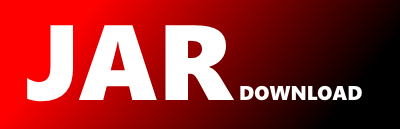
org.refcodes.configuration.ext.obfuscation.ObfuscationResourceProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-configuration-ext-obfuscation Show documentation
Show all versions of refcodes-configuration-ext-obfuscation Show documentation
Artifact for obfuscating chosen properties in a properties file.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.configuration.ext.obfuscation;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.text.ParseException;
import org.refcodes.configuration.ResourceProperties;
import org.refcodes.mixin.DecryptPrefixAccessor;
import org.refcodes.mixin.EncryptPrefixAccessor;
import org.refcodes.structure.Relation;
/**
* The {@link ObfuscationResourceProperties} adds functionality to the
* {@link ResourceProperties} type for encrypting and decrypting individual
* properties.
*
* Properties marked in the resource as "to be decrypted" are decrypted (e.g.
* with a host individual key). Those encrypted properties are decrypted
* "on-the-fly" (in memory only) upon accessing the according property.
* Retrieving a value via {@link #get(Object)} (or the like), which is prefixed
* with "decrypt:" (default), will be decrypted accordingly before passed back
* to the caller. The prefix may be changed by invoking the according
* implementaion's constructor.
*
* (depending on the implementation, the defaults may by changed to individual
* values)
*/
public interface ObfuscationResourceProperties extends ObfuscationProperties, ResourceProperties, EncryptPrefixAccessor, DecryptPrefixAccessor {
// /////////////////////////////////////////////////////////////////////////
// MUTATOR:
// /////////////////////////////////////////////////////////////////////////
/**
* The interface {@link MutableObfuscationResourceProperties} defines
* "dirty" methods allowing to modify ("mutate") the
* {@link ObfuscationResourceProperties}.
*
* In addition to the {@link ObfuscationResourceProperties}, encryption is
* additionally supported: Properties marked in the resource as "to be
* encrypted" by being prefixed with "encrypt:" (default) are encrypted
* (e.g. with a host individual key) when being added and instead are
* prefixed with "decrypt:" (default). Retrieving a value via
* {@link #get(Object)} (or the like), which is prefixed with "decrypt:"
* (default), will be decrypted accordingly before passed back to the
* caller. The prefix may be changed by invoking the according
* implementaion's constructor or the according methods.
*
* (depending on the implementation, the defaults may by changed to
* individual values)
*/
public interface MutableObfuscationResourceProperties extends ObfuscationResourceProperties, MutableResoureProperties {}
// /////////////////////////////////////////////////////////////////////////
// BUILDER:
// /////////////////////////////////////////////////////////////////////////
/**
* The interface {@link ObfuscationResourcePropertiesBuilder} defines
* builder functionality on top of the
* {@link MutableObfuscationResourceProperties}.
*/
public interface ObfuscationResourcePropertiesBuilder extends MutableObfuscationResourceProperties, ResourcePropertiesBuilder, ObfuscationPropertiesBuilder {
// /////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withDecryptPrefix( String aDecryptPrefix ) {
setDecryptPrefix( aDecryptPrefix );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withEncryptPrefix( String aEncryptPrefix ) {
setEncryptPrefix( aEncryptPrefix );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withInsert( Object aObj ) {
insert( aObj );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withInsertBetween( String aToPath, Object aFrom, String aFromPath ) {
insertBetween( aToPath, aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withInsertFrom( Object aFrom, String aFromPath ) {
insertFrom( aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withInsertTo( String aToPath, Object aFrom ) {
insertTo( aToPath, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withLoadFrom( Class> aResourceClass, String aFilePath ) throws IOException, ParseException {
loadFrom( aResourceClass, aFilePath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withLoadFrom( Class> aResourceClass, String aFilePath, char... aDelimiters ) throws IOException, ParseException {
loadFrom( aResourceClass, aFilePath, aDelimiters );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withLoadFrom( File aFile ) throws IOException, ParseException {
loadFrom( aFile );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withLoadFrom( File aFile, char... aDelimiters ) throws IOException, ParseException {
loadFrom( aFile, aDelimiters );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withLoadFrom( InputStream aInputStream ) throws IOException, ParseException {
loadFrom( aInputStream );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withLoadFrom( InputStream aInputStream, char... aDelimiters ) throws IOException, ParseException {
loadFrom( aInputStream, aDelimiters );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withLoadFrom( String aFilePath ) throws IOException, ParseException {
loadFrom( new File( aFilePath ) );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withLoadFrom( String aFilePath, char... aDelimiters ) throws IOException, ParseException {
loadFrom( aFilePath, aDelimiters );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withLoadFrom( URL aUrl ) throws IOException, ParseException {
loadFrom( aUrl );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withLoadFrom( URL aUrl, char... aDelimiters ) throws IOException, ParseException {
loadFrom( aUrl, aDelimiters );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withPut( Object aPath, String aValue ) {
put( toPath( aPath ), aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withPut( Object[] aPathElements, String aValue ) throws NumberFormatException {
put( toPath( aPathElements ), aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withPut( Relation aProperty ) {
put( aProperty );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withPut( String aKey, String aValue ) {
put( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withPut( String[] aPathElements, String aValue ) throws NumberFormatException {
put( toPath( aPathElements ), aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withPutBoolean( Object[] aPathElements, Boolean aValue ) {
putBoolean( toPath( aPathElements ), aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withPutBoolean( String aKey, Boolean aValue ) {
putBoolean( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withPutBoolean( String[] aPathElements, Boolean aValue ) {
putBoolean( toPath( aPathElements ), aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withPutByte( Object[] aPathElements, Byte aValue ) {
putByte( toPath( aPathElements ), aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withPutByte( String aKey, Byte aValue ) {
putByte( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withPutByte( String[] aPathElements, Byte aValue ) {
putByte( toPath( aPathElements ), aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withPutDouble( Object[] aPathElements, Double aValue ) {
putDouble( toPath( aPathElements ), aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withPutDouble( String aKey, Double aValue ) {
putDouble( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withPutDouble( String[] aPathElements, Double aValue ) {
putDouble( toPath( aPathElements ), aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withPutFloat( Object[] aPathElements, Float aValue ) {
putFloat( toPath( aPathElements ), aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withPutFloat( String aKey, Float aValue ) {
putFloat( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withPutFloat( String[] aPathElements, Float aValue ) {
putFloat( toPath( aPathElements ), aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withPutInteger( Object[] aPathElements, Integer aValue ) {
putInteger( toPath( aPathElements ), aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withPutInteger( String aKey, Integer aValue ) {
putInteger( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withPutInteger( String[] aPathElements, Integer aValue ) {
putInteger( toPath( aPathElements ), aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withPutLong( Object[] aPathElements, Long aValue ) {
putLong( toPath( aPathElements ), aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withPutLong( String aKey, Long aValue ) {
putLong( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withPutLong( String[] aPathElements, Long aValue ) {
putLong( toPath( aPathElements ), aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withPutShort( Object[] aPathElements, Short aValue ) {
putShort( toPath( aPathElements ), aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withPutShort( String aKey, Short aValue ) {
putShort( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withPutShort( String[] aPathElements, Short aValue ) {
putShort( toPath( aPathElements ), aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withRemoveFrom( String aPath ) {
removeFrom( aPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withSeekFrom( Class> aResourceClass, String aFilePath ) throws IOException, ParseException {
seekFrom( aResourceClass, aFilePath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withSeekFrom( Class> aResourceClass, String aFilePath, char... aDelimiters ) throws IOException, ParseException {
seekFrom( aFilePath, aDelimiters );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withSeekFrom( File aFile ) throws IOException, ParseException {
seekFrom( aFile );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withSeekFrom( File aFile, char... aDelimiters ) throws IOException, ParseException {
seekFrom( aFile, aDelimiters );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withSeekFrom( String aFilePath ) throws IOException, ParseException {
seekFrom( aFilePath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ObfuscationResourcePropertiesBuilder withSeekFrom( String aFilePath, char... aDelimiters ) throws IOException, ParseException {
seekFrom( aFilePath, aDelimiters );
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy