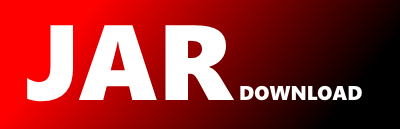
org.refcodes.data.SystemProperty Maven / Gradle / Ivy
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.data;
import org.refcodes.mixin.KeyAccessor;
import org.refcodes.mixin.ValueAccessor.ValueProperty;
/**
* {@link SystemProperty} define values to be used at runtime. Them are passed
* to a JVM by prefixing a "-D" to the actual "property=value" pair.
*/
public enum SystemProperty implements KeyAccessor, ValueProperty {
/**
* HTTP-Proxy host without a port ("http://my.company.org")
*/
HTTP_PROXY_HOST("http.proxyHost"),
/**
* HTTP-Proxy port ("3128")
*/
HTTP_PROXY_PORT("http.proxyPort"),
/**
* HTTPS-Proxy host without a port ("http://my.company.org")
*/
HTTPS_PROXY_HOST("https.proxyHost"),
/**
* HTTP-Proxy port ("3128")
*/
HTTPS_PROXY_PORT("https.proxyPort"),
/**
* SOCKS-Proxy host without a port ("http://my.company.org")
*/
SOCKS_PROXY_HOST("socksProxyHost"),
/**
* SOCKS-Proxy port ("3128")
*/
SOCKS_PROXY_PORT("socksProxyPort"),
/**
* System property "host.seed" for the Host-Seed for host-related IDs.
*/
HOST_SEED("host.seed"),
/**
* No-Proxy settings for the {@link #HTTP_PROXY_HOST}
* ({@link #HTTP_PROXY_PORT}) settings in a comma separated list
* ("localhost,127.0.0.0/8,127.0.1.1,127.0.1.1*,...")
*/
HTTP_NON_PROXY_HOSTS("http.nonProxyHosts"),
/**
* No-Proxy settings for the {@link #HTTPS_PROXY_HOST}
* ({@link #HTTPS_PROXY_PORT}) settings in a comma separated list
* ("localhost,127.0.0.0/8,127.0.1.1,127.0.1.1*,...")
*/
HTTPS_NON_PROXY_HOSTS("https.nonProxyHosts"),
/**
* Processor architecture on some systems (e.g. "AMD64").
*/
OS_ARCH("os.arch"),
/**
* Pass as JVM argument via "-Dconfig.dir=path_to_your_config_dir" (where
* path_to_your_config_dir stands for the path to the directory where you
* placed configuration files such as the "runtimelogger.ini
"
* file).
*/
CONFIG_DIR("config.dir"),
/**
* The OS specific file separator is retrieved by this system property.
*
* ATTENTION: System properties are to be retrieved via
* {@link System#getProperty(String)}.
*/
FILE_SEPARATOR("file.separator"),
/**
* The OS specific temp folder path.
*/
TEMP_DIR("java.io.tmpdir"),
/** At least set on Ubuntu-Linux:. */
PROCESS_ID("PID"),
/**
* Sequence used by operating system to separate lines in text files.
*/
LINE_SEPARATOR("line.separator"),
/**
* Operating system name.
*/
OPERATING_SYSTEM_NAME("os.name"),
/**
* Pass as JVM argument via "-Dconsole.height=n" (where n stands for the
* number of lines).
*/
CONSOLE_HEIGHT("console.height"),
/**
* Pass as JVM argument via "-Dconsole.width=n" (where n stands for the
* number of chars per row).
*/
CONSOLE_WIDTH("console.width"),
/**
* Pass as JVM argument via -Dconsole.ansi=<true|false>
* where "true" or "false" forces ANSI to be used / not used by REFCODES.ORG
* artifacts, no matter what capabilities were detected for the hosting
* terminal.
*/
CONSOLE_ANSI("console.ansi"),
/**
* The console's line-break property. Used to override any default line
* breaks for the REFCODES-ORG artifacts.
*/
CONSOLE_LINE_BREAK("console.lineBreak"),
/**
* Pass as JVM argument via "-Dlogger.layout=<layout>
"
* (where <layout>
stands for the chosen layout for the
* REFCODES.ORG logger artifacts.).
*/
LOGGER_LAYOUT("logger.layout"),
/**
* Pass as JVM argument via "-Dlogger.style=<style>
"
* (where <style>
stands for the chosen logger-style for
* the REFCODES.ORG logger artifacts.).
*/
LOGGER_STYLE("logger.style"),
/**
* System variable holding the user's home folder path.
*/
USER_HOME("user.home"),
/**
* System variable holding the user's login name.
*/
USER_NAME("user.name"),
/**
* Location of the Java keystore file containing an application process's
* own certificate and private key.
*/
KEY_STORE_FILE("javax.net.ssl.keyStore"),
/**
* Password to access the private key from the keystore file specified by
* {@link #KEY_STORE_FILE}.
*/
KEY_STORE_PASSWORD("javax.net.ssl.keyStorePassword"),
/**
* For Java keystore file format, this property has the value "jks" (or
* "JKS"). Its default value is already "jks".
*/
KEY_STORE_TYPE("javax.net.ssl.keyStoreType"),
/**
* Location of the Java keystore file containing the collection of CA
* certificates trusted by this application process (trust store).
*/
TRUST_STORE_FILE("javax.net.ssl.trustStore"),
/**
* Password to unlock the keystore file (store password) specified by
* {@link #TRUST_STORE_FILE}.
*/
TRUST_STORE_PASSWORD("javax.net.ssl.trustStorePassword"),
/**
* For Java keystore file format, this property has the value "jks" (or
* "JKS"). Its default value is already "jks".
*/
TRUST_STORE_TYPE("javax.net.ssl.trustStoreType");
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private String _systemPropertyName;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Instantiates a new system property.
*
* @param aSystemPropertyName the system property name
*/
private SystemProperty( String aSystemPropertyName ) {
_systemPropertyName = aSystemPropertyName;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public String getKey() {
return _systemPropertyName;
}
/**
* {@inheritDoc}
*/
@Override
public String getValue() {
return System.getProperty( _systemPropertyName );
}
/**
* {@inheritDoc}
*/
@Override
public void setValue( String aValue ) {
System.setProperty( _systemPropertyName, aValue );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy