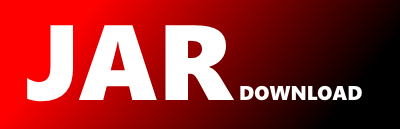
org.refcodes.component.impls.ConfigurableLifeCycleAutomatonImpl Maven / Gradle / Ivy
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// =============================================================================
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// =============================================================================
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// together with the GPL linking exception applied; as being applied by the GNU
// Classpath ("http://www.gnu.org/software/classpath/license.html")
// =============================================================================
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// =============================================================================
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.component.impls;
import org.refcodes.component.ConfigurableLifeCycleComponent;
import org.refcodes.component.ConfigurableLifeCycleComponent.ConfigurableLifeCycleAutomaton;
import org.refcodes.component.ConfigureException;
import org.refcodes.component.LifeCycleComponent;
import org.refcodes.component.LifeCycleComponent.LifeCycleAutomaton;
import org.refcodes.component.LifeCycleStatus;
import org.refcodes.component.PauseException;
import org.refcodes.component.ResumeException;
import org.refcodes.component.StartException;
import org.refcodes.component.StopException;
/**
* This class implements a {@link ConfigurableLifeCycleAutomaton}.
*
* @param the context used to initialize the implementing instance.
*/
public class ConfigurableLifeCycleAutomatonImpl implements ConfigurableLifeCycleAutomaton {
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private LifeCycleStatus _lifeCycleStatus = LifeCycleStatus.NONE;
private ConfigurableLifeCycleComponent _configurableLifeCycleComponent = null;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Empty constructor, such {@link LifeCycleAutomaton} cannot do much more
* than decline the various {@link LifeCycleStatus} states for you.
*/
public ConfigurableLifeCycleAutomatonImpl() {}
/**
* This constructor uses a {@link LifeCycleStatus} for wrapping it inside
* the {@link ConfigurableLifeCycleAutomatonImpl}, making sure of obeying
* and guarding the correct {@link LifeCycleStatus}'s order of
* {@link LifeCycleStatus} states for you.
*
* @param aLifeConfigurableCycleComponent The component to be guarded
* regarding the correct declination of the {@link LifeCycleStatus}
* states.
*/
public ConfigurableLifeCycleAutomatonImpl( ConfigurableLifeCycleComponent aLifeConfigurableCycleComponent ) {
_configurableLifeCycleComponent = aLifeConfigurableCycleComponent;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
@Override
public synchronized LifeCycleStatus getLifeCycleStatus() {
return _lifeCycleStatus;
}
// /////////////////////////////////////////////////////////////////////////
// CONVENIANCE METHODS:
// /////////////////////////////////////////////////////////////////////////
@Override
public synchronized void initialize( CTX aContext ) throws ConfigureException {
if ( !isInitalizable( aContext ) ) {
throw new ConfigureException( aContext, "Cannot initialize (configure) as the component is in status <" + _lifeCycleStatus + "> which is not the appropriate status for initializing (configuring)." );
}
if ( _configurableLifeCycleComponent != null ) {
_configurableLifeCycleComponent.initialize( aContext );
}
_lifeCycleStatus = LifeCycleStatus.INITIALIZED;
}
@SuppressWarnings("unchecked")
@Override
public synchronized boolean isInitalizable( CTX aContext ) {
boolean isConfigurable = (_lifeCycleStatus == LifeCycleStatus.NONE) || (isDestroyed());
if ( isConfigurable && _configurableLifeCycleComponent != null && _configurableLifeCycleComponent instanceof ConfigureAutomaton> ) {
ConfigureAutomaton theConfigureAutomaton = (ConfigureAutomaton) _configurableLifeCycleComponent;
return theConfigureAutomaton.isInitalizable( aContext );
}
return isConfigurable;
}
@Override
public synchronized boolean isInitialized() {
return (_lifeCycleStatus == LifeCycleStatus.INITIALIZED);
}
@Override
public synchronized boolean isStartable() {
return (isInitialized() || isStopped());
}
@Override
public synchronized void start() throws StartException {
if ( !isStartable() ) {
throw new StartException( "Cannot start as the component is in status <" + _lifeCycleStatus + "> which is not the appropriate status for starting." );
}
if ( _configurableLifeCycleComponent != null ) {
_configurableLifeCycleComponent.start();
}
_lifeCycleStatus = LifeCycleStatus.STARTED;
}
@Override
public synchronized boolean isRunning() {
return (_lifeCycleStatus == LifeCycleStatus.STARTED);
}
@Override
public synchronized boolean isPausable() {
return isRunning();
}
@Override
public synchronized void pause() throws PauseException {
if ( !isPausable() ) {
throw new PauseException( "Cannot pause as the component is in status <" + _lifeCycleStatus + "> which is not the appropriate status for pausing." );
}
if ( _configurableLifeCycleComponent != null ) {
_configurableLifeCycleComponent.pause();
}
_lifeCycleStatus = LifeCycleStatus.PAUSED;
}
@Override
public synchronized boolean isPaused() {
return (_lifeCycleStatus == LifeCycleStatus.PAUSED);
}
@Override
public synchronized boolean isResumable() {
return isPaused();
}
@Override
public synchronized void resume() throws ResumeException {
if ( !isResumable() ) {
throw new ResumeException( "Cannot resume as the component is in status <" + _lifeCycleStatus + "> which is not the appropriate status for resuming." );
}
if ( _configurableLifeCycleComponent != null ) {
_configurableLifeCycleComponent.resume();
}
_lifeCycleStatus = LifeCycleStatus.STARTED;
}
@Override
public synchronized boolean isStoppable() {
return isRunning() || isPaused();
}
@Override
public synchronized void stop() throws StopException {
if ( !isStoppable() ) {
throw new StopException( "Cannot stop as the component is in status <" + _lifeCycleStatus + "> which is not the appropriate status for stopping." );
}
if ( _configurableLifeCycleComponent != null ) {
_configurableLifeCycleComponent.stop();
}
_lifeCycleStatus = LifeCycleStatus.STOPPED;
}
@Override
public synchronized boolean isStopped() {
return (_lifeCycleStatus == LifeCycleStatus.STOPPED);
}
@Override
public synchronized boolean isDestroyable() {
return isStopped();
}
@Override
public synchronized void destroy() {
if ( !isDestroyable() ) {
return;
}
if ( _configurableLifeCycleComponent != null ) {
_configurableLifeCycleComponent.destroy();
}
_lifeCycleStatus = LifeCycleStatus.DESTROYED;
}
@Override
public synchronized boolean isDestroyed() {
return (_lifeCycleStatus == LifeCycleStatus.DESTROYED);
}
// /////////////////////////////////////////////////////////////////////////
// HOOKS:
// /////////////////////////////////////////////////////////////////////////
/**
* Provides access to the {@link LifeCycleComponent} instance.
*
* @return The {@link LifeCycleComponent} instance being set.
*/
protected ConfigurableLifeCycleComponent getLifeCycleComponent() {
return _configurableLifeCycleComponent;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy