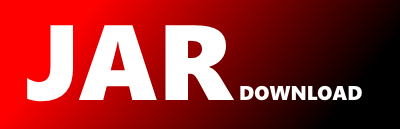
org.refcodes.component.ConnectionOpenable Maven / Gradle / Ivy
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// =============================================================================
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// =============================================================================
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// together with the GPL linking exception applied; as being applied by the GNU
// Classpath ("http://www.gnu.org/software/classpath/license.html")
// =============================================================================
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// =============================================================================
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.component;
import org.refcodes.component.Configurable.ConfigureAutomaton;
/**
* This mixin might be implemented by a component in order to provide opening
* connection(s) facilities. The semantics of this interface is very similar to
* that of the {@link Configurable} interface. To clarify the context regarding
* connections, the {@link ConnectionOpenable} interface has been introduced.
*
* In case a no connection is to be provided to the {@link #open(Object)} method
* (as it may have been passed via the constructor), you may use the
* {@link Openable} interface with its {@link Openable#open()} method, which
* does not require any arguments specifying a connection.
*
* @param The type of the connection to be used.
*/
public interface ConnectionOpenable {
/**
* Opens the component with the given connection, the component opens a
* connection with the given connection.
*
* @param aConnection The connection used for opening the connection.
*
* @throws OpenException Thrown in case opening or accessing an open line
* (connection, junction, link) caused problems.
*/
void open( CON aConnection ) throws OpenException;
/**
* The {@link ConnectionOpenAutomaton} interface defines those methods
* related to the opening of connection(s) life-cycle. The semantics of this
* interface is very similar to that of the {@link ConfigureAutomaton}
* interface. To clarify the context regarding connections, the
* {@link ConnectionOpenAutomaton} interface has been introduced.
*
* @param The type of the connection to be used.
*/
public interface ConnectionOpenAutomaton extends ConnectionOpenable, OpenedAccessor {
/**
* Determines whether the given connection may get opened, if true then
* component may open a connection with the given connection via the
* {@link #open(Object)} method. Usually no physical connection is
* established; usually criteria describing the provided connection are
* evaluated; for example the connection is tested against a black list,
* a white list or against well-formedness or whether the specified
* protocols are supported (in case of a connection being a String URL
* beginning with "http://", "ftp://" or similar).
* ---------------------------------------------------------------------
* CAUTION: Even in case true is returned, the actual opening of a
* connection may fail (e.g. due to network failure or authentication
* issues).
* ---------------------------------------------------------------------
*
* @param aConnection The connection for which to determine whether it
* can be used to open a connection.
*
* @return True if {@link #open(Object)} is theoretically possible.
*/
boolean isOpenable( CON aConnection );
}
}