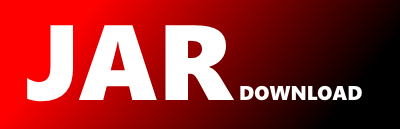
org.refcodes.component.impls.DeviceAutomatonImpl Maven / Gradle / Ivy
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// =============================================================================
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// =============================================================================
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// together with the GPL linking exception applied; as being applied by the GNU
// Classpath ("http://www.gnu.org/software/classpath/license.html")
// =============================================================================
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// =============================================================================
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.component.impls;
import org.refcodes.component.ConnectionStatus;
import org.refcodes.component.DeviceComponent;
import org.refcodes.component.DeviceComponent.DeviceAutomaton;
import org.refcodes.component.LifeCycleComponent.LifeCycleAutomaton;
import org.refcodes.component.LifeCycleStatus;
import org.refcodes.component.OpenException;
/**
* This class implements a {@link LifeCycleAutomaton}.
*/
public class DeviceAutomatonImpl implements DeviceAutomaton {
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private ConnectionStatus _connectionStatus = ConnectionStatus.NONE;
protected DeviceComponent _connectionComponent = null;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Empty constructor, such {@link LifeCycleAutomaton} cannot do much more
* than decline the various {@link LifeCycleStatus} states for you.
*/
public DeviceAutomatonImpl() {}
/**
* This constructor uses a {@link LifeCycleStatus} for wrapping it inside
* the {@link DeviceAutomatonImpl}, making sure of obeying and guarding the
* correct {@link LifeCycleStatus}'s order of {@link LifeCycleStatus} states
* for you.
*
* @param aConnectionComponent The component to be guarded regarding the
* correct declination of the {@link LifeCycleStatus} states.
*/
public DeviceAutomatonImpl( DeviceComponent aConnectionComponent ) {
_connectionComponent = aConnectionComponent;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
@Override
public synchronized ConnectionStatus getConnectionStatus() {
return _connectionStatus;
}
// /////////////////////////////////////////////////////////////////////////
// CONVENIANCE METHODS:
// /////////////////////////////////////////////////////////////////////////
@Override
public boolean isOpenable() {
return (_connectionStatus == ConnectionStatus.NONE) || (isClosed());
}
@Override
public void open() throws OpenException {
if ( !isOpenable() ) {
throw new OpenException( "Cannot open as the component is in status <" + _connectionStatus + "> which is not the appropriate status for opening." );
}
if ( _connectionComponent != null ) {
_connectionComponent.open();
}
_connectionStatus = ConnectionStatus.OPENED;
}
@Override
public boolean isOpened() {
return (_connectionStatus == ConnectionStatus.OPENED);
}
@Override
public boolean isClosable() {
return (_connectionStatus == ConnectionStatus.OPENED);
}
@Override
public boolean isClosed() {
return (_connectionStatus == ConnectionStatus.CLOSED);
}
@Override
public void close() {
if ( isOpened() ) {
try {
if ( _connectionComponent != null ) {
_connectionComponent.close();
}
}
catch ( Exception e ) {
// LOGGER.warn(
// "While closing the component, an unexpected exception was thrown (close must always work), setting connection status to <"
// + ConnectionStatus.CLOSED + ">.", e );
}
}
_connectionStatus = ConnectionStatus.CLOSED;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy