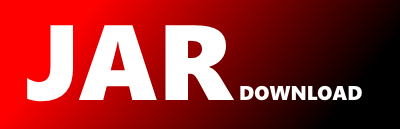
org.refcodes.data.AnsiEscapeCode Maven / Gradle / Ivy
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.data;
import java.util.ArrayList;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.refcodes.mixin.CodeAccessor;
/**
* This enumeration contains ANSI Escape-Codes for modifying the appearance of
* console output to ANSI enabled terminals. In order to compose your basic ANSI
* Escape-Code sequences, you may go for
* {@link #toEscapeSequence(AnsiEscapeCode...)}. Use
* {@link #toEscapedSequence(String)} when you wish to print the ANSI
* Escape-Code sequence without the sequence modifying your terminal output
* (e.g. helpful in error messages regarding ANSI Escape-Codes).
*/
public enum AnsiEscapeCode implements CodeAccessor {
/**
* Reset / Normal: All attributes off (reset).
*/
RESET((byte) 0),
/**
* Bold or increased intensity:
*/
BOLD((byte) 1),
/**
* Faint (decreased intensity):
*/
FAINT((byte) 2),
/**
* Italic: Not widely supported. Sometimes treated as inverse.
*/
ITALIC((byte) 3),
/**
* Underline
*/
UNDERLINE((byte) 4),
/**
* Slow Blink: Less than 150 per minute.
*/
SLOW_BLINK((byte) 5),
/**
* Rapid Blink: 150+ per minute. Not widely supported.
*/
RAPID_BLINK((byte) 6),
/**
* Reverse Video: Swap foreground and background colors.
*/
REVERSE_VIDEO((byte) 7),
/**
* Conceal: Not widely supported.
*/
CONCEAL((byte) 8),
/**
* Crossed-out: Characters legible but marked for deletion.
*/
CROSSED_OUT((byte) 9),
/**
* Primary(default) font
*/
DEFAULT_FONT((byte) 10),
/**
* Font 1: Select alternative font 1.
*/
FONT_1((byte) 11),
/**
* Font 2: Select alternative font 2.
*/
FONT_2((byte) 12),
/**
* Font 3: Select alternative font 3.
*/
FONT_3((byte) 13),
/**
* Font 4: Select alternative font 4.
*/
FONT_4((byte) 14),
/**
* Font 5: Select alternative font 5.
*/
FONT_5((byte) 15),
/**
* Font 6: Select alternative font 6.
*/
FONT_6((byte) 16),
/**
* Font 7: Select alternative font 7.
*/
FONT_7((byte) 17),
/**
* Font 8: Select alternative font 8.
*/
FONT_8((byte) 18),
/**
* Font 9: Select alternative font 9.
*/
FONT_9((byte) 19),
/**
* Fraktur: Rarely supported.
*/
FRAKTUR((byte) 20),
/**
* Doubly underline or Bold off: Double-underline per ECMA-48.
*/
DOUBLY_UNDERLINE_OR_BOLD_OFF((byte) 21),
/**
* Normal color or intensity: Neither bold nor faint.
*/
NORMAL_COLOR_OR_INTENSITY((byte) 22),
/**
* Not italic: Not Fraktur.
*/
NOT_ITALIC_NOT_FRAKTUR((byte) 23),
/**
* Underline off: Not singly or doubly underlined.
*/
UNDERLINE_OFF((byte) 24),
/**
* Blink off
*/
BLINK_OFF((byte) 25),
/**
* Inverse off
*/
INVERSE_OFF((byte) 27),
/**
* Reveal: Conceal off.
*/
REVEAL((byte) 28),
/**
* Not crossed out
*/
NOT_CROSSED_OUT((byte) 29),
/**
* Foreground Black: Set foreground color.
*/
FG_BLACK((byte) 30),
/**
* Foreground Red: Set foreground color.
*/
FG_RED((byte) 31),
/**
* Foreground Green: Set foreground color.
*/
FG_GREEN((byte) 32),
/**
* Foreground Yellow: Set foreground color.
*/
FG_YELLOW((byte) 33),
/**
* Foreground Blue: Set foreground color.
*/
FG_BLUE((byte) 34),
/**
* Foreground Magenta: Set foreground color.
*/
FG_MAGENTA((byte) 35),
/**
* Foreground Cyan: Set foreground color.
*/
FG_CYAN((byte) 36),
/**
* Foreground White: Set foreground color.
*/
FG_WHITE((byte) 37),
/**
* Set foreground color: Next arguments are 5;n
or
* 2;r;g;b
, see below:
*
* ESC[38:5:(n)m
: Select foreground color, (n) represents
* an index into a predefined 256-color lookup table
* ESC[38;2;(r);(g);(b)m
: Select RGB foreground color, (r)
* represents the 8 bit red portion of an RGB color, (g) represents the 8
* bit green portion of an RGB color, (b) represents the 8 bit blue portion
* of an RGB color
*
*/
SET_FOREGROUND_COLOR((byte) 38),
/**
* Default foreground color: implementation defined (according to standard)
*/
DEFAULT_FOREGROUND_COLOR((byte) 39),
/**
* Background Black: Set background color.
*/
BG_BLACK((byte) 40),
/**
* Background Red: Set background color.
*/
BG_RED((byte) 41),
/**
* Background Green: Set background color.
*/
BG_GREEN((byte) 42),
/**
* Background Yellow: Set background color.
*/
BG_YELLOW((byte) 43),
/**
* Background Blue: Set background color.
*/
BG_BLUE((byte) 44),
/**
* Background Magenta: Set background color.
*/
BG_MAGENTA((byte) 45),
/**
* Background Cyan: Set background color.
*/
BG_CYAN((byte) 46),
/**
* Background White: Set background color.
*/
BG_WHITE((byte) 47),
/**
* Set background color: Next arguments are 5;n
or
* 2;r;g;b
, see below:
*
* ESC[48:5:(n)m
; Select background color, (n) represents
* an index into a predefined 256-color lookup table
* ESC[48;2;(r);(g);(b)m
: Select RGB background color, (r)
* represents the 8 bit red portion of an RGB color, (g) represents the 8
* bit green portion of an RGB color, (b) represents the 8 bit blue portion
* of an RGB color
*
*/
SET_BACKGROUND_COLOR((byte) 48),
/**
* Default background color: implementation defined (according to standard)
*/
DEFAULT_BACKGROUND_COLOR((byte) 49),
/**
* Framed
*/
FRAMED((byte) 51),
/**
* Encircled
*/
ENCIRCLED((byte) 52),
/**
* Overlined
*/
OVERLINED((byte) 53),
/**
* Not framed or encircled
*/
NOT_FRAMED((byte) 54),
/**
* Not overlined
*/
NOT_OVERLINED((byte) 55),
/**
* Ideogram underline or right side line: Rarely supported.
*/
IDEOGRAM_UNDERLINE_OR_RIGHT_SIDE_LINE((byte) 60),
/**
* Ideogram double underline or double line on the right side : Rarely
* supported.
*/
IDEOGRAM_DOUBLE_UNDERLINE_OR_DOUBLE_LINE_ON_THE_RIGHT_SIDE((byte) 61),
/**
* Ideogram overline or left side line : Rarely supported.
*/
IDEOGRAM_OVERLINE_OR_LEFT_SIDE_LINE((byte) 62),
/**
* Ideogram double overline or double line on the left side : Rarely
* supported.
*/
IDEOGRAM_DOUBLE_OVERLINE_OR_DOUBLE_LINE_ON_THE_LEFT_SIDE((byte) 63),
/**
* Ideogram stress marking : Rarely supported.
*/
IDEOGRAM_STRESS_MARKING((byte) 64),
/**
* Ideogram attributes off: Reset the effects of all of 60–64.
*/
IDEOGRAM_ATTRIBUTES_OFF((byte) 65),
/**
* Foreground Bright Black: Set bright foreground color (aixterm (not in
* standard)).
*/
FG_BRIGHT_BLACK((byte) 90),
/**
* Foreground Bright Red: Set bright foreground color (aixterm (not in
* standard)).
*/
FG_BRIGHT_RED((byte) 91),
/**
* Foreground Bright Green: Set bright foreground color (aixterm (not in
* standard)).
*/
FG_BRIGHT_GREEN((byte) 92),
/**
* Foreground Bright Yellow: Set bright foreground color (aixterm (not in
* standard)).
*/
FG_BRIGHT_YELLOW((byte) 93),
/**
* Foreground Bright Blue: Set bright foreground color (aixterm (not in
* standard)).
*/
FG_BRIGHT_BLUE((byte) 94),
/**
* Foreground Bright Magenta: Set bright foreground color (aixterm (not in
* standard)).
*/
FG_BRIGHT_MAGENTA((byte) 95),
/**
* Foreground Bright Cyan: Set bright foreground color (aixterm (not in
* standard)).
*/
FG_BRIGHT_CYAN((byte) 96),
/**
* Foreground Bright White: Set bright foreground color (aixterm (not in
* standard)).
*/
FG_BRIGHT_WHITE((byte) 97),
/**
* Foreground Bright Default: Set bright foreground color (aixterm (not in
* standard)).
*/
FG_BRIGHT_DEFAULT((byte) 99),
/**
* Background Bright Black: Set bright background color (aixterm (not in
* standard)).
*/
BG_BRIGHT_BLACK((byte) 100),
/**
* Background Bright Red: Set bright background color (aixterm (not in
* standard)).
*/
BG_BRIGHT_RED((byte) 101),
/**
* Background Bright Green: Set bright background color (aixterm (not in
* standard)).
*/
BG_BRIGHT_GREEN((byte) 102),
/**
* Background Bright Yellow: Set bright background color (aixterm (not in
* standard)).
*/
BG_BRIGHT_YELLOW((byte) 103),
/**
* Background Bright Blue: Set bright background color (aixterm (not in
* standard)).
*/
BG_BRIGHT_BLUE((byte) 104),
/**
* Background Bright Magenta: Set bright background color (aixterm (not in
* standard)).
*/
BG_BRIGHT_MAGENTA((byte) 105),
/**
* Background Bright Cyan: Set bright background color (aixterm (not in
* standard)).
*/
BG_BRIGHT_CYAN((byte) 106),
/**
* Background Bright White: Set bright background color (aixterm (not in
* standard)).
*/
BG_BRIGHT_WHITE((byte) 107),
/**
* Background Bright Default: Set bright background color (aixterm (not in
* standard)).
*/
BG_BRIGHT_DEFAULT((byte) 109);
// /////////////////////////////////////////////////////////////////////////
// CONSTANTS:
// /////////////////////////////////////////////////////////////////////////
private static String REGEX = "\u001B\\[[;\\d]*m";
private static Pattern PATTERN = Pattern.compile( REGEX );
/**
* The ANSI Escape-Code with which each ANSI escape sequence is commenced.
*/
public static char ESCAPE = '\u001B';
/**
* The ANSI Escape-Code with which each ANSI escape sequence is concluded.
*/
public static char ESCAPE_SUFFIX = 'm';
/**
* The delimiter used to separate the parameters of an ANSI Escape-Sequence,
* e.g. the parameters "0", "1" and ""4" of the sequence
* "<ESC>[0;1;4m
".
*/
public static char ESCAPE_PARAMETER_DELIMITER = Delimiter.ANSI_ESCAPE_PARAMETER.getChar();
/**
* The delimiter used to separate the leading Escape-Code <ESC> of an
* ANSI Escape-Sequence from the succeeding portion, e.g. "[" separates
* <ESC> from "[0;1;4m" of the sequence
* "<ESC>[0;1;4m
".
*/
public static char ESCAPE_PREFIX_DELIMITER = Delimiter.ANSI_ESCAPE_PREFIX.getChar();
/**
* In case of {@link #SET_FOREGROUND_COLOR} or
* {@link #SET_BACKGROUND_COLOR}, the {@link #RGB_COLOR_SELECTOR_PARAMETER}
* specifies that the succeeding parameters represent RGB values.
*/
public static char RGB_COLOR_SELECTOR_PARAMETER = 2;
/**
* In case of {@link #SET_FOREGROUND_COLOR} or
* {@link #SET_BACKGROUND_COLOR}, the
* {@link #COLOR_TABLE_SELECTOR_PARAMETER} specifies that the succeeding
* parameter represents an index into the ANSI Color-Table.
*/
public static char COLOR_TABLE_SELECTOR_PARAMETER = 5;
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private byte _ansiEscapeCode;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Instantiates a new ansi escape code.
*
* @param aAnsiEscapeCode the ansi escape code
*/
private AnsiEscapeCode( byte aAnsiEscapeCode ) {
_ansiEscapeCode = aAnsiEscapeCode;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public Byte getCode() {
return _ansiEscapeCode;
}
/**
* Returns the plain ANSI escape sequence for this {@link AnsiEscapeCode}
* element. Use {@link #toEscapeSequence(AnsiEscapeCode...)} if you require
* an ANSI escape sequence consisting of more than just one
* {@link AnsiEscapeCode}.
*
* @return The correctly generated Escape-Code sequence directly printable
* to {@link System#out}.
*/
public String toEscapeSequence() {
return toEscapeSequence( this );
}
/**
* Basic functionality to create an ANSI Escape-Code sequence from the
* provided {@link AnsiEscapeCode} elements directly printable to
* {@link System#out}. This method can only provide placeholder such as
* ${n0}, ${n1}, ... for elements {@link #SET_FOREGROUND_COLOR} and
* {@link #SET_BACKGROUND_COLOR} (the numbers correspond to the order of
* their appearance). Consider building your sequence manually in case you
* want to use a RGB value instead of an index into the ANSI Color-Table.
*
* @param aAnsiEscapeCodes The Escape-Codes to be included in the
* Escape-Code sequence.
*
* @return The correctly generated Escape-Code sequence directly printable
* to {@link System#out}.
*/
public static String toEscapeSequence( AnsiEscapeCode... aAnsiEscapeCodes ) {
int n = 0;
final StringBuilder theSequence = new StringBuilder();
AnsiEscapeCode eCode;
theSequence.append( ESCAPE );
theSequence.append( ESCAPE_PREFIX_DELIMITER );
for ( int i = 0; i < aAnsiEscapeCodes.length; i++ ) {
eCode = aAnsiEscapeCodes[i];
theSequence.append( eCode.getCode() );
if ( eCode == SET_FOREGROUND_COLOR || eCode == SET_BACKGROUND_COLOR ) {
appendColorTableIndex( n, theSequence );
n++;
}
if ( i < aAnsiEscapeCodes.length - 1 ) {
theSequence.append( ESCAPE_PARAMETER_DELIMITER );
}
}
theSequence.append( ESCAPE_SUFFIX );
return theSequence.toString();
}
/**
* Makes the provided Escape-Sequence printable by replacing the
* {@link #ESCAPE} code with a printable char sequence. This means that the
* Escape-Sequence is disabled so that it will be printed out as plain text.
*
* @param aEscapeSequence The Escape-Sequence to be printed out as plain
* text.
*
* @return The plain text representation of the given Escape-Sequence.
*/
public static String toEscapedSequence( String aEscapeSequence ) {
return aEscapeSequence.replaceAll( ESCAPE + "", "\\u001B" );
}
/**
* Retrieves a list of regions (begin index, end index ) with ANSI escape
* codes. The begin index is being included and the end index is being
* excluded by the returned regions.
*
* @param aEscapedString The {@link String} for which to find the
* {@link Region} elements containing ANSI escape codes.
*
* @return The list of regions with ANSI escape codes.
*/
public static Region[] toEscapedSequenceRegions( String aEscapedString ) {
final Matcher theMatcher = PATTERN.matcher( aEscapedString );
final List theRegions = new ArrayList<>();
while ( theMatcher.find() ) {
theRegions.add( new Region( theMatcher.start(), theMatcher.end() ) );
}
return theRegions.toArray( new Region[theRegions.size()] );
}
/**
* Removes any ANSI escape codes from the provided {@link String}.
*
* @param aEscapedString The (potentially) ANSI escaped {@link String}.
*
* @return The {@link String} without any ANSI escape codes.
*/
public static String toUnescapedString( String aEscapedString ) {
return aEscapedString.replaceAll( REGEX, "" );
}
/**
* Returns the effective length of the given {@link String} by ignoring any
* ANSI escape codes contained within the provided {@link String}.
*
* @param aEscapedString The (potentially) ANSI escaped {@link String}.
*
* @return The length of the {@link String} without any ANSI escape codes
* being counted.
*/
public static int toUnescapedLength( String aEscapedString ) {
return aEscapedString.replaceAll( REGEX, "" ).length();
}
/**
* Returns the length of the text, considering whether the text is expected
* to have ANSI escape codes not considered to be part of the length.
*
* @param aText The text for which to get the length.
* @param hasAnsiEscapeCodes Whether to take any (non printable) ANSI escape
* codes into account which would, when not being considered, cause
* wrong results.
*
* @return The according print length.
*/
public static int toLength( String aText, boolean hasAnsiEscapeCodes ) {
if ( hasAnsiEscapeCodes ) {
return toUnescapedLength( aText );
}
return aText.length();
}
/**
* Returns the escaped ANSI Escape-Code to be printable without triggering
* the actual ANSI escape commands.
*
* @return The escaped ANSI Escape-Code.
*/
public String toEscaped() {
return ( _ansiEscapeCode + "" ).replaceAll( ESCAPE + "", "\\u001B" );
}
// /////////////////////////////////////////////////////////////////////////
// HELPER:
// /////////////////////////////////////////////////////////////////////////
private static void appendColorTableIndex( int aColorPaletteIndex, StringBuilder aSequence ) {
aSequence.append( ESCAPE_PARAMETER_DELIMITER );
aSequence.append( COLOR_TABLE_SELECTOR_PARAMETER );
aSequence.append( ESCAPE_PARAMETER_DELIMITER );
aSequence.append( "${n" );
aSequence.append( aColorPaletteIndex );
aSequence.append( "}" );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy