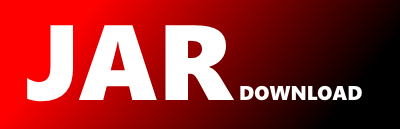
org.refcodes.decoupling.DependencyException Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-decoupling Show documentation
Show all versions of refcodes-decoupling Show documentation
Artifact for breaking up dependencies between components or modules of
a software system (dependency injection and inversion of control).
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// =============================================================================
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// =============================================================================
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// together with the GPL linking exception applied; as being applied by the GNU
// Classpath ("http://www.gnu.org/software/classpath/license.html")
// =============================================================================
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// =============================================================================
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.decoupling;
import org.refcodes.exception.AbstractException;
/**
* The {@link DependencyException} is the base checked exception for the
* refcodes-decoupling
package.
*/
@SuppressWarnings("rawtypes")
public abstract class DependencyException extends AbstractException implements DependencyAccessor {
private static final long serialVersionUID = 1L;
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
protected Dependency> _dependency;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*
* @param aDependency The related {@link Dependency}.
*/
public DependencyException( String aMessage, Dependency> aDependency, String aErrorCode ) {
super( aMessage, aErrorCode );
_dependency = aDependency;
}
/**
* {@inheritDoc}
*
* @param aDependency The related {@link Dependency}.
*/
public DependencyException( String aMessage, Dependency> aDependency, Throwable aCause, String aErrorCode ) {
super( aMessage, aCause, aErrorCode );
_dependency = aDependency;
}
/**
* {@inheritDoc}
*
* @param aDependency The related {@link Dependency}.
*/
public DependencyException( String aMessage, Dependency> aDependency, Throwable aCause ) {
super( aMessage, aCause );
_dependency = aDependency;
}
/**
* {@inheritDoc}
*
* @param aDependency The related {@link Dependency}.
*/
public DependencyException( String aMessage, Dependency> aDependency ) {
super( aMessage );
_dependency = aDependency;
}
/**
* {@inheritDoc}
*
* @param aDependency The related {@link Dependency}.
*/
public DependencyException( Dependency> aDependency, Throwable aCause, String aErrorCode ) {
super( aCause, aErrorCode );
_dependency = aDependency;
}
/**
* {@inheritDoc}
*
* @param aDependency The related {@link Dependency}.
*/
public DependencyException( Dependency> aDependency, Throwable aCause ) {
super( aCause );
_dependency = aDependency;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public Dependency> getDependency() {
return _dependency;
}
/**
* {@inheritDoc}
*/
@Override
public Object[] getPatternArguments() {
return new Object[] { _dependency };
}
// /////////////////////////////////////////////////////////////////////////
// INNER CLASSES:
// /////////////////////////////////////////////////////////////////////////
/**
* The {@link DependenciesException} is a {@link DependencyException}
* containing additional related dependency.
*/
protected abstract static class DependenciesException extends DependencyException implements DependenciesAccessor {
// /////////////////////////////////////////////////////////////////////
// STATICS:
// /////////////////////////////////////////////////////////////////////
private static final long serialVersionUID = 1L;
// /////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////
protected Dependency>[] _dependencies;
// /////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*
* @param aDependencies the {@link Dependency} declarations related to
* this exception.
*/
public DependenciesException( String aMessage, Dependency> aDependency, Dependency>[] aDependencies, String aErrorCode ) {
super( aMessage, aDependency, aErrorCode );
_dependencies = aDependencies;
}
/**
* {@inheritDoc}
*
* @param aDependencies the {@link Dependency} declarations related to
* this exception.
*/
public DependenciesException( String aMessage, Dependency> aDependency, Dependency>[] aDependencies, Throwable aCause, String aErrorCode ) {
super( aMessage, aDependency, aCause, aErrorCode );
_dependencies = aDependencies;
}
/**
* {@inheritDoc}
*
* @param aDependencies the {@link Dependency} declarations related to
* this exception.
*/
public DependenciesException( String aMessage, Dependency> aDependency, Dependency>[] aDependencies, Throwable aCause ) {
super( aMessage, aDependency, aCause );
_dependencies = aDependencies;
}
/**
* {@inheritDoc}
*
* @param aDependencies the {@link Dependency} declarations related to
* this exception.
*/
public DependenciesException( String aMessage, Dependency> aDependency, Dependency>[] aDependencies ) {
super( aMessage, aDependency );
_dependencies = aDependencies;
}
/**
* {@inheritDoc}
*
* @param aDependencies the {@link Dependency} declarations related to
* this exception.
*/
public DependenciesException( Dependency> aDependency, Dependency>[] aDependencies, Throwable aCause, String aErrorCode ) {
super( aDependency, aCause, aErrorCode );
_dependencies = aDependencies;
}
/**
* {@inheritDoc}
*
* @param aDependencies the {@link Dependency} declarations related to
* this exception.
*/
public DependenciesException( Dependency> aDependency, Dependency>[] aDependencies, Throwable aCause ) {
super( aDependency, aCause );
_dependencies = aDependencies;
}
// /////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public Dependency>[] getDependencies() {
return _dependencies;
}
/**
* {@inheritDoc}
*/
@Override
public Object[] getPatternArguments() {
return new Object[] { _dependency, _dependencies };
}
}
/**
* The {@link DependencyClaimException} is a {@link DependencyException}
* containing an additional related {@link Claim} instance relative to
* associated {@link Claim} instances.
*/
protected abstract static class DependencyClaimException extends DependencyException implements ClaimAccessor, ClaimsAccessor {
// /////////////////////////////////////////////////////////////////////
// STATICS:
// /////////////////////////////////////////////////////////////////////
private static final long serialVersionUID = 1L;
// /////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////
protected Claim[] _claims;
protected Claim _claim;
// /////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*
* @param aClaim the {@link Claim} instance responsible for this
* exception.
* @param aClaims the {@link Claim} instances related to this exception.
*/
public DependencyClaimException( String aMessage, Claim aClaim, Claim[] aClaims, Dependency> aDependency, String aErrorCode ) {
super( aMessage, aDependency, aErrorCode );
_claim = aClaim;
_claims = aClaims;
}
/**
* {@inheritDoc}
*
* @param aClaim the {@link Claim} instance responsible for this
* exception.
* @param aClaims the {@link Claim} instances related to this exception.
*/
public DependencyClaimException( String aMessage, Claim aClaim, Claim[] aClaims, Dependency> aDependency, Throwable aCause, String aErrorCode ) {
super( aMessage, aDependency, aCause, aErrorCode );
_claim = aClaim;
_claims = aClaims;
}
/**
* {@inheritDoc}
*
* @param aClaim the {@link Claim} instance responsible for this
* exception.
* @param aClaims the {@link Claim} instances related to this exception.
*/
public DependencyClaimException( String aMessage, Claim aClaim, Claim[] aClaims, Dependency> aDependency, Throwable aCause ) {
super( aMessage, aDependency, aCause );
_claim = aClaim;
_claims = aClaims;
}
/**
* {@inheritDoc}
*
* @param aClaim the {@link Claim} instance responsible for this
* exception.
* @param aClaims the {@link Claim} instances related to this exception.
*/
public DependencyClaimException( String aMessage, Claim aClaim, Claim[] aClaims, Dependency> aDependency ) {
super( aMessage, aDependency );
_claim = aClaim;
_claims = aClaims;
}
/**
* {@inheritDoc}
*
* @param aClaim the {@link Claim} instance responsible for this
* exception.
* @param aClaims the {@link Claim} instances related to this exception.
*/
public DependencyClaimException( Claim aClaim, Claim[] aClaims, Dependency> aDependency, Throwable aCause, String aErrorCode ) {
super( aDependency, aCause, aErrorCode );
_claim = aClaim;
_claims = aClaims;
}
/**
* {@inheritDoc}
*
* @param aClaim the {@link Claim} instance responsible for this
* exception.
* @param aClaims the {@link Claim} instances related to this exception.
*/
public DependencyClaimException( Claim aClaim, Claim[] aClaims, Dependency> aDependency, Throwable aCause ) {
super( aDependency, aCause );
_claim = aClaim;
_claims = aClaims;
}
// /////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public Claim[] getClaims() {
return _claims;
}
/**
* {@inheritDoc}
*/
@Override
public Claim getClaim() {
return _claim;
}
/**
* {@inheritDoc}
*/
@Override
public Object[] getPatternArguments() {
return new Object[] { _claim, _claims, _dependency };
}
}
/**
* The {@link ClaimDependenciesException} is a {@link DependenciesException}
* containing an additional related {@link Claim} instance.
*/
protected static class ClaimDependenciesException extends DependenciesException implements ClaimAccessor {
// /////////////////////////////////////////////////////////////////////
// STATICS:
// /////////////////////////////////////////////////////////////////////
private static final long serialVersionUID = 1L;
// /////////////////////////////////////////////////////////////////////
// CONSTANTS:
// /////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////
protected Claim _claim;
// /////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*
* @param aClaim the {@link Claim} instance responsible for this
* exception.
*/
public ClaimDependenciesException( String aMessage, Claim aClaim, Dependency> aDependency, Dependency>[] aDependencies, String aErrorCode ) {
super( aMessage, aDependency, aDependencies, aErrorCode );
_claim = aClaim;
}
/**
* {@inheritDoc}
*
* @param aClaim the {@link Claim} instance responsible for this
* exception.
*/
public ClaimDependenciesException( String aMessage, Claim aClaim, Dependency> aDependency, Dependency>[] aDependencies, Throwable aCause, String aErrorCode ) {
super( aMessage, aDependency, aDependencies, aCause, aErrorCode );
_claim = aClaim;
}
/**
* {@inheritDoc}
*
* @param aClaim the {@link Claim} instance responsible for this
* exception.
*/
public ClaimDependenciesException( String aMessage, Claim aClaim, Dependency> aDependency, Dependency>[] aDependencies, Throwable aCause ) {
super( aMessage, aDependency, aDependencies, aCause );
_claim = aClaim;
}
/**
* {@inheritDoc}
*
* @param aClaim the {@link Claim} instance responsible for this
* exception.
*/
public ClaimDependenciesException( String aMessage, Claim aClaim, Dependency> aDependency, Dependency>[] aDependencies ) {
super( aMessage, aDependency, aDependencies );
_claim = aClaim;
}
/**
* {@inheritDoc}
*
* @param aClaim the {@link Claim} instance responsible for this
* exception.
*/
public ClaimDependenciesException( Claim aClaim, Dependency> aDependency, Dependency>[] aDependencies, Throwable aCause, String aErrorCode ) {
super( aDependency, aDependencies, aCause, aErrorCode );
_claim = aClaim;
}
/**
* {@inheritDoc}
*
* @param aClaim the {@link Claim} instance responsible for this
* exception.
*/
public ClaimDependenciesException( Claim aClaim, Dependency> aDependency, Dependency>[] aDependencies, Throwable aCause ) {
super( aDependency, aDependencies, aCause );
_claim = aClaim;
}
// /////////////////////////////////////////////////////////////////////
// INJECTION:
// /////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////
/**
* Retrieves the {@link Claim} instance responsible for this exception.
*
* @return The according {@link Claim} instance.
*/
@Override
public Claim getClaim() {
return _claim;
}
/**
* {@inheritDoc}
*/
@Override
public Object[] getPatternArguments() {
return new Object[] { _claim, _dependency, _dependencies };
}
// /////////////////////////////////////////////////////////////////////
// HOOKS:
// /////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////
// HELPER:
// /////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////
// INNER CLASSES:
// /////////////////////////////////////////////////////////////////////
}
/**
* The {@link InitializerDependenciesException} is a
* {@link DependenciesException} containing an additional related
* {@link InitializerClaim} instance.
*/
protected static class InitializerDependenciesException extends DependenciesException {
// /////////////////////////////////////////////////////////////////////
// STATICS:
// /////////////////////////////////////////////////////////////////////
private static final long serialVersionUID = 1L;
// /////////////////////////////////////////////////////////////////////
// CONSTANTS:
// /////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////
protected InitializerClaim, ?> _initializerClaim;
// /////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*
* @param aInitializerClaim the {@link InitializerClaim} instance
* responsible for this exception.
*/
public InitializerDependenciesException( String aMessage, InitializerClaim aInitializerClaim, Dependency> aDependency, Dependency>[] aDependencies, String aErrorCode ) {
super( aMessage, aDependency, aDependencies, aErrorCode );
_initializerClaim = aInitializerClaim;
}
/**
* {@inheritDoc}
*
* @param aInitializerClaim the {@link InitializerClaim} instance
* responsible for this exception.
*/
public InitializerDependenciesException( String aMessage, InitializerClaim aInitializerClaim, Dependency> aDependency, Dependency>[] aDependencies, Throwable aCause, String aErrorCode ) {
super( aMessage, aDependency, aDependencies, aCause, aErrorCode );
_initializerClaim = aInitializerClaim;
}
/**
* {@inheritDoc}
*
* @param aInitializerClaim the {@link InitializerClaim} instance
* responsible for this exception.
*/
public InitializerDependenciesException( String aMessage, InitializerClaim aInitializerClaim, Dependency> aDependency, Dependency>[] aDependencies, Throwable aCause ) {
super( aMessage, aDependency, aDependencies, aCause );
_initializerClaim = aInitializerClaim;
}
/**
* {@inheritDoc}
*
* @param aInitializerClaim the {@link InitializerClaim} instance
* responsible for this exception.
*/
public InitializerDependenciesException( String aMessage, InitializerClaim aInitializerClaim, Dependency> aDependency, Dependency>[] aDependencies ) {
super( aMessage, aDependency, aDependencies );
_initializerClaim = aInitializerClaim;
}
/**
* {@inheritDoc}
*
* @param aInitializerClaim the {@link InitializerClaim} instance
* responsible for this exception.
