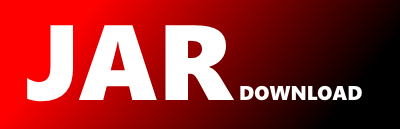
org.refcodes.decoupling.Context Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-decoupling Show documentation
Show all versions of refcodes-decoupling Show documentation
Artifact for breaking up dependencies between components or modules of
a software system (dependency injection and inversion of control).
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.decoupling;
import java.lang.reflect.Array;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashSet;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Set;
import org.refcodes.mixin.Schemable;
/**
* The {@link Context} describes the components and modules wired together by
* the {@link Reactor}.
*/
@SuppressWarnings("rawtypes")
public class Context implements Schemable, DependenciesAccessor {
// /////////////////////////////////////////////////////////////////////////
// STATICS:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// CONSTANTS:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private Dependency>[] _dependencies;
private Object[] _profiles;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Empty constructor to be used in conjunction with the
* {@link #initialize(Dependency[])} method!.
*
* @param aProfiles The profiles which have been applied when creating this
* {@link Context}.
*/
protected Context( Object[] aProfiles ) {
_profiles = aProfiles;
}
/**
* Creates the {@link Context} with the managed {@link Dependency}
* instances.
*
* @param aDependencies The {@link Dependency} declarations of which the
* {@link Context} consists.
* @param aProfiles The profiles which have been applied when creating this
* {@link Context}.
*/
public Context( Dependency>[] aDependencies, Object[] aProfiles ) {
_dependencies = aDependencies;
_profiles = aProfiles;
}
// /////////////////////////////////////////////////////////////////////////
// INJECTION:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* Retrieves the The profiles which have been applied when creating this
* {@link Context}.
*
* @return The according profiles.
*/
public Object[] getProfiles() {
return _profiles;
}
/**
* Retrieves the {@link Dependency} declarations contained in the
* {@link Context}.
*
* @return The {@link Dependency} declarations of which the {@link Context}
* consists.
*/
@Override
public Dependency>[] getDependencies() {
return _dependencies;
}
/**
* Determines the {@link Dependency} element for the given instance created
* from the {@link Dependency}.
*
* @param aInstance The instance which's {@link Dependency} element creating
* this instance is to be retrieved.
*
* @return The according {@link Dependency} element or null if none
* {@link Dependency} element is responsible for crating the
* provided instance.
*/
public Dependency> getDependencyByInstance( Object aInstance ) {
for ( Dependency> eDepenendency : _dependencies ) {
if ( eDepenendency.hasInstance( aInstance ) ) {
return eDepenendency;
}
}
return null;
}
/**
* Retrieves the {@link Dependency} declaration with the given alias managed
* by this {@link Context}, derived from the {@link Dependency}
* {@link Dependency} declaration from which the {@link Reactor} created
* this {@link Context}.
*
* @param aAlias The alias of {@link Dependency} declaration to retrieve.
*
* @return The according {@link Dependency} declaration managed by this
* {@link Context}.
*/
public Dependency> getDependencyByAlias( String aAlias ) {
if ( aAlias != null ) {
for ( Dependency> eDependency : _dependencies ) {
if ( aAlias.equals( eDependency.getAlias() ) ) return eDependency;
}
}
return null;
}
/**
* Retrieves the {@link Dependency} declarations of the given type managed
* by this {@link Context}, derived from the {@link Dependency} declarations
* from which the {@link Reactor} created this {@link Context}.
*
* @param the generic type
* @param aType The type of {@link Dependency} declarations to retrieve.
*
* @return The according {@link Dependency} declarations managed by this
* {@link Context}.
*/
@SuppressWarnings("unchecked")
public Dependency[] getDependenciesByType( Class aType ) {
List> theDependnecies = new ArrayList<>();
for ( Dependency> eDependency : _dependencies ) {
if ( aType.isAssignableFrom( eDependency.getType() ) ) {
theDependnecies.add( (Dependency) eDependency );
}
}
return theDependnecies.toArray( (Dependency[]) Array.newInstance( Dependency.class, theDependnecies.size() ) );
}
/**
* Retrieves the {@link Dependency} declarations of given tags managed by
* this {@link Context}, derived from the {@link Dependency}
* {@link Dependency} declarations from which the {@link Reactor} created
* this {@link Context}.
*
* @param aTags The tags of the {@link Dependency} declarations to retrieve.
*
* @return The according {@link Dependency} declarations managed by this
* {@link Context}.
*/
public Dependency>[] getDependenciesByTags( Object... aTags ) {
List> theDependencies = new ArrayList<>();
for ( Dependency> eDependency : _dependencies ) {
out: {
for ( Object eTag : aTags ) {
for ( Object eDependencyTag : eDependency.getTags() ) {
if ( eTag.toString().equalsIgnoreCase( eDependencyTag.toString() ) ) {
theDependencies.add( eDependency );
break out;
}
}
}
}
}
return theDependencies.toArray( new Dependency>[theDependencies.size()] );
}
/**
* Retrieves the {@link Dependency} declarations of given profiles managed
* by this {@link Context}, derived from the {@link Dependency}
* {@link Dependency} declarations from which the {@link Reactor} created
* this {@link Context}.
*
* @param aProfiles The profiles of the {@link Dependency} declarations to
* retrieve.
*
* @return The according {@link Dependency} declarations managed by this
* {@link Context}.
*/
public Dependency>[] getDependenciesByProfiles( Object... aProfiles ) {
List> theDependencies = new ArrayList<>();
for ( Dependency> eDependency : _dependencies ) {
out: {
for ( Object eProfile : aProfiles ) {
for ( Object eDependencyProfile : eDependency.getProfiles() ) {
if ( eProfile.toString().equalsIgnoreCase( eDependencyProfile.toString() ) ) {
theDependencies.add( eDependency );
break out;
}
}
}
}
}
return theDependencies.toArray( new Dependency>[theDependencies.size()] );
}
/**
* Retrieves the instances managed by this {@link Context}, derived from the
* {@link Dependency} declarations from which the {@link Reactor} created
* this {@link Context}.
*
* @return The instances managed by this {@link Context}.
*/
public Object[] getInstances() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy