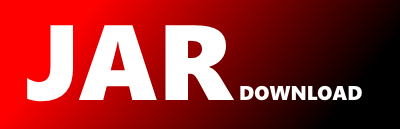
org.refcodes.component.ConfigurableHandle Maven / Gradle / Ivy
package org.refcodes.component;
import org.refcodes.component.Configurable.ConfigureAutomaton;
/**
* The {@link ConfigurableHandle} interface defines those methods related to the
* handle based initialize/configure life-cycle.
*
* The handle reference requires the {@link Configurable} interface to be
* implemented.
*
* @param The type of the handle.
*
* @param The context used to initialize the referenced instance.
*/
public interface ConfigurableHandle {
/**
* Determines whether the handle reference is configurable by implementing
* the {@link Configurable} interface.
*
* @param aHandle The handle to test whether the reference provides the
* according functionality.
*
* @return True in case the reference provides the according functionality.
*
* @throws UnknownHandleRuntimeException in case the handle is unknown.
*/
boolean hasConfigurable( H aHandle ) throws UnknownHandleRuntimeException;
/**
* Initialize/configure the component identified by the given handle.
*
* @param aContext The context to be passed to the implementing instance.
*
* @param aHandle The handle identifying the component.
*
* @throws ConfigureException in case initializing fails.
*
* @throws UnsupportedHandleOperationRuntimeException in case the reference
* of the handle does not support the requested operation.
*
* @throws UnknownHandleRuntimeException in case the given handle is
* unknown.
*
* @throws IllegaleHandleStateChangeRuntimeException Thrown in case a state
* change is not possible due to the current state the referenced
* component is in.
*/
void initialize( H aHandle, CTX aContext ) throws ConfigureException, UnknownHandleRuntimeException, UnsupportedHandleOperationRuntimeException, IllegaleHandleStateChangeRuntimeException;
/**
* The {@link ConfigureAutomatonHandle} interface defines those methods
* related to the handle based initialize/configure life-cycle.
*
* The handle reference requires the {@link ConfigureAutomaton} interface to
* be implemented.
*
* @param The type of the handle.
*
* @param the context used to initialize the referenced instance.
*/
public interface ConfigureAutomatonHandle extends ConfigurableHandle, InitializedHandle {
/**
* Determines whether the handle reference is configurable by
* implementing the {@link ConfigureAutomaton} interface.
*
* @param aHandle The handle to test whether the reference provides the
* according functionality.
*
* @return True in case the reference provides the according
* functionality.
*
* @throws UnknownHandleRuntimeException in case the handle is unknown.
*/
boolean hasConfigureAutomaton( H aHandle ) throws UnknownHandleRuntimeException;
/**
* Determines whether the component identified by the given handle may
* get initialized/configured.
*
* @param aHandle The handle identifying the component.
*
* @param aContext The context to be passed to the implementing
* instance.
*
* @return True if {@link #initialize(Object, Object)} is possible.
*
* @throws UnsupportedHandleOperationRuntimeException in case the
* reference of the handle does not support the requested
* operation.
*
* @throws UnknownHandleRuntimeException in case the given handle is
* unknown.
*/
boolean isInitalizable( H aHandle, CTX aContext ) throws UnknownHandleRuntimeException, UnsupportedHandleOperationRuntimeException;
}
}