*/
public InitializerDependenciesException( InitializerClaim aInitializerClaim, Dependency> aDependency, Dependency>[] aDependencies, Throwable aCause, String aErrorCode ) {
super( aDependency, aDependencies, aCause, aErrorCode );
_initializerClaim = aInitializerClaim;
}
/**
* {@inheritDoc}
*
* @param aInitializerClaim the {@link InitializerClaim} instance
* responsible for this exception. s
*/
public InitializerDependenciesException( InitializerClaim aInitializerClaim, Dependency> aDependency, Dependency>[] aDependencies, Throwable aCause ) {
super( aDependency, aDependencies, aCause );
_initializerClaim = aInitializerClaim;
}
// /////////////////////////////////////////////////////////////////////
// INJECTION:
// /////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////
/**
* Retrieves the {@link InitializerClaim} instance responsible for this
* exception.
*
* @return The according {@link InitializerClaim} instance.
*/
public InitializerClaim, ?> getInitializer() {
return _initializerClaim;
}
/**
* {@inheritDoc}
*/
@Override
public Object[] getPatternArguments() {
return new Object[] { _initializerClaim, _dependency, _dependencies };
}
// /////////////////////////////////////////////////////////////////////
// HOOKS:
// /////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////
// HELPER:
// /////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////
// INNER CLASSES:
// /////////////////////////////////////////////////////////////////////
}
/**
* The {@link FactoryDependenciesException} is a
* {@link DependenciesException} containing an additional related
* {@link FactoryClaim} instance.
*/
protected static class FactoryDependenciesException extends DependenciesException {
// /////////////////////////////////////////////////////////////////////
// STATICS:
// /////////////////////////////////////////////////////////////////////
private static final long serialVersionUID = 1L;
// /////////////////////////////////////////////////////////////////////
// CONSTANTS:
// /////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////
protected FactoryClaim, ?> _factoryClaim;
// /////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*
* @param aFactoryClaim the {@link FactoryClaim} instance responsible
* for this exception.
*/
public FactoryDependenciesException( String aMessage, FactoryClaim aFactoryClaim, Dependency> aDependency, Dependency>[] aDependencies, String aErrorCode ) {
super( aMessage, aDependency, aDependencies, aErrorCode );
_factoryClaim = aFactoryClaim;
}
/**
* {@inheritDoc}
*
* @param aFactoryClaim the {@link FactoryClaim} instance responsible
* for this exception.
*/
public FactoryDependenciesException( String aMessage, FactoryClaim aFactoryClaim, Dependency> aDependency, Dependency>[] aDependencies, Throwable aCause, String aErrorCode ) {
super( aMessage, aDependency, aDependencies, aCause, aErrorCode );
_factoryClaim = aFactoryClaim;
}
/**
* {@inheritDoc}
*
* @param aFactoryClaim the {@link FactoryClaim} instance responsible
* for this exception.
*/
public FactoryDependenciesException( String aMessage, FactoryClaim aFactoryClaim, Dependency> aDependency, Dependency>[] aDependencies, Throwable aCause ) {
super( aMessage, aDependency, aDependencies, aCause );
_factoryClaim = aFactoryClaim;
}
/**
* {@inheritDoc}
*
* @param aFactoryClaim the {@link FactoryClaim} instance responsible
* for this exception.
*/
public FactoryDependenciesException( String aMessage, FactoryClaim aFactoryClaim, Dependency> aDependency, Dependency>[] aDependencies ) {
super( aMessage, aDependency, aDependencies );
_factoryClaim = aFactoryClaim;
}
/**
* {@inheritDoc}
*
* @param aFactoryClaim the {@link FactoryClaim} instance responsible
* for this exception.
*/
public FactoryDependenciesException( FactoryClaim aFactoryClaim, Dependency> aDependency, Dependency>[] aDependencies, Throwable aCause, String aErrorCode ) {
super( aDependency, aDependencies, aCause, aErrorCode );
_factoryClaim = aFactoryClaim;
}
/**
* {@inheritDoc}
*
* @param aFactoryClaim the {@link FactoryClaim} instance responsible
* for this exception.
*/
public FactoryDependenciesException( FactoryClaim aFactoryClaim, Dependency> aDependency, Dependency>[] aDependencies, Throwable aCause ) {
super( aDependency, aDependencies, aCause );
_factoryClaim = aFactoryClaim;
}
// /////////////////////////////////////////////////////////////////////
// INJECTION:
// /////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////
/**
* Retrieves the {@link FactoryClaim} instance responsible for this
* exception.
*
* @return The according {@link FactoryClaim} instance.
*/
public FactoryClaim, ?> getFactory() {
return _factoryClaim;
}
/**
* {@inheritDoc}
*/
@Override
public Object[] getPatternArguments() {
return new Object[] { _factoryClaim, _dependency, _dependencies };
}
// /////////////////////////////////////////////////////////////////////
// HOOKS:
// /////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////
// HELPER:
// /////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////
// INNER CLASSES:
// /////////////////////////////////////////////////////////////////////
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy