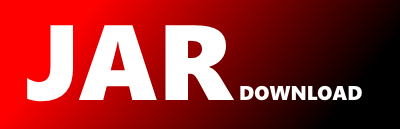
org.refcodes.data.Text Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-data Show documentation
Show all versions of refcodes-data Show documentation
Artifact with commonly used constants.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// =============================================================================
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// =============================================================================
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// together with the GPL linking exception applied; as being applied by the GNU
// Classpath ("http://www.gnu.org/software/classpath/license.html")
// =============================================================================
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// =============================================================================
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.data;
import org.refcodes.licensing.License;
import org.refcodes.mixin.TextAccessor;
/**
* Commonly used text blocks in the code.
*/
public enum Text implements TextAccessor {
REFCODES_ORG("REFCODES.ORG"),
UNSUPPORTED_OPERATION("*** OPERATION NOT SUPPORTED ***"),
ILLEGAL_STATE("Illegal state detected for invoking the operation; this instance's state does not allow this operation (it might be disposed, destroyed, decomposed, closed or similar)."),
NOT_YET_IMPLEMENTED("*** NOT YET IMPLEMENTED ***"),
NULL_VALUE("(null)"),
// @formatter:off
LICENSE( License.TEXT ),
// @formatter:on
// @formatter:off
/**
* See "https://en.wikipedia.org/wiki/Arecibo_message"
*/
ARECIBO_MESSAGE( new String[] {
"00000010101010000000000",
"00101000001010000000100",
"10001000100010010110010",
"10101010101010100100100",
"00000000000000000000000",
"00000000000011000000000",
"00000000001101000000000",
"00000000001101000000000",
"00000000010101000000000",
"00000000011111000000000",
"00000000000000000000000",
"11000011100011000011000",
"10000000000000110010000",
"11010001100011000011010",
"11111011111011111011111",
"00000000000000000000000",
"00010000000000000000010",
"00000000000000000000000",
"00001000000000000000001",
"11111000000000000011111",
"00000000000000000000000",
"11000011000011100011000",
"10000000100000000010000",
"11010000110001110011010",
"11111011111011111011111",
"00000000000000000000000",
"00010000001100000000010",
"00000000001100000000000",
"00001000001100000000001",
"11111000001100000011111",
"00000000001100000000000",
"00100000000100000000100",
"00010000001100000001000",
"00001100001100000010000",
"00000011000100001100000",
"00000000001100110000000",
"00000011000100001100000",
"00001100001100000010000",
"00010000001000000001000",
"00100000001100000000100",
"01000000001100000000100",
"01000000000100000001000",
"00100000001000000010000",
"00010000000000001100000",
"00001100000000110000000",
"00100011101011000000000",
"00100000001000000000000",
"00100000111110000000000",
"00100001011101001011011",
"00000010011100100111111",
"10111000011100000110111",
"00000000010100000111011",
"00100000010100000111111",
"00100000010100000110000",
"00100000110110000000000",
"00000000000000000000000",
"00111000001000000000000",
"00111010100010101010101",
"00111000000000101010100",
"00000000000000101000000",
"00000000111110000000000",
"00000011111111100000000",
"00001110000000111000000",
"00011000000000001100000",
"00110100000000010110000",
"01100110000000110011000",
"01000101000001010001000",
"01000100100010010001000",
"00000100010100010000000",
"00000100001000010000000",
"00000100000000010000000",
"00000001001010000000000",
"01111001111101001111000"
});
// @formatter:on
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private String[] _textBlock;
private String _text;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Instantiates a new text.
*
* @param aText the text
*/
private Text( String aText ) {
_text = aText;
_textBlock = new String[] { aText };
}
/**
* Instantiates a new text.
*
* @param aTextBlock the text block
*/
private Text( String[] aTextBlock ) {
_textBlock = aTextBlock;
final StringBuilder theText = new StringBuilder();
for ( String eText : aTextBlock ) {
if ( theText.length() != 0 ) {
theText.append( System.lineSeparator() );
}
theText.append( eText );
}
_text = theText.toString();
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public String getText() {
return _text;
}
/**
* {@inheritDoc}
*/
@Override
public String toString() {
return _text;
}
/**
* Returns the text block representation of this element.
*
* @return The text block representation.
*/
public String[] toStrings() {
return _textBlock;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy