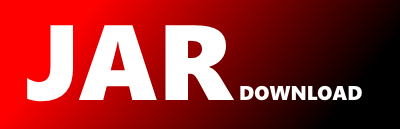
org.refcodes.data.Scheme Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-data Show documentation
Show all versions of refcodes-data Show documentation
Artifact with commonly used constants.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// =============================================================================
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// =============================================================================
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// together with the GPL linking exception applied; as being applied by the GNU
// Classpath ("http://www.gnu.org/software/classpath/license.html")
// =============================================================================
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// =============================================================================
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.data;
import org.refcodes.mixin.NameAccessor;
import org.refcodes.mixin.PortAccessor;
/**
* The {@link Scheme} define values useful when working with files or a
* file-system. An URL-Scheme is constructed as follows:
*
* "name:scheme-specific-part"
*
* The name is the protocol of the scheme such as "file" or "http". The scheme
* specific part is the part after the colon (":") specific to the given scheme.
* For a "file" scheme it would be just an empty {@link String} where as for
* "http" it would be "//" (as of "http://", "http" being the name).
*/
public enum Scheme implements NameAccessor, PortAccessor {
JAR("jar", "", FilenameExtension.JAR.getFilenameExtension() + Delimiter.JAR_URL_RESOURCE.getChar()),
ZIP("zip", "", FilenameExtension.ZIP.getFilenameExtension() + Delimiter.JAR_URL_RESOURCE.getChar()),
SH("sh", "", FilenameExtension.SH.getFilenameExtension() + Delimiter.JAR_URL_RESOURCE.getChar()),
FILE("file", "", "file" + Suffix.PROTOCOL.getSuffix() + Delimiter.PATH.getChar()),
HTTP("http", "" + Delimiter.PATH.getChar() + Delimiter.PATH.getChar(), Port.HTTP.getPort()),
HTTPS("https", "" + Delimiter.PATH.getChar() + Delimiter.PATH.getChar(), Port.HTTPS.getPort()),
SOCKS("socks", "" + Delimiter.PATH.getChar() + Delimiter.PATH.getChar()),
SOCKS4("socks4", "" + Delimiter.PATH.getChar() + Delimiter.PATH.getChar()),
SOCKS5("socks5", "" + Delimiter.PATH.getChar() + Delimiter.PATH.getChar()),
UNKNOWN("???", "");
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private String _name;
private String _specific;
private String _marker;
private int _port = -1;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Instantiates a new protocol.
*
* @param aName The protocol name e.g. scheme.
* @param aSpecific The scheme's specific suffix of the name.
*/
private Scheme( String aName, String aSpecific ) {
_name = aName;
_specific = aSpecific;
_marker = aName + Suffix.PROTOCOL.getSuffix() + _specific;
}
/**
* Instantiates a new protocol.
*
* @param aName The protocol name e.g. scheme.
* @param aSpecific The scheme's specific suffix of the name.
* @param aPort If applicable, then the port!
*/
private Scheme( String aName, String aSpecific, int aPort ) {
_name = aName;
_specific = aSpecific;
_marker = aName + Suffix.PROTOCOL.getSuffix() + _specific;
_port = aPort;
}
/**
* Instantiates a new protocol.
*
* @param aName The protocol name e.g. scheme.
* @param aSpecific The scheme's specific suffix of the name.
* @param aMarker The marker for a locator's "inner" scheme declaration
*/
private Scheme( String aName, String aSpecific, String aMarker ) {
_name = aName;
_specific = aSpecific;
_marker = aMarker;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public String getName() {
return _name;
}
/**
* Returns the scheme's specific part as of "name:scheme-specific-part". The
* double-slash "//" is the scheme-specific-part of "http://".
*
* @return The scheme's specific part. For HTTP it would be "//".
*/
public String getSpecific() {
return _specific;
}
/**
* Returns protocol being the scheme name and the scheme specific part ,
* e.g. "http://" or "file:".
*
* @return The protocol.
*/
public String toProtocol() {
return _name + Suffix.PROTOCOL.getSuffix() + _specific;
}
/**
* Returns the locator part pointing into the resource of the given URL.
*
* @param aUrl The URL from which to retrieve the locator part.
* @param aBeginIndex The index from where to start.
*
* @return The locator part of the given URL or null if there is no valid
* resource protocol found.
*/
public String toUrl( String aUrl, int aBeginIndex ) {
String theLocator = aUrl;
int i = theLocator.indexOf( _marker, aBeginIndex );
if ( i != -1 ) {
i += (_marker.length() - 1);
return theLocator.substring( 0, i );
}
return null;
}
/**
* Returns the locator part pointing into the resource of the given URL.
*
* @param aUrl The URL from which to retrieve the locator part.
*
* @return The locator part of the given URL or null if there is no valid
* resource protocol found.
*/
public String toUrl( String aUrl ) {
return toUrl( aUrl, 0 );
}
/**
* Returns the index of the locator part pointing into the resource of the
* given URL.
*
* @param aUrl The URL from which to retrieve the locator part.
* @param aBeginIndex The index from where to start.
*
* @return The index of locator part of the given URL or -1 if there is no
* valid resource protocol found.
*/
public int nextMarkerIndex( String aUrl, int aBeginIndex ) {
int i = aUrl.indexOf( _marker, aBeginIndex );
if ( i != -1 ) {
i += (_marker.length() - 1);
}
return i;
}
/**
* Returns the index of locator part pointing into the resource of the given
* URL.
*
* @param aUrl The URL from which to retrieve the locator part.
*
* @return The the index locator part of the given URL or -1 if there is no
* valid resource protocol found.
*/
public int firstMarkerIndex( String aUrl ) {
int i = aUrl.indexOf( _marker, 0 );
if ( i != -1 ) {
i += (_marker.length() - 1);
}
return i;
}
/**
* Returns the prefix for the resource locator as of the {@link Scheme}.
* E.g. a HTTP protocol will provide the resource locator prefix "http://"
* and a FILE protocol will provide the resource locator prefix "file:/"
* prefix.
*
* @return The resource locator's prefix.
*/
public String getMarker() {
return _marker;
}
/**
* {@inheritDoc}
*/
@Override
public int getPort() {
return _port;
}
// /////////////////////////////////////////////////////////////////////////
// HELPER:
// /////////////////////////////////////////////////////////////////////////
/**
* Returns that {@link Scheme} represented by the given name.
*
* @param aName The name for which to determine the {@link Scheme}.
*
* @return The determined {@link Scheme} or null if none was determinable.
*/
public static Scheme fromName( String aName ) {
if ( aName != null ) {
for ( Scheme eElement : values() ) {
if ( eElement.getName().equalsIgnoreCase( aName ) ) {
return eElement;
}
}
}
return null;
}
/**
* Returns that {@link Scheme} represented by the given protocol.
*
* @param aProtocol The protocol for which to determine the {@link Scheme}.
*
* @return The determined {@link Scheme} or null if none was determinable.
*/
public static Scheme fromProtocol( String aProtocol ) {
if ( aProtocol != null ) {
for ( Scheme eElement : values() ) {
if ( eElement.toProtocol().toLowerCase().startsWith( aProtocol.toLowerCase() ) ) {
return eElement;
}
}
}
return null;
}
/**
* Determines the {@link Scheme} from the given URL.
*
* @param aUrl The URL from which to determine the {@link Scheme}.
*
* @return The according {@link Scheme} or null if none matching was found.
*/
public static Scheme fromScheme( String aUrl ) {
if ( aUrl != null ) {
for ( Scheme eElement : values() ) {
if ( aUrl.toLowerCase().startsWith( eElement.toProtocol().toLowerCase() ) ) {
return eElement;
}
}
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy