategy, Arrays.asList( aComponents ) );
}
/**
* Helper method for decomposing all {@link Decomposeable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}. An {@link ExecutorService} can be
* provided in case some EJB container is to use a managed
* {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when decomposing
* the {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws DecomposeException in case initialization of at least one
* {@link Decomposeable} instance failed.
*/
@SafeVarargs
public static void decompose( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, Object... aComponents ) {
decompose( aComponentExecutionStrategy, aExecutorService, Arrays.asList( aComponents ) );
}
// /////////////////////////////////////////////////////////////////////////
// DISPOSE:
// /////////////////////////////////////////////////////////////////////////
/**
* Helper method, disposes the given {@link Component} in case it is
* {@link DisposeSupport}.
*
* @param aComponent The {@link Component} to dispose.
*
* @throws DisposeException Thrown in case initialization failed.
*/
public static void dispose( Object aComponent ) {
if ( aComponent instanceof DisposeSupport ) {
((DisposeSupport) aComponent).dispose();
}
}
/**
* Helper method for decomposing all {@link DisposeSupport}
* {@link Component} instances found in the provided {@link Collection}. The
* strategy with which the {@link Component} instances are processed is
* defined with the provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when decomposing
* the {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to dispose (in case them implement the
* {@link DisposeSupport} interface).
*
* @throws DisposeException in case initialization of at least one
* {@link DisposeSupport} instance failed.
*/
public static void dispose( ExecutionStrategy aComponentExecutionStrategy, Collection> aComponents ) {
dispose( aComponentExecutionStrategy, ControlFlowUtility.createDaemonExecutorService(), aComponents );
}
/**
* Helper method for decomposing all {@link DisposeSupport}
* {@link Component} instances found in the provided {@link Collection}. The
* strategy with which the {@link Component} instances are processed is
* defined with the provided {@link ExecutionStrategy}. An
* {@link ExecutorService} can be provided in case some EJB container is to
* use a managed {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when decomposing
* the {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws DisposeException in case initialization of at least one
* {@link DisposeSupport} instance failed.
*/
public static void dispose( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, Collection> aComponents ) {
try {
execute( aComponentExecutionStrategy, aExecutorService, _disposeCallableFactoryImpl, aComponents, (Object[]) null );
}
catch ( Exception e ) {
throw new HiddenException( e );
}
}
/**
* Helper method for decomposing all {@link DisposeSupport}
* {@link Component} instances found in the provided {@link Collection}. The
* strategy with which the {@link Component} instances are processed is
* defined with the provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when decomposing
* the {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to dispose (in case them implement the
* {@link DisposeSupport} interface).
*
* @throws DisposeException in case initialization of at least one
* {@link DisposeSupport} instance failed.
*/
@SafeVarargs
public static void dispose( ExecutionStrategy aComponentExecutionStrategy, Object... aComponents ) {
dispose( aComponentExecutionStrategy, Arrays.asList( aComponents ) );
}
/**
* Helper method for decomposing all {@link DisposeSupport}
* {@link Component} instances found in the provided {@link Collection}. The
* strategy with which the {@link Component} instances are processed is
* defined with the provided {@link ExecutionStrategy}. An
* {@link ExecutorService} can be provided in case some EJB container is to
* use a managed {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when decomposing
* the {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws DisposeException in case initialization of at least one
* {@link DisposeSupport} instance failed.
*/
@SafeVarargs
public static void dispose( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, Object... aComponents ) {
dispose( aComponentExecutionStrategy, aExecutorService, Arrays.asList( aComponents ) );
}
// /////////////////////////////////////////////////////////////////////////
// FLUSH:
// /////////////////////////////////////////////////////////////////////////
/**
* Helper method, flushs the given {@link Component} in case it is
* {@link Flushable}.
*
* @param aComponent The {@link Component} to flush.
*
* @throws OpenException Thrown in case initialization failed.
*/
public static void flush( Object aComponent ) throws OpenException {
if ( aComponent instanceof Flushable ) {
try {
((Flushable) aComponent).flush();
}
catch ( IOException e ) {
throw new OpenException( "Unable to flush component <" + aComponent + ">.", e );
}
}
}
/**
* Helper method for flushing all {@link Flushable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when flushing the
* {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to flush (in case them implement the
* {@link Flushable} interface).
*
* @throws OpenException in case initialization of at least one
* {@link Flushable} instance failed.
*/
public static void flush( ExecutionStrategy aComponentExecutionStrategy, Collection> aComponents ) throws OpenException {
flush( aComponentExecutionStrategy, ControlFlowUtility.createDaemonExecutorService(), aComponents );
}
/**
* Helper method for flushing all {@link Flushable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}. An {@link ExecutorService} can be
* provided in case some EJB container is to use a managed
* {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when flushing the
* {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws OpenException in case initialization of at least one
* {@link Flushable} instance failed.
*/
public static void flush( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, Collection> aComponents ) throws OpenException {
try {
execute( aComponentExecutionStrategy, aExecutorService, _flushCallableFactoryImpl, aComponents, (Object[]) null );
}
catch ( Exception e ) {
throw new OpenException( "Unable to flush component(s).", e );
}
}
/**
* Helper method for flushing all {@link Flushable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when flushing the
* {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to flush (in case them implement the
* {@link Flushable} interface).
*
* @throws OpenException in case initialization of at least one
* {@link Flushable} instance failed.
*/
@SafeVarargs
public static void flush( ExecutionStrategy aComponentExecutionStrategy, Object... aComponents ) throws OpenException {
flush( aComponentExecutionStrategy, Arrays.asList( aComponents ) );
}
/**
* Helper method for flushing all {@link Flushable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}. An {@link ExecutorService} can be
* provided in case some EJB container is to use a managed
* {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when flushing the
* {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws OpenException in case initialization of at least one
* {@link Flushable} instance failed.
*/
@SafeVarargs
public static void flush( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, Object... aComponents ) throws OpenException {
flush( aComponentExecutionStrategy, aExecutorService, Arrays.asList( aComponents ) );
}
// /////////////////////////////////////////////////////////////////////////
// RESET:
// /////////////////////////////////////////////////////////////////////////
/**
* Helper method, resets the given {@link Component} in case it is
* {@link Resetable}.
*
* @param aComponent The {@link Component} to reset.
*
* @throws ResetException Thrown in case initialization failed.
*/
public static void reset( Object aComponent ) {
if ( aComponent instanceof Resetable ) {
((Resetable) aComponent).reset();
}
}
/**
* Helper method for decomposing all {@link Resetable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when decomposing
* the {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to reset (in case them implement the
* {@link Resetable} interface).
*
* @throws ResetException in case initialization of at least one
* {@link Resetable} instance failed.
*/
public static void reset( ExecutionStrategy aComponentExecutionStrategy, Collection> aComponents ) {
reset( aComponentExecutionStrategy, ControlFlowUtility.createDaemonExecutorService(), aComponents );
}
/**
* Helper method for decomposing all {@link Resetable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}. An {@link ExecutorService} can be
* provided in case some EJB container is to use a managed
* {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when decomposing
* the {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws ResetException in case initialization of at least one
* {@link Resetable} instance failed.
*/
public static void reset( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, Collection> aComponents ) {
try {
execute( aComponentExecutionStrategy, aExecutorService, _resetCallableFactoryImpl, aComponents, (Object[]) null );
}
catch ( Exception e ) {
throw new HiddenException( e );
}
}
/**
* Helper method for decomposing all {@link Resetable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when decomposing
* the {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to reset (in case them implement the
* {@link Resetable} interface).
*
* @throws ResetException in case initialization of at least one
* {@link Resetable} instance failed.
*/
@SafeVarargs
public static void reset( ExecutionStrategy aComponentExecutionStrategy, Object... aComponents ) {
reset( aComponentExecutionStrategy, Arrays.asList( aComponents ) );
}
/**
* Helper method for decomposing all {@link Resetable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}. An {@link ExecutorService} can be
* provided in case some EJB container is to use a managed
* {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when decomposing
* the {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws ResetException in case initialization of at least one
* {@link Resetable} instance failed.
*/
@SafeVarargs
public static void reset( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, Object... aComponents ) {
reset( aComponentExecutionStrategy, aExecutorService, Arrays.asList( aComponents ) );
}
// /////////////////////////////////////////////////////////////////////////
// OPEN:
// /////////////////////////////////////////////////////////////////////////
/**
* Helper method, opens the given {@link Component} in case it is
* {@link Openable}.
*
* @param aComponent The {@link Component} to open.
*
* @throws OpenException Thrown in case initialization failed.
*/
public static void open( Object aComponent ) throws OpenException {
if ( aComponent instanceof Openable ) {
((Openable) aComponent).open();
}
}
/**
* Helper method for opening all {@link Openable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when opening the
* {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to open (in case them implement the
* {@link Openable} interface).
*
* @throws OpenException in case initialization of at least one
* {@link Openable} instance failed.
*/
public static void open( ExecutionStrategy aComponentExecutionStrategy, Collection> aComponents ) throws OpenException {
open( aComponentExecutionStrategy, ControlFlowUtility.createDaemonExecutorService(), aComponents );
}
/**
* Helper method for opening all {@link Openable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}. An {@link ExecutorService} can be
* provided in case some EJB container is to use a managed
* {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when opening the
* {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws OpenException in case initialization of at least one
* {@link Openable} instance failed.
*/
public static void open( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, Collection> aComponents ) throws OpenException {
try {
execute( aComponentExecutionStrategy, aExecutorService, _openCallableFactoryImpl, aComponents, (Object[]) null );
}
catch ( OpenException e ) {
throw e;
}
catch ( Exception e ) {
throw new OpenException( e );
}
}
/**
* Helper method for opening all {@link Openable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when opening the
* {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to open (in case them implement the
* {@link Openable} interface).
*
* @throws OpenException in case initialization of at least one
* {@link Openable} instance failed.
*/
@SafeVarargs
public static void open( ExecutionStrategy aComponentExecutionStrategy, Object... aComponents ) throws OpenException {
open( aComponentExecutionStrategy, Arrays.asList( aComponents ) );
}
/**
* Helper method for opening all {@link Openable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}. An {@link ExecutorService} can be
* provided in case some EJB container is to use a managed
* {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when opening the
* {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws OpenException in case initialization of at least one
* {@link Openable} instance failed.
*/
@SafeVarargs
public static void open( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, Object... aComponents ) throws OpenException {
open( aComponentExecutionStrategy, aExecutorService, Arrays.asList( aComponents ) );
}
// /////////////////////////////////////////////////////////////////////////
// CONNECT:
// /////////////////////////////////////////////////////////////////////////
/**
* Helper method, configuring the given {@link Component} in case it is
* {@link ConnectionOpenable}.
*
* @param aComponent The {@link Component} to configure.
*
* @throws OpenException Thrown in case connecting failed.
*/
@SuppressWarnings({
"unchecked", "rawtypes"
})
public static void open( Object aComponent, CON aConnection ) throws OpenException {
if ( aComponent instanceof ConnectionOpenable> ) {
((ConnectionOpenable) aComponent).open( aConnection );
}
}
/**
* Helper method for configuring all {@link ConnectionOpenable}
* {@link Component} instances found in the provided {@link Collection}. The
* strategy with which the {@link Component} instances are processed is
* defined with the provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when connecting
* the {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to connect (in case them implement the
* {@link ConnectionOpenable} interface).
*
* @throws OpenException in case connecting of at least one
* {@link ConnectionOpenable} instance failed.
*/
public static void open( ExecutionStrategy aComponentExecutionStrategy, CON aConnection, Collection> aComponents ) throws OpenException {
open( aComponentExecutionStrategy, ControlFlowUtility.createDaemonExecutorService(), aConnection, aComponents );
}
/**
* Helper method for configuring all {@link ConnectionOpenable}
* {@link Component} instances found in the provided {@link Collection}. The
* strategy with which the {@link Component} instances are processed is
* defined with the provided {@link ExecutionStrategy}. An
* {@link ExecutorService} can be provided in case some EJB container is to
* use a managed {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when connecting
* the {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws OpenException in case connecting of at least one
* {@link ConnectionOpenable} instance failed.
*/
public static void open( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, CON aConnection, Collection> aComponents ) throws OpenException {
try {
execute( aComponentExecutionStrategy, aExecutorService, _connectCallableFactoryImpl, aComponents, aConnection );
}
catch ( OpenException e ) {
throw e;
}
catch ( Exception e ) {
throw new OpenException( e );
}
}
/**
* Helper method for configuring all {@link ConfigureException}
* {@link Component} instances found in the provided {@link Collection}. The
* strategy with which the {@link Component} instances are processed is
* defined with the provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when connecting
* the {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to connect (in case them implement the
* {@link ConfigureException} interface).
*
* @throws OpenException in case connecting of at least one
* {@link ConfigureException} instance failed.
*/
@SafeVarargs
public static void open( ExecutionStrategy aComponentExecutionStrategy, CON aConnection, Object... aComponents ) throws OpenException {
open( aComponentExecutionStrategy, aConnection, Arrays.asList( aComponents ) );
}
/**
* Helper method for configuring all {@link ConfigureException}
* {@link Component} instances found in the provided {@link Collection}. The
* strategy with which the {@link Component} instances are processed is
* defined with the provided {@link ExecutionStrategy}. An
* {@link ExecutorService} can be provided in case some EJB container is to
* use a managed {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when connecting
* the {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws OpenException in case connecting of at least one
* {@link ConfigureException} instance failed.
*/
@SafeVarargs
public static void open( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, CON aConnection, Object... aComponents ) throws OpenException {
open( aComponentExecutionStrategy, aExecutorService, aConnection, Arrays.asList( aComponents ) );
}
// /////////////////////////////////////////////////////////////////////////
// I/O CONNECT:
// /////////////////////////////////////////////////////////////////////////
/**
* Helper method, configuring the given {@link Component} in case it is
* {@link BidirectionalConnectionOpenable}.
*
* @param aComponent The {@link Component} to configure.
*
* @throws OpenException Thrown in case connecting failed.
*/
@SuppressWarnings({
"rawtypes", "unchecked"
})
public static void open( Object aComponent, INPUT aInputConnection, OUTPUT aOutputConnection ) throws OpenException {
if ( aComponent instanceof BidirectionalConnectionOpenable, ?> ) {
((BidirectionalConnectionOpenable) aComponent).open( aInputConnection, aOutputConnection );
}
}
/**
* Helper method for configuring all {@link BidirectionalConnectionOpenable}
* {@link Component} instances found in the provided {@link Collection}. The
* strategy with which the {@link Component} instances are processed is
* defined with the provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when connecting
* the {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to connect (in case them implement the
* {@link BidirectionalConnectionOpenable} interface).
*
* @throws OpenException in case connecting of at least one
* {@link BidirectionalConnectionOpenable} instance failed.
*/
public static void open( ExecutionStrategy aComponentExecutionStrategy, INPUT aInputConnection, OUTPUT aOutputConnection, Collection> aComponents ) throws OpenException {
open( aComponentExecutionStrategy, ControlFlowUtility.createDaemonExecutorService(), aInputConnection, aOutputConnection, aComponents );
}
/**
* Helper method for configuring all {@link BidirectionalConnectionOpenable}
* {@link Component} instances found in the provided {@link Collection}. The
* strategy with which the {@link Component} instances are processed is
* defined with the provided {@link ExecutionStrategy}. An
* {@link ExecutorService} can be provided in case some EJB container is to
* use a managed {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when connecting
* the {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws OpenException in case connecting of at least one
* {@link BidirectionalConnectionOpenable} instance failed.
*/
public static void open( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, INPUT aInputConnection, OUTPUT aOutputConnection, Collection> aComponents ) throws OpenException {
try {
execute( aComponentExecutionStrategy, aExecutorService, _ioConnectCallableFactoryImpl, aComponents, aInputConnection, aOutputConnection );
}
catch ( OpenException e ) {
throw e;
}
catch ( Exception e ) {
throw new OpenException( e );
}
}
/**
* Helper method for configuring all {@link ConfigureException}
* {@link Component} instances found in the provided {@link Collection}. The
* strategy with which the {@link Component} instances are processed is
* defined with the provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when connecting
* the {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to connect (in case them implement the
* {@link ConfigureException} interface).
*
* @throws OpenException in case connecting of at least one
* {@link ConfigureException} instance failed.
*/
@SafeVarargs
public static void open( ExecutionStrategy aComponentExecutionStrategy, INPUT aInputConnection, OUTPUT aOutputConnection, Object... aComponents ) throws OpenException {
open( aComponentExecutionStrategy, aInputConnection, aOutputConnection, Arrays.asList( aComponents ) );
}
/**
* Helper method for configuring all {@link ConfigureException}
* {@link Component} instances found in the provided {@link Collection}. The
* strategy with which the {@link Component} instances are processed is
* defined with the provided {@link ExecutionStrategy}. An
* {@link ExecutorService} can be provided in case some EJB container is to
* use a managed {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when connecting
* the {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws OpenException in case connecting of at least one
* {@link ConfigureException} instance failed.
*/
@SafeVarargs
public static void open( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, INPUT aInputConnection, OUTPUT aOutputConnection, Object... aComponents ) throws OpenException {
open( aComponentExecutionStrategy, aExecutorService, aInputConnection, aOutputConnection, Arrays.asList( aComponents ) );
}
// /////////////////////////////////////////////////////////////////////////
// CLOSE:
// /////////////////////////////////////////////////////////////////////////
/**
* Helper method, closes the given {@link Component} in case it is
* {@link Closable}.
*
* @param aComponent The {@link Component} to close.
*
* @throws CloseException Thrown in case closing or pre-closing (flushing)
* fails.
*/
public static void close( Object aComponent ) throws CloseException {
if ( aComponent instanceof Closable ) {
((Closable) aComponent).close();
}
}
/**
* Helper method for decomposing all {@link Closable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when decomposing
* the {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to close (in case them implement the
* {@link Closable} interface).
*
* @throws CloseException Thrown in case closing or pre-closing (flushing)
* of at least one {@link Closable} instance failed.
*/
public static void close( ExecutionStrategy aComponentExecutionStrategy, Collection> aComponents ) {
close( aComponentExecutionStrategy, ControlFlowUtility.createDaemonExecutorService(), aComponents );
}
/**
* Helper method for decomposing all {@link Closable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}. An {@link ExecutorService} can be
* provided in case some EJB container is to use a managed
* {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when decomposing
* the {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws CloseException Thrown in case closing or pre-closing (flushing)
* fails.
*/
public static void close( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, Collection> aComponents ) {
try {
execute( aComponentExecutionStrategy, aExecutorService, _closeCallableFactoryImpl, aComponents, (Object[]) null );
}
catch ( Exception e ) {
throw new HiddenException( e );
}
}
/**
* Helper method for decomposing all {@link Closable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}.
*
* @param aComponentExecutionStrategy The strategy to use when decomposing
* the {@link Component} instances.
*
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to close (in case them implement the
* {@link Closable} interface).
*
* @throws CloseException Thrown in case closing or pre-closing (flushing)
* of at least one {@link Closable} instance failed.
*/
@SafeVarargs
public static void close( ExecutionStrategy aComponentExecutionStrategy, Object... aComponents ) {
close( aComponentExecutionStrategy, Arrays.asList( aComponents ) );
}
/**
* Helper method for decomposing all {@link Closable} {@link Component}
* instances found in the provided {@link Collection}. The strategy with
* which the {@link Component} instances are processed is defined with the
* provided {@link ExecutionStrategy}. An {@link ExecutorService} can be
* provided in case some EJB container is to use a managed
* {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when decomposing
* the {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
*
* @throws CloseException Thrown in case closing or pre-closing (flushing)
* of at least one {@link Closable} instance failed.
*/
@SafeVarargs
public static void close( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, Object... aComponents ) {
close( aComponentExecutionStrategy, aExecutorService, Arrays.asList( aComponents ) );
}
// /////////////////////////////////////////////////////////////////////////
// HELPER:
// /////////////////////////////////////////////////////////////////////////
/**
* Helper method for initializing all {@link Initializable}
* {@link Component} instances found in the provided {@link Collection}. The
* strategy with which the {@link Component} instances are processed is
* defined with the provided {@link ExecutionStrategy}. An
* {@link ExecutorService} can be provided in case some EJB container is to
* use a managed {@link ExecutorService} provided by an EJB server.
*
* @param aComponentExecutionStrategy The strategy to use when initializing
* the {@link Component} instances.
* @param aExecutorService The {@link ExecutorService} to use when creating
* threads, required in case an {@link ExecutionStrategy#PARALLEL} or
* {@link ExecutionStrategy#JOIN} is used.
* @param aComponents The {@link Collection} containing the
* {@link Component} instances to process (in case them implement the
* required interface).
* @param aArguments An additional argument such as the connection CON or
* the context CTX required in special cases.
*
* @throws Exception in case processing of at least one {@link Component}
* instance failed.
*/
@SuppressWarnings("unchecked")
public static void execute( ExecutionStrategy aComponentExecutionStrategy, ExecutorService aExecutorService, CallableFactory aCallableFactory, Collection> aComponents, Object... aArguments ) throws Exception {
// SEQUENTIAL:
if ( aComponentExecutionStrategy == ExecutionStrategy.SEQUENTIAL ) {
for ( Object e : aComponents ) {
if ( aCallableFactory.getType().isAssignableFrom( e.getClass() ) ) {
Callable theCallable = aCallableFactory.toCallable( (T) e, aArguments );
theCallable.call();
}
}
}
// CONCURRENT:
else if ( aComponentExecutionStrategy == ExecutionStrategy.PARALLEL || aComponentExecutionStrategy == ExecutionStrategy.JOIN ) {
Set> theCallables = new HashSet>();
for ( Object e : aComponents ) {
if ( aCallableFactory.getType().isAssignableFrom( e.getClass() ) ) {
Callable theCallable = aCallableFactory.toCallable( (T) e, aArguments );
theCallables.add( theCallable );
}
}
// PARALLEL:
if ( aComponentExecutionStrategy == ExecutionStrategy.PARALLEL ) {
try {
aExecutorService.invokeAll( theCallables );
}
catch ( InterruptedException ignored ) {}
}
// JOIN:
else if ( aComponentExecutionStrategy == ExecutionStrategy.JOIN ) {
Set> theFutures = new HashSet>();
for ( Callable> eCallable : theCallables ) {
theFutures.add( aExecutorService.submit( eCallable ) );
}
ControlFlowUtility.waitForFutures( theFutures );
}
}
}
// /////////////////////////////////////////////////////////////////////////
// INNER INTERFACES:
// /////////////////////////////////////////////////////////////////////////
/**
* A factory passed to to the
* {@link ComponentUtility#execute(ExecutionStrategy, ExecutorService, Class, Collection)}
* method for generating {@link Callable} instances of dedicated types as of
* the context where the
* {@link ComponentUtility#execute(ExecutionStrategy, ExecutorService, Class, Collection)}
* is invoked.
*
* @param The type of the {@link Component} supported.
*/
private static interface CallableFactory {
/**
* Creates a {@link Callable} from the given {@link Component}.
*
* @param aComponent The {@link Component} for which to create a
* {@link Callable}.
*
* @param aArguments Some {@link Callable} implementations require an
* additional argument such as the context CTX or the connection
* CON for configuration.
*
* @return A {@link Callable} created from the provided argument.
*/
Callable toCallable( T aComponent, Object... aArguments );
/**
* Returns the type of the {@link Component} to be processed.
*
* @return The type of the {@link Component}.
*/
Class getType();
}
// /////////////////////////////////////////////////////////////////////////
// INNER CLASSES:
// /////////////////////////////////////////////////////////////////////////
/**
* Implementation of a INITIALIZE daemon.
*/
private static class InitializeCallableImpl implements Callable {
// /////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////
private Initializable _component;
// /////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////
/**
* Encapsulates the given component in a daemon.
*
* @param aComponent The component to be encapsulated.
*/
private InitializeCallableImpl( Initializable aComponent ) {
_component = aComponent;
}
// /////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////
@Override
public Void call() throws Exception {
_component.initialize();
return null;
}
}
/**
* Implementation of a CONFIGURE daemon.
*/
private static class ConfigureCallableImpl implements Callable {
// /////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////
private Configurable _component;
private CTX _context;
// /////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////
/**
* Encapsulates the given component in a daemon.
*
* @param aComponent The component to be encapsulated.
*/
private ConfigureCallableImpl( Configurable aComponent, CTX aContext ) {
_component = aComponent;
_context = aContext;
}
// /////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////
@Override
public Void call() throws Exception {
_component.initialize( _context );
return null;
}
}
/**
* Implementation of a START daemon.
*/
private static class StartCallableImpl implements Callable {
// /////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////
private Startable _component;
// /////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////
/**
* Encapsulates the given component in a daemon.
*
* @param aComponent The component to be encapsulated.
*/
private StartCallableImpl( Startable aComponent ) {
_component = aComponent;
}
// /////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////
@Override
public Void call() throws Exception {
_component.start();
return null;
}
}
/**
* Implementation of a PAUSE daemon.
*/
private static class PauseCallableImpl implements Callable {
// /////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////
private Pausable _component;
// /////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////
/**
* Encapsulates the given component in a daemon.
*
* @param aComponent The component to be encapsulated.
*/
private PauseCallableImpl( Pausable aComponent ) {
_component = aComponent;
}
// /////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////
@Override
public Void call() throws Exception {
_component.pause();
return null;
}
}
/**
* Implementation of a RESUME daemon.
*/
private static class ResumeCallableImpl implements Callable {
// /////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////
private Resumable _component;
// /////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////
/**
* Encapsulates the given component in a daemon.
*
* @param aComponent The component to be encapsulated.
*/
private ResumeCallableImpl( Resumable aComponent ) {
_component = aComponent;
}
// /////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////
@Override
public Void call() throws Exception {
_component.resume();
return null;
}
}
/**
* Implementation of a STOP daemon.
*/
private static class StopCallableImpl implements Callable {
// /////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////
private Stoppable _component;
// /////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////
/**
* Encapsulates the given component in a daemon.
*
* @param aComponent The component to be encapsulated.
*/
private StopCallableImpl( Stoppable aComponent ) {
_component = aComponent;
}
// /////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////
@Override
public Void call() throws Exception {
_component.stop();
return null;
}
}
/**
* Implementation of a DESTROY daemon.
*/
private static class DestroyCallableImpl implements Callable {
// /////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////
private Destroyable _component;
// /////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////
/**
* Encapsulates the given component in a daemon.
*
* @param aComponent The component to be encapsulated.
*/
private DestroyCallableImpl( Destroyable aComponent ) {
_component = aComponent;
}
// /////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////
@Override
public Void call() throws Exception {
_component.destroy();
return null;
}
}
/**
* Implementation of a DECOMPOSE daemon.
*/
private static class DecomposeCallableImpl implements Callable {
// /////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////
private Decomposeable _component;
// /////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////
/**
* Encapsulates the given component in a daemon.
*
* @param aComponent The component to be encapsulated.
*/
private DecomposeCallableImpl( Decomposeable aComponent ) {
_component = aComponent;
}
// /////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////
@Override
public Void call() throws Exception {
_component.decompose();
return null;
}
}
/**
* Implementation of a DISPOSE daemon.
*/
private static class DisposeCallableImpl implements Callable {
// /////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////
private DisposeSupport _component;
// /////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////
/**
* Encapsulates the given component in a daemon.
*
* @param aComponent The component to be encapsulated.
*/
private DisposeCallableImpl( DisposeSupport aComponent ) {
_component = aComponent;
}
// /////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////
@Override
public Void call() throws Exception {
_component.dispose();
return null;
}
}
/**
* Implementation of a FLUSH daemon.
*/
private static class FlushCallableImpl implements Callable {
// /////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////
private Flushable _component;
// /////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////
/**
* Encapsulates the given component in a daemon.
*
* @param aComponent The component to be encapsulated.
*/
private FlushCallableImpl( Flushable aComponent ) {
_component = aComponent;
}
// /////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////
@Override
public Void call() throws Exception {
_component.flush();
return null;
}
}
/**
* Implementation of a RESET daemon.
*/
private static class ResetCallableImpl implements Callable {
// /////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////
private Resetable _component;
// /////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////
/**
* Encapsulates the given component in a daemon.
*
* @param aComponent The component to be encapsulated.
*/
private ResetCallableImpl( Resetable aComponent ) {
_component = aComponent;
}
// /////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////
@Override
public Void call() throws Exception {
_component.reset();
return null;
}
}
/**
* Implementation of a OPEN daemon.
*/
private static class OpenCallableImpl implements Callable {
// /////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////
private Openable _component;
// /////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////
/**
* Encapsulates the given component in a daemon.
*
* @param aComponent The component to be encapsulated.
*/
private OpenCallableImpl( Openable aComponent ) {
_component = aComponent;
}
// /////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////
@Override
public Void call() throws Exception {
_component.open();
return null;
}
}
/**
* Implementation of a CONNECT daemon.
*/
private static class ConnectCallableImpl implements Callable {
// /////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////
private ConnectionOpenable _component;
private CON _connection;
// /////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////
/**
* Encapsulates the given component in a daemon.
*
* @param aComponent The component to be encapsulated.
*/
private ConnectCallableImpl( ConnectionOpenable aComponent, CON aConnection ) {
_component = aComponent;
_connection = aConnection;
}
// /////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////
@Override
public Void call() throws Exception {
_component.open( _connection );
return null;
}
}
/**
* Implementation of a I/O CONNECT daemon.
*/
private static class IoConnectCallableImpl implements Callable {
// /////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////
private BidirectionalConnectionOpenable _component;
private INPUT _inputConnection;
private OUTPUT _outputConnection;
// /////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////
/**
* Encapsulates the given component in a daemon.
*
* @param aComponent The component to be encapsulated.
*/
private IoConnectCallableImpl( BidirectionalConnectionOpenable aComponent, INPUT aInputConnection, OUTPUT aOutputConnection ) {
_component = aComponent;
_inputConnection = aInputConnection;
_outputConnection = aOutputConnection;
}
// /////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////
@Override
public Void call() throws Exception {
_component.open( _inputConnection, _outputConnection );
return null;
}
}
/**
* Implementation of a CLOSE daemon.
*/
private static class CloseCallableImpl implements Callable {
// /////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////
private Closable _component;
// /////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////
/**
* Encapsulates the given component in a daemon.
*
* @param aComponent The component to be encapsulated.
*/
private CloseCallableImpl( Closable aComponent ) {
_component = aComponent;
}
// /////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////
@Override
public Void call() throws Exception {
_component.close();
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy