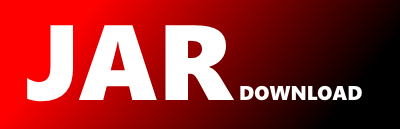
org.refcodes.data.Delimiter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-data Show documentation
Show all versions of refcodes-data Show documentation
Artifact with commonly used constants.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.data;
import java.nio.file.FileSystems;
import org.refcodes.mixin.CharAccessor;
/**
* Commonly used characters when working with separated values or properties and
* the like making use of text separator characters .
*/
public enum Delimiter implements CharAccessor {
/**
* A number (index) is prefixed by a hash ("#").
*/
INDEX('#'),
/**
* A fragment is the hash ("#") separated suffix of an URL.
*/
URL_FRAGMENT('#'),
/**
* User-info representing a credentials in an URL are separated be a colon
* (":").
*/
URL_CREDENTIALS(':'),
/**
* User-info (e.g. credentials) in an URL are separated be an ampersand
* ("@").
*/
URL_USER_INFO('@'),
/**
* The default path delimiter for all kinds of paths ("/"); the delimiter is
* used to compose address locators for resources from inside JAR files or
* for *nix alike operating systems. To get the operating system's file
* separator use {@link SystemProperty#FILE_SEPARATOR}.
*/
PATH('/'),
/**
* The path delimiter for all descendants of good old MS-DOS.
*/
DOS_PATH('\\'),
/**
* The system's file path delimiter as used by the underlying operating
* system.
*/
SYSTEM_FILE_PATH(FileSystems.getDefault().getSeparator() != null && FileSystems.getDefault().getSeparator().length() > 0 ? FileSystems.getDefault().getSeparator().charAt( 0 ) : Delimiter.PATH.getChar()),
/**
* Default delimiter for CSV (comma separated values) lines; this is not
* necessarily a comma (","), moreover we choose to use the semicolon ";".
*/
CSV(';'),
/**
* When concatenating the elements of an array to a single line, then per
* default this delimiter us used (":").
*/
ARRAY(':'),
/**
* IPv4 delimiter as of the CIDR notation (see
* "https://en.wikipedia.org/wiki/Classless_Inter-Domain_Routing#CIDR_notation").
*/
IP_V4('.'),
/**
* IPv6 delimiter as of the CIDR notation (see
* "https://en.wikipedia.org/wiki/Classless_Inter-Domain_Routing#CIDR_notation").
*/
IP_V6(':'),
/**
* When separating a host from a port in an URL, then per definition this
* delimiter is used (":").
*/
URL_PORT(':'),
/**
* When concatenating the elements of a list to a single line, then per
* default this delimiter us used (",").
*/
LIST(','),
/**
* When concatenating elements in a word written in snake case (e.g.
* "FIRST_LAST" for concatenating "first" with "last"), then this delimiter
* may be your choice ("_").
*
* @see "https://en.wikipedia.org/wiki/Snake_case"
*/
SNAKE_CASE('_'),
/**
* When concatenating elements in a word written in kebab case (e.g.
* "FIRST-LAST" for concatenating "first" with "last"), then this delimiter
* may be your choice ("-").
*
* @see "https://en.wikipedia.org/wiki/Kebab_case"
*/
KEBAB_CASE('-'),
/**
* A hierarchy such as a package structure often is represented by dot "."
* separated hierarchy "levels", e.g. "org.refcodes.data": The top level is
* "org", the next level is "refcodes", the fully qualified level
* representation would be "org.refcodes". The fully qualified level
* representation for the level "data" would be "org.refcodes.data". Got it?
* ;-)
*/
NAMESPACE('.'),
/**
* The delimiter separating properties (key/value-pairs/tuples) from each
* other (";").
*/
COOKIE_PROPERTIES(';'),
/**
* The delimiter separating a cookie's tuple name from its value ("=").
*/
COOKIE_TUPEL('='),
/**
* The delimiter separating a key from a value of a key/value property
* ("=").
*/
PROPERTY('='),
/**
* Separates the JAR file from the therein addressed resource ("!").
*/
JAR_URL_RESOURCE('!'),
/**
* The separator separating a method name from a class name ("#").
*/
METHOD_NAME('#'),
/**
* The separator separating an inner class from a class in a fully qualified
* class name ("$").
*/
INNER_CLASS('$'),
/**
* The separator separating the packages from a package hierarchy (".").
*/
PACKAGE_HIERARCHY('.'),
/**
* The delimiter char separating a cipher UID from an encrypted text (":").
*/
CIPHER_UID(':'),
/**
* The delimiter char separating correlation IDs from each other (":").
*/
CORRELATION_ID(':'),
/**
* The delimiter char separating one web field from another in a query
* string of an URL ("&").
*/
WEB_FIELD('&'),
/**
* The delimiter char separating the locator part from a query string of an
* URL ("?").
*/
URL_QUERY('?'),
/**
* The delimiter separating a HTTP header's media type declaration from the
* (optional) parameters and the parameters from each other (";").
*/
MEDIA_TYPE_PARAMETERS(';'),
/**
* The delimiter in HTTP headers for multiple elements in a single header
* field, e.g. in the ACCEPT Header-Field separating multiple media type
* declarations from each other (",").
*/
HTTP_HEADER_ELEMENTS(',');
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private char _delimeter;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Instantiates a new delimiter.
*
* @param aDelimieter the delimieter
*/
private Delimiter( char aDelimieter ) {
_delimeter = aDelimieter;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public char getChar() {
return _delimeter;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy