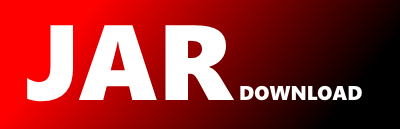
org.refcodes.decoupling.InstanceMode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-decoupling Show documentation
Show all versions of refcodes-decoupling Show documentation
Artifact for breaking up dependencies between components or modules of
a software system (dependency injection and inversion of control).
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.decoupling;
import java.util.HashSet;
import java.util.Set;
/**
* The {@link InstanceMode} describes how an instance for a {@link Dependency}
* is managed (instantiated).
*/
public enum InstanceMode implements InstanceMetrics {
/**
* Technically identical to {@link #SINGLETON_IS_MANDATORY} with emphasizing
* on the semantics of being a default {@link Dependency}, usually used by
* (sub-classes of) the {@link Reactor} type to emphasize that the
* {@link Dependency} is part of the implementation (singleton = yes,
* required = yes).
*/
SINGLETON_BY_DEFAULT(true, true),
/**
* Just a single instance is required and created in any case, not only when
* when demanded by other dependencies, no matter how often the instance is
* being demanded (singleton = yes, required = yes).
*/
SINGLETON_IS_MANDATORY(true, true),
/**
* At least one instance is required and created in any case, not only when
* being demanded, though each time an instance is demanded(!) by other
* dependencies, a new instance is returned (the first time an instance is
* demanded, the initially created instance is injected, singleton = false,
* required = yes).
*/
INSTANCE_IS_MANDATORY(false, true),
/**
* Just a single instance is created on demanded(!) by other dependencies,
* no matter how often the instance is being demanded (singleton = yes,
* required = no).
*/
SINGLETON_ON_DEMAND(true, false),
/**
* Each time an instance is demanded(!) by other dependencies, a new
* instance is returned (singleton = no, required = no).
*/
INSTANCE_ON_DEMAND(false, false);
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private boolean _isSingleton;
private boolean _isMandatory;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
private InstanceMode( boolean isSingleton, boolean isMandatory ) {
_isSingleton = isSingleton;
_isMandatory = isMandatory;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public boolean isSingleton() {
return _isSingleton;
}
/**
* {@inheritDoc}
*/
@Override
public boolean isMandatory() {
return _isMandatory;
}
/**
* Retrieves all {@link InstanceMode} elements representing a singleton.
*
* @return The singleton elements.
*/
public static InstanceMetrics[] toSingletonModes() {
final Set theModes = new HashSet<>();
for ( InstanceMode eMode : values() ) {
if ( eMode.isSingleton() ) {
theModes.add( eMode );
}
}
return theModes.toArray( new InstanceMode[theModes.size()] );
}
/**
* Retrieves all {@link InstanceMode} elements representing a required
* instances.
*
* @return The required instances elements.
*/
public static InstanceMetrics[] toRequiredModes() {
final Set theModes = new HashSet<>();
for ( InstanceMode eMode : values() ) {
if ( eMode.isMandatory() ) {
theModes.add( eMode );
}
}
return theModes.toArray( new InstanceMode[theModes.size()] );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy