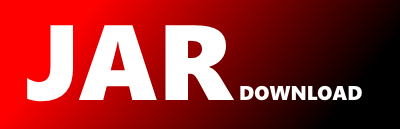
org.refcodes.data.EscapeCode Maven / Gradle / Ivy
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.data;
/**
* The {@link EscapeCode} enumeration contains all of Java's escape codes
* (https://docs.oracle.com/javase/tutorial/java/data/characters.html) and the
* escape sequences (being the defused escape codes) as well as methods to
* retrieve the escape code from the escape sequence (as of
* {@link #toEscapeCode(String)}) and vice versa (as of
* {@link #toEscapeCode(char)}).
*/
public enum EscapeCode {
TAB('\t', "\\t"),
BACKSPACE('\b', "\\b"),
NEWLINE('\n', "\\n"),
CARRIAGE_RETURN('\r', "\\r"),
FORM_FEED('\f', "\\f"),
SINGLE_QUOTE('\'', "\\'"),
DOUBLE_QUOTE('\"', "\\\""),
BASCKSLASH('\\', "\\\\");
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private char _escapeCode;
private String _escapeSequence;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
private EscapeCode( char aEscapeChar, String aEscapedSequence ) {
_escapeCode = aEscapeChar;
_escapeSequence = aEscapedSequence;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* Retrieves the escape sequence {@link String} for the according
* {@link EscapeCode} being the escaped escape code.
*
* @return The according escape sequence.
*/
public String getEscapeSequence() {
return _escapeSequence;
}
/**
* Retrieves the escape code character for the according {@link EscapeCode}.
*
* @return The according escape code.
*/
public char getEscapeCode() {
return _escapeCode;
}
/**
* Translate the provided {@link EscapeCode} character to the according
* escape sequence.
*
* @param aEscapeCode The escape code character to translate to the
* according {@link EscapeCode} element.
*
* @return The according {@link EscapeCode} or null if the provided escape
* code did not represent an escape code as of the
* {@link EscapeCode} enumeration.
*/
public static EscapeCode toEscapeCode( char aEscapeCode ) {
for ( EscapeCode eCode : values() ) {
if ( eCode.getEscapeCode() == aEscapeCode ) {
return eCode;
}
}
return null;
}
/**
* Translate the provided escape sequence to the according
* {@link EscapeCode} element.
*
* @param aEscapeSequence The escape sequence to translate to the according
* {@link EscapeCode} element.
*
* @return The according {@link EscapeCode} or null if the provided escape
* code did not represent an escape code as of the
* {@link EscapeCode} enumeration.
*/
public static EscapeCode toEscapeCode( String aEscapeSequence ) {
for ( EscapeCode eCode : values() ) {
if ( eCode.getEscapeSequence().equals( aEscapeSequence ) ) {
return eCode;
}
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy