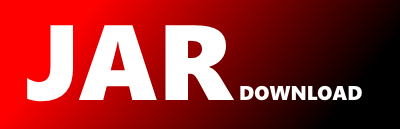
org.refcodes.cryptography.alt.filesystem.impls.FileSystemDecryptionServerImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-cryptography-alt-filesystem Show documentation
Show all versions of refcodes-cryptography-alt-filesystem Show documentation
Artifact for a file system based implementation of the cryptography framework.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// =============================================================================
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// =============================================================================
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// together with the GPL linking exception applied; as being applied by the GNU
// Classpath ("http://www.gnu.org/software/classpath/license.html")
// =============================================================================
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// =============================================================================
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.cryptography.alt.filesystem.impls;
import java.io.IOException;
import java.io.InputStream;
import java.io.ObjectInputStream;
import java.util.ArrayList;
import java.util.List;
import org.refcodes.cryptography.CipherVersion;
import org.refcodes.cryptography.DecryptionServer;
import org.refcodes.cryptography.traps.SignatureVerificationException;
import org.refcodes.exception.traps.HiddenException;
import org.refcodes.filesystem.FileHandle;
import org.refcodes.filesystem.FileSystem;
import org.refcodes.filesystem.traps.ConcurrentAccessException;
import org.refcodes.filesystem.traps.IllegalFileHandleException;
import org.refcodes.filesystem.traps.IllegalPathException;
import org.refcodes.filesystem.traps.NoListAccessException;
import org.refcodes.filesystem.traps.NoReadAccessException;
import org.refcodes.filesystem.traps.UnknownFileException;
import org.refcodes.filesystem.traps.UnknownFileSystemException;
import org.refcodes.filesystem.traps.UnknownPathException;
import org.refcodes.filesystem.utils.FileSystemUtility;
import org.refcodes.logger.RuntimeLogger;
import org.refcodes.logger.factories.impls.RuntimeLoggerFactorySingleton;
/**
* Abstract file system based implementation for non abstract
* {@link DecryptionServer} implementations.
*/
public class FileSystemDecryptionServerImpl implements DecryptionServer {
private static RuntimeLogger LOGGER = RuntimeLoggerFactorySingleton.getInstance().createInstance();
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private FileSystem _fileSystem;
private String _path;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTOR:
// /////////////////////////////////////////////////////////////////////////
/**
* Constructs the server with the required services and configuration
* passed.
*
* @param aFileSystem The data store service where to retrieve the
* Cipher-Versions from
* @param aPath The directory where the cryptography relevant data is being
* persisted
*/
public FileSystemDecryptionServerImpl( FileSystem aFileSystem, String aPath ) {
_fileSystem = aFileSystem;
_path = FileSystemUtility.toNormalizedPath( aPath );
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public List getCipherVersions( String aNameSpace, String aMessage, String aSignature ) throws SignatureVerificationException {
String theNameSpaceKey = FileSystemUtility.toKey( _path, aNameSpace );
LOGGER.debug( "Using file system key \"" + theNameSpaceKey + "\"." );
try {
List theFileHandles = _fileSystem.getFileHandles( theNameSpaceKey, true );
ObjectInputStream eObjectInputStream = null;
InputStream eInputStream = null;
CipherVersion eCipherVersion;
List theEncryptedCipherVersions = new ArrayList();
for ( FileHandle eFileHandle : theFileHandles ) {
LOGGER.debug( "Trying to load Cipher-Version from file (handle) with key \"" + eFileHandle.toKey() + "\" ..." );
try {
eInputStream = _fileSystem.fromFile( eFileHandle );
eObjectInputStream = new ObjectInputStream( eInputStream );
eCipherVersion = (CipherVersion) eObjectInputStream.readObject();
theEncryptedCipherVersions.add( eCipherVersion );
LOGGER.debug( "Loaded Cipher-Version from file (handle) with key \"" + eFileHandle.toKey() + "\"!" );
}
finally {
if ( eObjectInputStream != null ) try {
eObjectInputStream.close();
}
catch ( IOException e ) {
LOGGER.warn( "Unable to close output stream for file system path \"" + eFileHandle.getPath() + "\" and name \"" + eFileHandle.getName() + "\"!" );
}
if ( eInputStream != null ) try {
eInputStream.close();
}
catch ( IOException e ) {
LOGGER.warn( "Unable to close object output stream for file system path \"" + eFileHandle.getPath() + "\" and name \"" + eFileHandle.getName() + "\"!" );
}
}
}
return theEncryptedCipherVersions;
}
catch ( NoListAccessException e ) {
throw new HiddenException( e );
}
catch ( UnknownFileSystemException e ) {
throw new HiddenException( e );
}
catch ( UnknownPathException e ) {
throw new HiddenException( e );
}
catch ( IllegalPathException e ) {
throw new HiddenException( e );
}
catch ( IllegalFileHandleException e ) {
throw new HiddenException( e );
}
catch ( NoReadAccessException e ) {
throw new HiddenException( e );
}
catch ( ConcurrentAccessException e ) {
throw new HiddenException( e );
}
catch ( UnknownFileException e ) {
throw new HiddenException( e );
}
catch ( ClassNotFoundException e ) {
throw new HiddenException( e );
}
catch ( IOException e ) {
throw new HiddenException( e );
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy