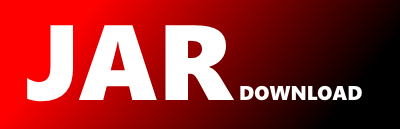
org.refcodes.component.DestroyableHandle Maven / Gradle / Ivy
package org.refcodes.component;
import org.refcodes.component.Destroyable.DestroyAutomaton;
/**
* The {@link DestroyableHandle} interface defines those methods related to the
* handle based destroy life-cycle.
*
* The handle reference requires the {@link Destroyable} interface to be
* implemented.
*
* @param The type of the handle.
*/
public interface DestroyableHandle {
/**
* Determines whether the handle reference is destroyable by implementing
* the {@link Destroyable} interface.
*
* @param aHandle The handle to test whether the reference provides the
* according functionality.
*
* @return True in case the reference provides the according functionality.
*
* @throws UnknownHandleRuntimeException in case the handle is unknown.
*/
boolean hasDestroyable( H aHandle ) throws UnknownHandleRuntimeException;
/**
* Destroys the component identified by the given handle.
*
* @param aHandle The handle identifying the component.
*
* @throws UnsupportedHandleOperationRuntimeException in case the reference
* of the handle does not support the requested operation.
*
* @throws UnknownHandleRuntimeException in case the given handle is
* unknown.
*
* @throws IllegaleHandleStateChangeRuntimeException Thrown in case a state
* change is not possible due to the current state the referenced
* component is in.
*/
void destroy( H aHandle ) throws UnknownHandleRuntimeException, UnsupportedHandleOperationRuntimeException, IllegaleHandleStateChangeRuntimeException;
/**
* The {@link DestroyAutomatonHandle} interface defines those methods
* related to the handle based destroy life-cycle.
*
* The handle reference requires the {@link DestroyAutomaton} interface to
* be implemented.
*
* @param The type of the handle.
*/
public interface DestroyAutomatonHandle extends DestroyableHandle {
/**
* Determines whether the handle reference is destroyable by
* implementing the {@link DestroyAutomaton} interface.
*
* @param aHandle The handle to test whether the reference provides the
* according functionality.
*
* @return True in case the reference provides the according
* functionality.
*
* @throws UnknownHandleRuntimeException in case the handle is unknown.
*/
boolean hasDestroyAutomaton( H aHandle ) throws UnknownHandleRuntimeException;
/**
* Determines whether the component identified by the given handle may
* get destroyed.
*
* @param aHandle The handle identifying the component.
*
* @return True if {@link #destroy(Object)} is possible.
*
* @throws UnsupportedHandleOperationRuntimeException in case the
* reference of the handle does not support the requested
* operation.
* @throws UnknownHandleRuntimeException in case the given handle is
* unknown.
*/
boolean isDestroyable( H aHandle ) throws UnknownHandleRuntimeException, UnsupportedHandleOperationRuntimeException;
/**
* Determines whether the component identified by the given handle is
* destroyed.
*
* @param aHandle The handle identifying the component.
*
* @return True in case of being destroyed, else false.
*
* @throws UnsupportedHandleOperationRuntimeException in case the
* reference of the handle does not support the requested
* operation.
* @throws UnknownHandleRuntimeException in case the given handle is
* unknown.
*/
boolean isDestroyed( H aHandle ) throws UnknownHandleRuntimeException, UnsupportedHandleOperationRuntimeException;
}
}