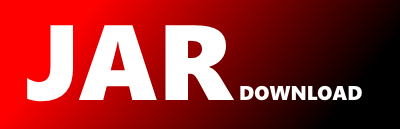
org.refcodes.decoupling.Claim Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-decoupling Show documentation
Show all versions of refcodes-decoupling Show documentation
Artifact for breaking up dependencies between components or modules of
a software system (dependency injection and inversion of control).
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.decoupling;
import java.util.Objects;
import org.refcodes.mixin.AliasAccessor;
import org.refcodes.mixin.Schemable;
import org.refcodes.mixin.TypeAccessor;
/**
* A claim describes a {@link Claim} required by a {@link Dependency}.
*/
@SuppressWarnings("rawtypes")
public class Claim implements AliasAccessor, TypeAccessor, Schemable, Comparable {
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
protected String _alias;
protected Class> _type;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Instantiates a new {@link Claim}.
*/
protected Claim() {}
/**
* Constructs a {@link Claim} for the given type (the alias is, if not
* provided, derived from type).
*
* @param aType The type of the {@link Claim}.
*/
public Claim( Class> aType ) {
this( aType, null );
}
/**
* Constructs a {@link Claim} for the given type (the alias is, if not
* provided, derived from type).
*
* @param aAlias The alias for the {@link Claim}.
*/
public Claim( String aAlias ) {
this( null, aAlias );
}
/**
* Constructs a {@link Claim} for the given type (the alias is, if not
* provided, derived from type).
*
* @param aType The type of the {@link Claim}.
* @param aAlias The alias for the {@link Claim}.
*/
public Claim( Class> aType, String aAlias ) {
_alias = aAlias;
_type = aType;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public String getAlias() {
return _alias;
}
/**
* {@inheritDoc}
*/
@Override
public Class getType() {
return _type;
}
/**
* Determines whether the given {@link Dependency} declaration matches this
* {@link Claim} instance.
*
* @param aDependency The {@link Dependency} declaration which to match
* against this {@link Claim} instance.
*
* @return True in case the {@link Claim} instance can be satisfied by the
* given {@link Dependency} declaration.
*/
public boolean isClaim( Dependency> aDependency ) {
if ( _alias != null && !_alias.equalsIgnoreCase( aDependency.getAlias() ) ) {
return false;
}
if ( _type != null && !_type.isAssignableFrom( aDependency.getType() ) ) {
return false;
}
return true;
}
/**
* {@inheritDoc}
*/
@Override
public DependencySchema toSchema() {
return new DependencySchema( this );
}
/**
* {@inheritDoc}
*/
@Override
public int compareTo( Claim aClaim ) {
final String thisAlias = _alias != null ? _alias : "";
final String thatAlias = aClaim != null && aClaim.getAlias() != null ? aClaim.getAlias() : "";
return thisAlias.compareTo( thatAlias );
}
/**
* {@inheritDoc}
*/
@Override
public int hashCode() {
return Objects.hash( _alias, _type );
}
/**
* {@inheritDoc}
*/
@Override
public boolean equals( Object obj ) {
if ( this == obj ) {
return true;
}
if ( obj == null ) {
return false;
}
if ( getClass() != obj.getClass() ) {
return false;
}
final Claim other = (Claim) obj;
return Objects.equals( _alias, other._alias ) && Objects.equals( _type, other._type );
}
/**
* {@inheritDoc}
*/
@Override
public String toString() {
return getClass().getSimpleName() + " [alias=" + _alias + ", type=" + _type + "]";
}
// /////////////////////////////////////////////////////////////////////////
// HOOKS:
// /////////////////////////////////////////////////////////////////////////
/**
* Sets the alias.
*
* @param aAlias The alias to be set.
*/
void setAlias( String aAlias ) {
_alias = aAlias;
}
/**
* Sets the type.
*
* @param aType The type to be set.
*/
void setType( Class> aType ) {
_type = aType;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy