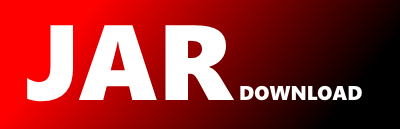
org.refcodes.data.AnsiEscapeCode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-data Show documentation
Show all versions of refcodes-data Show documentation
Artifact with commonly used constants.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.data;
import org.refcodes.mixin.CodeAccessor;
/**
* Some default ANSI Escape-Codes to be prepended or append to your ANSIfied
* {@link String} instances. Set a color e.g. with prepending
* {@link #FG_BLUE_BRIGHT} to your {@link String} and appending {@link #RESET}
* to reset the current ANSI console's state (an ANSI console remembers your
* settings till them are overwritten or reseted). The codes found here
* represent just a few possibilities which we placed here as them are used here
* and there throughout the REFCODES.ORG artifacts. For professional ANSI escape
* code handling see fusesource' jansi API at
* "https://github.com/fusesource/jansi".
*/
public enum AnsiEscapeCode implements CodeAccessor {
// @formatter:off
// RESET = ansi().reset().toString();
// FG_WHITE_UNDERLINE = ansi().a( Attribute.UNDERLINE ).fg( Color.WHITE ).toString();
// FG_WHITE_BRIGHT_BG_BLACK = ansi().fgBright( Color.WHITE ).bg( Color.BLACK ).toString();
// FG_RED_BRIGHT_BOLD = ansi().bold().fgBright( Color.RED ).toString();
// FG_WHITE_BRIGHT_BG_RED = ansi().fgBright( Color.WHITE ).bgBright( Color.RED ).toString();
// FG_GREEN_BRIGHT = ansi().fgBright( Color.GREEN ).toString();
// FG_RED = ansi().fg( Color.RED ).toString();
// FG_BLUE_BRIGHT_BOLD = ansi().fgBright( Color.BLUE ).bold().toString();
// FG_DEFAULT_BRIGHT = ansi().fgBright( Color.DEFAULT ).toString();
// FG_BLUE_BRIGHT = ansi().fgBright( Color.BLUE ).toString();
// FG_RED_BRIGHT = ansi().fgBright( Color.RED ).toString();
// @formatter:on
// @formatter:off
/**
* Reset the current ANSI console's color settings to default settings.
*/
RESET("\u001B[0m"),
/**
* Sets a white foreground (text) color.
*/
FG_WHITE("\u001B[37m"),
/**
* Sets a white underlined foreground (text) color.
*/
FG_WHITE_UNDERLINE("\u001B[4;37m"),
/**
* Sets a bright white foreground (text) with a black background color.
*/
FG_WHITE_BRIGHT_BG_BLACK("\u001B[97;40m"),
/**
* Sets a bright red foreground (text) color.
*/
FG_RED_BRIGHT_BOLD("\u001B[1;91m"),
/**
* Sets a bright white foreground (text) with a red background color.
*/
FG_WHITE_BRIGHT_BG_RED("\u001B[97;101m"),
/**
* Sets a bright green foreground (text) color.
*/
FG_GREEN_BRIGHT("\u001B[92m"),
/**
* Sets a red foreground (text) color.
*/
FG_RED("\u001B[31m"),
/**
* Sets a bright blue foreground (text) color.
*/
FG_BLUE_BRIGHT_BOLD("\u001B[94;1m"),
/**
* Sets a default bright foreground (text) color.
*/
FG_DEFAULT_BRIGHT("\u001B[99m"),
/**
* Sets a bright blue foreground (text) color.
*/
FG_BLUE_BRIGHT("\u001B[94m"),
/**
* Sets a bright red foreground (text) color.
*/
FG_RED_BRIGHT("\u001B[91m"),
/**
* Sets a bright yellow foreground (text) with a bright blue background color.
*/
FG_YELLOW_BRIGHT_BG_BLUE_BRIGHT("\u001B[93;104m"),
/**
* The ANSI Escape-Code with which each ANSI escape sequence is commenced.
*/
ESC( '\u001B' + "");
// @formatter:on
// /////////////////////////////////////////////////////////////////////////
// CONSTANTS:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private String _ansiEscapeCode;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Instantiates a new ansi escape code.
*
* @param aAnsiEscapeCode the ansi escape code
*/
private AnsiEscapeCode( String aAnsiEscapeCode ) {
_ansiEscapeCode = aAnsiEscapeCode;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public String getCode() {
return _ansiEscapeCode;
}
/**
* Returns the escaped ANSI Escape-Code to be printable without triggering
* the actual ANSI escape commands.
*
* @return The escaped ANSI Escape-Code.
*/
public String toEscaped() {
return _ansiEscapeCode.replaceAll( ESC.getCode(), "\\u001B" );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy