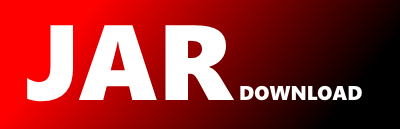
org.refcodes.data.FilenameExtension Maven / Gradle / Ivy
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// =============================================================================
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// =============================================================================
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// together with the GPL linking exception applied; as being applied by the GNU
// Classpath ("http://www.gnu.org/software/classpath/license.html")
// =============================================================================
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// =============================================================================
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.data;
import org.refcodes.mixin.FilenameExtensionAccessor;
import org.refcodes.mixin.FilenameSuffixAccessor;
/**
* The {@link FilenameExtension} define values useful when working with files or
* a file-system.
*/
public enum FilenameExtension implements FilenameExtensionAccessor, FilenameSuffixAccessor {
TEMP("tmp"),
BACKUP("bak"),
TAR("tar"),
GZIP("gz"),
TAR_GZIP("tgz"),
CSV("csv"),
TXT("txt"),
JAR("jar"),
ZIP("zip"),
SH("sh"),
/**
* The file suffix used for files containing a single cipher version.
*/
CIPHER_VERSION("cv"),
PROPERTIES("properties"),
JSON("json"),
TOML("toml"),
YAML("yaml"),
XML("xml"),
/**
* Windows configuration filename extension.
*/
INI("ini"),
/**
* BASE64 encoded data.
*/
BASE64("base64"),
/**
* Chaos encrypted and base 64 encoded data.
*/
CHAOS64("chaos64"),
/**
* Chaos encrypted encoded data.
*/
CHAOS("chaos"),
TIFF("tiff"),
GIF("tiff"),
PNG("png"),
BMP("bmp"),
JPG("bmp");
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private String _extension;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Instantiates a new file name extension.
*
* @param aExtension the extension
*/
private FilenameExtension( String aExtension ) {
_extension = aExtension;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* A filename suffix included the prefixed dot (".") of the file name
* filename extension. Gets the extension with a prefixed dot (".") for
* directly appending it to a base filename.
*
* @return the extension including a prefixed dot (".");
*/
@Override
public String getFilenameSuffix() {
return Delimiter.FILENAME_EXTENSION.getChar() + _extension;
}
/**
* Retrieves the filename extension. A filename extensions does not(!)
* contain the dot "." separating the extension from the name, e.g. "ini"
* (instead of ".ini").
*
* @return The filename extension stored by the filename extension property.
*/
@Override
public String getFilenameExtension() {
return _extension;
}
/**
* Determines the filename's extension.
*
* @param aFilename The filename for which to determine the filename
* extension.
*
* @return The filename extension or null if it has none extension.
*/
public static String toRawFileNameExtension( String aFilename ) {
if ( aFilename != null && aFilename.length() != 0 ) {
int index = aFilename.indexOf( Delimiter.FILENAME_EXTENSION.getChar() );
if ( index == 0 ) {
aFilename = aFilename.substring( 1 );
}
index = aFilename.indexOf( Delimiter.FILENAME_EXTENSION.getChar() );
if ( index != -1 ) {
aFilename = aFilename.substring( index + 1 );
while ( ( index = aFilename.indexOf( Delimiter.FILENAME_EXTENSION.getChar() ) ) != -1 ) {
aFilename = aFilename.substring( index + 1 );
}
if ( aFilename.length() != 0 ) {
return aFilename;
}
}
}
return null;
}
/**
* Determines the filename's {@link FilenameExtension} definition.
*
* @param aFilename The filename for which to determine the
* {@link FilenameExtension}.
*
* @return The {@link FilenameExtension} or null if it has none extension.
*/
public static FilenameExtension toFileNameExtension( String aFilename ) {
final String theRawExtension = toRawFileNameExtension( aFilename );
for ( FilenameExtension eExtension : values() ) {
if ( eExtension.getFilenameExtension().equalsIgnoreCase( theRawExtension ) ) {
return eExtension;
}
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy