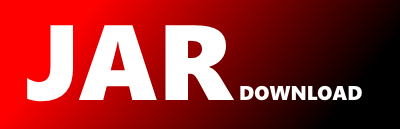
org.refcodes.component.ConfigurableLifeCycleComponent Maven / Gradle / Ivy
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// =============================================================================
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// =============================================================================
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// together with the GPL linking exception applied; as being applied by the GNU
// Classpath ("http://www.gnu.org/software/classpath/license.html")
// =============================================================================
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// =============================================================================
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.component;
import org.refcodes.component.LifeCycleComponent.LifeCycleAutomaton;
import org.refcodes.component.LifeCycleComponentHandle.LifeCycleAutomatonHandle;
/**
* A component implementing the {@link ConfigurableLifeCycleComponent} interface
* supports a life-cycle. I.e. such a component may be instructed from the
* outside to run through several stages from getting started till being
* destroyed. The valid state changes are mainly as follows:
*
* "initialize" - "start" - "pause" - "resume" - "stop" - "destroy"
*
*
* For example: "initialize" - "start" - "pause" - "resume" - "pause" - "resume"
* - "stop" - "start" - "pause" - "resume" - "stop" - "destroy"
*
* The {@link LifeCycleAutomatonHandle} is a component managing various
* {@link ConfigurableLifeCycleComponent}s each identified by a dedicated
* handle. Operations on the {@link ConfigurableLifeCycleComponent} are invoked
* by this {@link LifeCycleAutomatonHandle} with a handle identifying the
* according {@link ConfigurableLifeCycleComponent}.
*
* The {@link ConfigurableLifeCycleComponent} contains the business-logic where
* as the {@link LifeCycleAutomatonHandle} provides the frame for managing this
* business-logic. The {@link LifeCycleAutomaton} takes care of the correct
* life-cycle applied on a {@link ConfigurableLifeCycleComponent}.
*
* @param the context used to initialize the implementing instance.
*/
public interface ConfigurableLifeCycleComponent extends Configurable, Startable, Pausable, Resumable, Stoppable, Destroyable {
/**
* A system implementing the {@link ConfigurableLifeCycleAutomaton}
* interface supports managing {@link ConfigurableLifeCycleComponent}
* instances and takes care that the open/close statuses are invoked in the
* correct order by throwing according exceptions in case the
* open/close-cycle is invoked in the wrong order.
*
* A {@link ConfigurableLifeCycleAutomaton} may be used to wrap a
* {@link ConfigurableLifeCycleComponent} by a
* {@link HandleConfigurableAutomaton} for managing
* {@link ConfigurableLifeCycleAutomaton} instances.
*
* The {@link ConfigurableLifeCycleComponent} contains the business-logic
* where as the {@link HandleConfigurableAutomaton} provides the frame for
* managing this business-logic. The {@link ConfigurableLifeCycleAutomaton}
* takes care of the correct open/close-cycle applied on a
* {@link ConfigurableLifeCycleComponent}.
*
* @param the context used to initialize the implementing instance.
*/
public interface ConfigurableLifeCycleAutomaton extends ConfigurableLifeCycleComponent, ConfigureAutomaton, StartAutomaton, PauseAutomaton, ResumeAutomaton, StopAutomaton, DestroyAutomaton, LifeCycleStatusAccessor {}
}