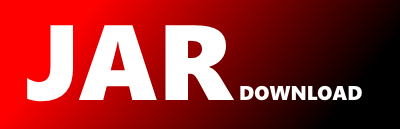
org.refcodes.component.ResumableHandle Maven / Gradle / Ivy
package org.refcodes.component;
import org.refcodes.component.Resumable.ResumeAutomaton;
/**
* The {@link ResumableHandle} interface defines those methods related to the
* handle based resume life-cycle.
*
* The handle reference requires the {@link Resumable} interface to be
* implemented.
*
* @param The type of the handle.
*/
public interface ResumableHandle {
/**
* Determines whether the handle reference is resumable by implementing the
* {@link Resumable} interface.
*
* @param aHandle The handle to test whether the reference provides the
* according functionality.
*
* @return True in case the reference provides the according functionality.
*
* @throws UnknownHandleRuntimeException in case the handle is unknown.
*/
boolean hasResumable( H aHandle ) throws UnknownHandleRuntimeException;
/**
* Resumes the component identified by the given handle.
*
* @param aHandle The handle identifying the component.
*
* @throws ResumeException in case resuming fails.
*
* @throws UnsupportedHandleOperationRuntimeException in case the reference
* of the handle does not support the requested operation.
*
* @throws UnknownHandleRuntimeException in case the given handle is
* unknown.
*
* @throws IllegaleHandleStateChangeRuntimeException Thrown in case a state
* change is not possible due to the current state the referenced
* component is in.
*/
void resume( H aHandle ) throws ResumeException, UnknownHandleRuntimeException, UnsupportedHandleOperationRuntimeException, IllegaleHandleStateChangeRuntimeException;
/**
* The {@link ResumeAutomatonHandle} interface defines those methods related
* to the handle based resume life-cycle.
*
* The handle reference requires the {@link ResumeAutomaton} interface to be
* implemented.
*
* @param The type of the handle.
*/
public interface ResumeAutomatonHandle extends RunningHandle, ResumableHandle {
/**
* Determines whether the handle reference is resumable by implementing
* the {@link ResumeAutomaton} interface.
*
* @param aHandle The handle to test whether the reference provides the
* according functionality.
*
* @return True in case the reference provides the according
* functionality.
*
* @throws UnknownHandleRuntimeException in case the handle is unknown.
*/
boolean hasResumeAutomaton( H aHandle ) throws UnknownHandleRuntimeException;
/**
* Determines whether the component identified by the given handle may
* get resumed.
*
* @param aHandle The handle identifying the component.
*
* @return True if {@link #resume(Object)} is possible.
*
* @throws UnsupportedHandleOperationRuntimeException in case the
* reference of the handle does not support the requested
* operation.
* @throws UnknownHandleRuntimeException in case the given handle is
* unknown.
*/
boolean isResumable( H aHandle ) throws UnknownHandleRuntimeException, UnsupportedHandleOperationRuntimeException;
}
}