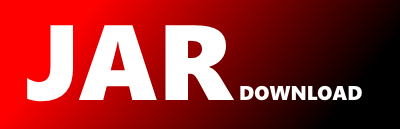
org.refcodes.data.EnvironmentVariable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-data Show documentation
Show all versions of refcodes-data Show documentation
Artifact with commonly used constants.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.data;
import org.refcodes.mixin.KeyAccessor;
import org.refcodes.mixin.ValueAccessor.ValueProperty;
/**
* {@link EnvironmentVariable} for retrieving some common environment variables
* with ease.
*/
public enum EnvironmentVariable implements KeyAccessor, ValueProperty {
/**
* The virtual and the physical console width height and environment
* variable. E.g. ANSICON=80x19999 (80x25)
*/
ANSICON("ANSICON"),
/**
* ConEmu's environment variable regarding ANSI support.
*/
CONSOLE_CONEMU_ANSI("ConEmuANSI"),
/**
* Stands for the path to the directory where you placed configuration files
* such as the "runtimelogger.ini
" file.
*/
// CONFIG_DIR("CONFIG_DIR"),
/**
* The console height environment variable.
*/
TERMINAL_HEIGHT("LINES"),
/**
* The console width environment variable on *ix like shells.
*/
TERMINAL_WIDTH("COLUMNS"),
/**
* Stands for the chars per row to be taken by REFCODES.ORG artifacts.
*/
CONSOLE_WIDTH("CONSOLE_WIDTH"),
/**
* Stands for the lines per column to be taken by REFCODES.ORG artifacts.
*/
CONSOLE_HEIGHT("CONSOLE_HEIGHT"),
/**
* The console's line-break property. Used to override any default line
* breaks for the REFCODES-ORG artifacts.
*/
CONSOLE_LINE_BREAK("CONSOLE_LINE_BREAK"),
/**
* Set to "true" or "false", forces ANSI to be used / not used by
* REFCODES.ORG artifacts, no matter what capabilities were detected for the
* hosting terminal.
*/
CONSOLE_ANSI("CONSOLE_ANSI"),
/**
* Stands for the chosen layout for the REFCODES.ORG logger artifacts.
*/
LOGGER_LAYOUT("LOGGER_LAYOUT"),
/**
* Stands for the chosen style for the REFCODES.ORG logger artifacts.
*/
LOGGER_STYLE("LOGGER_STYLE"),
/**
* The environment variable (on windows) holding the computer's name.
*/
COMPUTERNAME("COMPUTERNAME"),
/**
* The environment variable (on windows) holding the computer's name.
*/
HOSTNAME("HOSTNAME"),
/**
* Environment variable holding the user's home folder path.
*/
USER_HOME("HOME"),
/**
* Environment variable holding the current session's terminal.
*/
TERM("TERM"),
/**
* Number of processors.
*/
NUMBER_OF_PROCESSORS("NUMBER_OF_PROCESSORS"),
/**
* Environment variable "HOST_SEED" for the Host-Seed for host-related IDs.
*/
HOST_SEED("HOST_SEED"),
/**
* HTTP-Proxy setting in URL notation ("http://my.company.org:3128")
*/
HTTP_PROXY("HTTP_PROXY"),
/**
* HTTPS-Proxy setting in URL notation ("http://my.company.org:3128")
*/
HTTPS_PROXY("HTTPS_PROXY"),
/**
* No-Proxy settings for the {@link #HTTP_PROXY} ({@link #HTTPS_PROXY})
* settings in a comma separated list
* ("localhost,127.0.0.0/8,127.0.1.1,127.0.1.1*,...")
*/
NO_PROXY("NO_PROXY"),
/**
* Processor architecture on some systems (e.g. "AMD64").
*/
PROCESSOR_ARCHITECTURE("PROCESSOR_ARCHITECTURE"),
/**
* Processor architecture on some systems (e.g. "AMD64").
*/
PROCESSOR_ARCHITEW6432("PROCESSOR_ARCHITEW6432");
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private String _envVariableName;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Instantiates a new "environment variable".
*
* @param aEnvVariableName the environment variable's name
*/
private EnvironmentVariable( String aEnvVariableName ) {
_envVariableName = aEnvVariableName;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public String getKey() {
return _envVariableName;
}
/**
* Retrieves the value, first the upper-case variant of the environment
* variable is tried out. If not set or empty, then the lower-case variant
* is used.
*
* @return The value of the environment variable.
*/
@Override
public String getValue() {
String theValue = System.getenv( _envVariableName.toUpperCase() );
if ( theValue == null || theValue.length() == 0 ) {
theValue = System.getenv( _envVariableName.toLowerCase() );
if ( theValue == null || theValue.length() == 0 ) {
theValue = System.getenv( _envVariableName );
}
}
return theValue;
}
/**
* Sets the value, first the upper-case variant of the environment variable
* is tried out. If not set or empty, then the lower-case variant is tried.
*
* @param aValue the new value
*/
@Override
public void setValue( String aValue ) {
String theValue = System.getenv( _envVariableName.toUpperCase() );
if ( theValue == null || theValue.length() == 0 ) {
theValue = System.getenv( _envVariableName.toLowerCase() );
if ( theValue != null && theValue.length() != 0 ) {
System.getenv().put( _envVariableName.toLowerCase(), aValue );
return;
}
else {
theValue = System.getenv( _envVariableName );
if ( theValue != null && theValue.length() != 0 ) {
System.getenv().put( _envVariableName, aValue );
return;
}
}
}
System.getenv().put( _envVariableName.toUpperCase(), aValue );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy