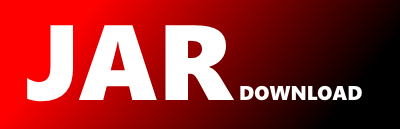
org.refcodes.data.MemoryUnit Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-data Show documentation
Show all versions of refcodes-data Show documentation
Artifact with commonly used constants.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.data;
import java.math.BigDecimal;
/**
* The {@link MemoryUnit} provides enumerations for common memory units
* alongside conversions to and from memory size specified in bytes.
*/
public enum MemoryUnit {
// /////////////////////////////////////////////////////////////////////////
// STATICS:
// /////////////////////////////////////////////////////////////////////////
BYTE("Byte", new BigDecimal( 1 )),
KILOBYTE("KByte", new BigDecimal( 1024 )),
MEGABYTE("MByte", new BigDecimal( 1024 ).multiply( new BigDecimal( 1024 ) )),
GIGABYTE("GByte", new BigDecimal( 1024 ).multiply( new BigDecimal( 1024 ) ).multiply( new BigDecimal( 1024 ) )),
TERABYTE("TByte", new BigDecimal( 1024 ).multiply( new BigDecimal( 1024 ) ).multiply( new BigDecimal( 1024 ) ).multiply( new BigDecimal( 1024 ) )),
PETABYTE("PByte", new BigDecimal( 1024 ).multiply( new BigDecimal( 1024 ) ).multiply( new BigDecimal( 1024 ) ).multiply( new BigDecimal( 1024 ) ).multiply( new BigDecimal( 1024 ) )),
EXABYTE("EByte", new BigDecimal( 1024 ).multiply( new BigDecimal( 1024 ) ).multiply( new BigDecimal( 1024 ) ).multiply( new BigDecimal( 1024 ) ).multiply( new BigDecimal( 1024 ) ).multiply( new BigDecimal( 1024 ) )),
ZETTABYTE("ZByte", new BigDecimal( 1024 ).multiply( new BigDecimal( 1024 ) ).multiply( new BigDecimal( 1024 ) ).multiply( new BigDecimal( 1024 ) ).multiply( new BigDecimal( 1024 ) ).multiply( new BigDecimal( 1024 ) ).multiply( new BigDecimal( 1024 ) )),
YOTTABYTE("YByte", new BigDecimal( 1024 ).multiply( new BigDecimal( 1024 ) ).multiply( new BigDecimal( 1024 ) ).multiply( new BigDecimal( 1024 ) ).multiply( new BigDecimal( 1024 ) ).multiply( new BigDecimal( 1024 ) ).multiply( new BigDecimal( 1024 ) ).multiply( new BigDecimal( 1024 ) ));
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private String _unit;
private BigDecimal _bytes;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
private MemoryUnit( String aUnit, BigDecimal aBytes ) {
_unit = aUnit;
_bytes = aBytes;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* Returns the name of the memory unit, e.g. "KB" for kilobyte or "MB" for
* megabyte.
*
* @return The name (abbreviation) of the unit.
*/
public String getUnit() {
return _unit;
}
/**
* Returns the number of bytes representing a unit, e.g. 1024 for
* {@link MemoryUnit#KILOBYTE}.
*
* @return The number of bytes per unit.
*/
public BigDecimal getBytes() {
return _bytes;
}
/**
* Calculates the number of bytes for the given value provided for the
* according {@link MemoryUnit}.
*
* @param aUnitValue The value to be converted.
*
* @return The converted value in bytes.
*/
public BigDecimal toBytes( double aUnitValue ) {
return _bytes.multiply( new BigDecimal( aUnitValue ) );
}
/**
* Calculates the number of bytes for the given value provided for the
* according {@link MemoryUnit}.
*
* @param aUnitValue The value to be converted.
*
* @return The converted value in bytes.
*/
public BigDecimal toBytes( BigDecimal aUnitValue ) {
return _bytes.multiply( aUnitValue );
}
/**
* Calculates the number of bytes for the given value provided for the
* according {@link MemoryUnit}.
*
* @param aUnitValue The value to be converted.
*
* @return The converted value in bytes.
*/
public BigDecimal toBytes( long aUnitValue ) {
return _bytes.multiply( new BigDecimal( aUnitValue ) );
}
/**
* Calculates the number of bytes for the given value provided for the
* according {@link MemoryUnit}.
*
* @param aBytes the bytes
*
* @return The converted value in bytes.
*/
public BigDecimal fromBytes( long aBytes ) {
return new BigDecimal( aBytes ).divide( _bytes );
}
/**
* Calculates the number of bytes for the given value provided for the
* according {@link MemoryUnit}.
*
* @param aBytes the bytes
*
* @return The converted value in bytes.
*/
public BigDecimal fromBytes( BigDecimal aBytes ) {
return aBytes.divide( _bytes );
}
/**
* Determines the best fitting unit to represent the given number of bytes
* in terms of "which memory unit is the biggest memory unit of which one
* unit is smaller than the given amount of bytes".
*
* @param aBytes The number of bytes for which to determine the most
* suitable {@link MemoryUnit}
*
* @return The according {@link MemoryUnit};
*/
public static MemoryUnit toSuitableUnit( long aBytes ) {
return toSuitableUnit( new BigDecimal( aBytes ) );
}
/**
* Determines the best fitting unit to represent the given number of bytes
* in terms of "which memory unit is the biggest memory unit of which one
* unit is smaller than the given amount of bytes".
*
* @param aBytes The number of bytes for which to determine the most
* suitable {@link MemoryUnit}
*
* @return The according {@link MemoryUnit};
*/
public static MemoryUnit toSuitableUnit( BigDecimal aBytes ) {
MemoryUnit eBestUnit = BYTE;
for ( MemoryUnit eUnit : values() ) {
if ( aBytes.divide( eUnit.getBytes() ).longValue() < 1 ) {
return eBestUnit;
}
eBestUnit = eUnit;
}
return eBestUnit;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy