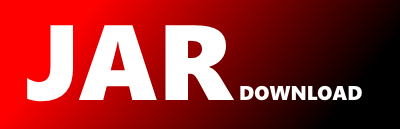
org.refcodes.decoupling.DependencyBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-decoupling Show documentation
Show all versions of refcodes-decoupling Show documentation
Artifact for breaking up dependencies between components or modules of
a software system (dependency injection and inversion of control).
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.decoupling;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashSet;
import java.util.function.BiFunction;
import java.util.function.Function;
import org.refcodes.decoupling.ClaimsAccessor.ClaimsBuilder;
import org.refcodes.decoupling.ClaimsAccessor.ClaimsProperty;
import org.refcodes.decoupling.InstanceMetricsAccessor.InstanceMetricsBuilder;
import org.refcodes.decoupling.InstanceMetricsAccessor.InstanceMetricsProperty;
import org.refcodes.decoupling.ProfilesAccessor.ProfilesBuilder;
import org.refcodes.decoupling.ProfilesAccessor.ProfilesProperty;
import org.refcodes.decoupling.TagsAccessor.TagsBuilder;
import org.refcodes.decoupling.TagsAccessor.TagsProperty;
import org.refcodes.factory.Factory;
import org.refcodes.factory.Initializer;
import org.refcodes.mixin.AliasAccessor.AliasBuilder;
import org.refcodes.mixin.AliasAccessor.AliasProperty;
import org.refcodes.mixin.InstanceAccessor.InstanceBuilder;
import org.refcodes.mixin.InstanceAccessor.InstanceProperty;
import org.refcodes.mixin.TypeAccessor.TypeBuilder;
import org.refcodes.mixin.TypeAccessor.TypeProperty;
import org.refcodes.textual.CaseStyleBuilder;
/**
* A {@link DependencyBuilder} describes a component or module depending on or
* required by other components or modules.
*
* @param the generic type of the {@link Dependency}.
*/
public class DependencyBuilder extends Dependency implements AliasProperty, AliasBuilder>, TypeProperty, TypeBuilder>, InstanceProperty, InstanceBuilder>, ProfilesProperty, ProfilesBuilder>, TagsProperty, TagsBuilder>, InstanceMetricsProperty, InstanceMetricsBuilder>, ClaimsProperty, ClaimsBuilder> {
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Empty constructor for creating an instance of the
* {@link DependencyBuilder} in which to apply the builder methods to set
* the desired state.
*/
protected DependencyBuilder() {}
/**
* {@inheritDoc}
*/
public DependencyBuilder( Class aType ) {
super( aType );
}
/**
* {@inheritDoc}
*/
public DependencyBuilder( T aInstance ) {
super( aInstance );
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public T getInstance() {
return _instances.size() != 0 ? _instances.iterator().next() : null;
}
/**
* Adds {@link Claim} for the given type and with the given alias to the
* {@link Claim} instances.
*
* @param aAlias The alias for the given {@link Claim}.
*
* @return True in case the {@link Claim} was added, false in case the
* {@link Claim} has already been added.
*/
public boolean addClaim( String aAlias ) {
return _claims.add( new Claim( aAlias ) );
}
/**
* Adds the given {@link Claim} to the {@link Claim} instances.
*
* @param aClaim the claim to be added.
*
* @return True in case the {@link Claim} was added, false in case the
* {@link Claim} has already been added.
*/
public boolean addClaim( Claim aClaim ) {
return _claims.add( aClaim );
}
/**
* Adds {@link Claim} for the given type to the {@link Claim} instances.
*
* @param aType the type of the claimed dependency.
*
* @return True in case the {@link Claim} was added, false in case the
* {@link Claim} has already been added.
*/
public boolean addClaim( Class> aType ) {
return _claims.add( new Claim( aType ) );
}
/**
* Adds {@link Claim} for the given type and with the given alias to the
* {@link Claim} instances.
*
* @param aType the type of the claimed dependency.
* @param aAlias The alias for the given {@link Claim}.
*
* @return True in case the {@link Claim} was added, false in case the
* {@link Claim} has already been added.
*/
public boolean addClaim( Class> aType, String aAlias ) {
return _claims.add( new Claim( aType, aAlias ) );
}
/**
* Adds the given profile to the profiles.
*
* @param aProfile The profile to add.
*
* @return True in case the profile was added, false in case the profile has
* already been added.
*/
public boolean addProfile( Object aProfile ) {
return _profiles.add( aProfile );
}
/**
* Adds the given tag to the tags.
*
* @param aTag The tag to add.
*
* @return True in case the tag was added, false in case the tag has already
* been added.
*/
public boolean addTag( Object aTag ) {
return _tags.add( aTag );
}
/**
* Sets the {@link InitializerClaim}, being a {@link Claim} dedicated to
* preprocess the {@link Dependency} after instantiation.
*
* @param The type of the {@link Dependency} being claimed by the
* {@link InitializerClaim}.
* @param aInitializer The setup configuring this {@link Dependency}'s
* instances.
*/
public void setInitializer( InitializerClaim aInitializer ) {
_initializer = aInitializer;
}
/**
* Sets the {@link InitializerClaim}, being a {@link Claim} dedicated to
* preprocess the {@link Dependency} after instantiation.
*
* @param The type of the {@link Dependency} being required by the
* {@link InitializerClaim}.
* @param aDependency The according claim required by the
* {@link InitializerClaim}.
* @param aInitializer The setup configuring this {@link Dependency}'s
* instances.
*/
public void setInitializer( Class aDependency, BiFunction aInitializer ) {
_initializer = new InitializerClaim<>( aDependency, aInitializer );
}
/**
* Sets the {@link InitializerClaim}, being a {@link Claim} dedicated to
* preprocess the {@link Dependency} after instantiation.
*
* @param The type of the {@link Dependency} being claimed by the
* {@link InitializerClaim}.
* @param aDependency The according claim required by the
* {@link InitializerClaim}.
* @param aInitializer The setup configuring this {@link Dependency}'s
* instances.
* @param aAlias the alias for the {@link InitializerClaim} to be added.
*/
public void setInitializer( Class aDependency, BiFunction aInitializer, String aAlias ) {
_initializer = new InitializerClaim<>( aDependency, aInitializer, aAlias );
}
/**
* Sets the {@link InitializerClaim}, being a {@link Claim} dedicated to
* preprocess the {@link Dependency} after instantiation. The according
* {@link InitializerClaim} must implement the {@link Initializer}
* functional interface for setting up this {@link Dependency}'s instances.
*
* @param The type of the {@link Dependency} being claimed by the
* {@link InitializerClaim}.
* @param aFactory The factory setting up this {@link Dependency}'s
* instance.
*/
public > void setInitializer( Class aFactory ) {
setInitializer( aFactory, ( t, i ) -> i.initialize( t ) );
}
/**
* Sets the {@link InitializerClaim}, being a {@link Claim} dedicated to
* preprocess the {@link Dependency} after instantiation. The according
* {@link InitializerClaim} must implement the {@link Initializer}
* functional interface for setting up this {@link Dependency}'s instances.
*
* @param The type of the {@link Dependency} being claimed by the
* {@link InitializerClaim}.
* @param aFactory The factory setting up this {@link Dependency}'s
* instance.
* @param aAlias the alias for the {@link InitializerClaim} to be added.
*/
public > void setInitializer( Class aFactory, String aAlias ) {
setInitializer( aFactory, ( t, i ) -> i.initialize( t ), aAlias );
}
/**
* Sets the {@link FactoryClaim}, being a {@link Claim} dedicated to
* fabricate (create) the {@link Dependency}'s instance.
*
* @param The type of the {@link Dependency} being claimed by the
* {@link FactoryClaim}.
* @param aFactory The {@link FactoryClaim} instance used to create
* (fabricate) the {@link Dependency}'s instance.
*/
public void setFactory( FactoryClaim aFactory ) {
_factory = aFactory;
}
/**
* Sets the {@link FactoryClaim}, being a {@link Claim} dedicated to
* fabricate (create) the {@link Dependency}'s instance.
*
* @param The type of the {@link Dependency} being claimed by the
* {@link FactoryClaim}.
* @param aDependency The according claim required by the
* {@link FactoryClaim}.
* @param aFactory The factory used to create (fabricate) the
* {@link Dependency}'s instance.
*/
public void setFactory( Class aDependency, Function aFactory ) {
_factory = new FactoryClaim<>( aDependency, aFactory );
}
/**
* Sets the {@link FactoryClaim}, being a {@link Claim} dedicated to
* fabricate (create) the {@link Dependency}'s instance.
*
* @param The type of the {@link Dependency} being claimed by the
* {@link FactoryClaim}.
* @param aDependency The according claim required by the
* {@link FactoryClaim}.
* @param aFactory The factory setting up this {@link Dependency}'s
* instance.
* @param aAlias the alias for the {@link FactoryClaim} to be added.
*/
public void setFactory( Class aDependency, Function aFactory, String aAlias ) {
_factory = new FactoryClaim<>( aDependency, aFactory, aAlias );
}
/**
* Sets the {@link FactoryClaim}, being a {@link Claim} dedicated to
* fabricate (create) the {@link Dependency}'s instance.
*
* @param The type of the {@link Dependency} being claimed by the
* {@link FactoryClaim}.
* @param aFactory The factory setting up this {@link Dependency}'s
* instance.
*/
public > void setFactory( Class aFactory ) {
setFactory( aFactory, Factory::create );
}
/**
* Sets the {@link FactoryClaim}, being a {@link Claim} dedicated to
* fabricate (create) the {@link Dependency}'s instance.
*
* @param The type of the {@link Dependency} being claimed by the
* {@link FactoryClaim}.
* @param aFactory The factory setting up this {@link Dependency}'s
* instance.
* @param aAlias the alias for the {@link FactoryClaim} to be added.
*/
public > void setFactory( Class aFactory, String aAlias ) {
setFactory( aFactory, Factory::create, aAlias );
}
/**
* {@inheritDoc}
*/
@Override
public void setAlias( String aAlias ) {
_alias = aAlias;
}
/**
* {@inheritDoc}
*/
@Override
public void setInstance( T aInstance ) {
super.setInstance( aInstance );
}
/**
* {@inheritDoc}
*/
@Override
public void setInstanceMetrics( InstanceMetrics aInstanceMetrics ) {
_instanceMetrics = aInstanceMetrics;
}
/**
* Sets the {@link InstanceMode} which describes how an instance for a
* {@link Dependency} is managed.
*
* @param aInstanceMode The according {@link InstanceMode}.
*/
public void setInstanceMetrics( InstanceMode aInstanceMode ) {
_instanceMetrics = aInstanceMode;
}
/**
* {@inheritDoc}
*/
@Override
public void setProfiles( Object[] aProfiles ) {
aProfiles = aProfiles != null ? aProfiles : new Object[] {};
_profiles = new HashSet<>( Arrays.asList( aProfiles ) );
}
/**
* Sets the profiles assigned to the {@link DependencyBuilder} instance.
*
* @param aProfiles The profiles to be assigned.
*/
public void setProfiles( Collection> aProfiles ) {
_profiles = new HashSet<>( aProfiles );
}
/**
* {@inheritDoc}
*/
@Override
public void setTags( Object[] aTags ) {
aTags = aTags != null ? aTags : new Object[] {};
_tags = new HashSet<>( Arrays.asList( aTags ) );
}
/**
* Sets the tags assigned to the {@link DependencyBuilder} instance.
*
* @param aTags The tags to be assigned.
*/
public void setTags( Collection> aTags ) {
_tags = new HashSet<>( aTags );
}
/**
* {@inheritDoc}
*/
@Override
public void setClaims( Claim[] aClaims ) {
aClaims = aClaims != null ? aClaims : new Claim[] {};
_claims = new HashSet<>( Arrays.asList( aClaims ) );
}
/**
* Sets the {@link Claim} instances to the {@link DependencyBuilder}
* instance.
*
* @param aClaims The {@link Claim} instances to be assigned.
*/
public void setClaims( Collection aClaims ) {
_claims = new HashSet<>( aClaims );
}
/**
* {@inheritDoc}
*/
@Override
public void setType( Class aType ) {
if ( aType == null ) {
throw new IllegalArgumentException( "The provided type must not(!) be null!" );
}
_type = aType;
if ( _alias == null && _alias.isEmpty() ) {
_alias = CaseStyleBuilder.asCamelCase( _type.getSimpleName() );
}
}
/**
* Builder method for the according {@link #addClaim(Claim)} method.
*
* @param aClaim the {@link Claim} to be added.
*
* @return This instance as of the builder pattern with additions to finish
* building the current {@link Claim}.
*/
public DependencyBuilder withAddClaim( Claim aClaim ) {
addClaim( aClaim );
return this;
}
/**
* Builder method for the according {@link #addClaim(Claim)} method.
*
* @param aAlias the alias for the {@link Claim} to be added.
*
* @return This instance as of the builder pattern with additions to finish
* building the current {@link Claim}.
*/
public DependencyBuilder withAddClaim( String aAlias ) {
addClaim( new Claim( aAlias ) );
return this;
}
/**
* Builder method for the according {@link #addClaim(Class)} method.
*
* @param aType the type of the claimed dependency.
*
* @return This instance as of the builder pattern with additions to finish
* building the current {@link Claim}.
*/
public DependencyBuilder withAddClaim( Class> aType ) {
addClaim( new Claim( aType ) );
return this;
}
/**
* Builder method for the according {@link #addClaim(Class, String)} method.
*
* @param aType the type of the claimed dependency.
* @param aAlias The alias of the given {@link Claim}.
*
* @return This instance as of the builder pattern with additions to finish
* building the current {@link Claim}.
*/
public DependencyBuilder withAddClaim( Class> aType, String aAlias ) {
addClaim( new Claim( aType, aAlias ) );
return this;
}
/**
* Builder method for adding a single profile.
*
* @param aProfile The profile to be added.
*
* @return This instance as of the builder pattern.
*/
public DependencyBuilder withAddProfile( Object aProfile ) {
_profiles.add( aProfile );
return this;
}
/**
* Builder method for adding a single tag.
*
* @param aTag The tag to be added.
*
* @return This instance as of the builder pattern.
*/
public DependencyBuilder withAddTag( Object aTag ) {
_tags.add( aTag );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public DependencyBuilder withAlias( String aAlias ) {
setAlias( aAlias );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public DependencyBuilder withInstance( T aInstance ) {
setInstance( aInstance );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public DependencyBuilder withInstanceMetrics( InstanceMetrics aInstanceMetrics ) {
setInstanceMetrics( aInstanceMetrics );
return this;
}
/**
* Builder method for the {@link #setInstanceMetrics(InstanceMode)} method.
*
* @param aInstanceMode The according {@link InstanceMode}.
*
* @return This instance as of the builder pattern.
*/
public DependencyBuilder withInstanceMetrics( InstanceMode aInstanceMode ) {
setInstanceMetrics( aInstanceMode );
return this;
}
/**
* Builder method for the {@link #setProfiles(Object[])} method.
*
* @param aProfiles The profiles to be assigned.
*
* @return This instance as of the builder pattern.
*/
public DependencyBuilder withProfiles( Collection> aProfiles ) {
setProfiles( aProfiles );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public DependencyBuilder withProfiles( Object... aProfiles ) {
setProfiles( aProfiles );
return this;
}
/**
* Builder method for the {@link #setTags(Object[])} method.
*
* @param aTags The tags to be assigned.
*
* @return This instance as of the builder pattern.
*/
public DependencyBuilder withTags( Collection> aTags ) {
setTags( aTags );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public DependencyBuilder withTags( Object... aTags ) {
setTags( aTags );
return this;
}
/**
* Builder method for the {@link #setClaims(Claim[])} method.
*
* @param aClaims The {@link Claim} instances to be assigned.
*
* @return This instance as of the builder pattern.
*/
public DependencyBuilder withClaims( Collection aClaims ) {
setClaims( aClaims );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public DependencyBuilder withClaims( Claim... aClaims ) {
setClaims( aClaims );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public DependencyBuilder withType( Class aType ) {
setType( aType );
return this;
}
/**
* Builder method for the {@link #setInitializer(InitializerClaim)} method.
*
* @param The type of the {@link Dependency} being claimed by the
* {@link InitializerClaim}.
* @param aInitializer The setup configuring this {@link Dependency}'s
* instances.
*
* @return This instance as of the builder pattern.
*/
public DependencyBuilder withInitializer( InitializerClaim aInitializer ) {
setInitializer( aInitializer );
return this;
}
/**
* Builder method for the {@link #setInitializer(Class, BiFunction)} method.
*
* @param The type of the {@link Dependency} being claimed by the
* {@link InitializerClaim}.
* @param aDependency The according dependency required by the
* {@link InitializerClaim}.
* @param aInitializer The setup configuring this {@link Dependency}'s
* instances.
*
* @return This instance as of the builder pattern.
*/
public DependencyBuilder withInitializer( Class aDependency, BiFunction aInitializer ) {
setInitializer( aDependency, aInitializer );
return this;
}
/**
* Builder method for the {@link #setInitializer(Class, BiFunction, String)}
* method.
*
* @param The type of the {@link Dependency} being claimed by the
* {@link InitializerClaim}.
* @param aDependency The according claim required by the
* {@link InitializerClaim}.
* @param aInitializer The setup configuring this {@link Dependency}'s
* instances.
* @param aAlias the alias for the {@link InitializerClaim} to be added.
*
* @return This instance as of the builder pattern.
*/
public DependencyBuilder withInitializer( Class aDependency, BiFunction aInitializer, String aAlias ) {
setInitializer( aDependency, aInitializer, aAlias );
return this;
}
/**
* Builder method for the {@link #setInitializer(Class)} method.
*
* @param The type of the {@link Dependency} being claimed by the
* {@link InitializerClaim}.
* @param aInitializer The setup configuring this {@link Dependency}'s
* instances.
*
* @return This instance as of the builder pattern.
*/
public > DependencyBuilder withInitializer( Class aInitializer ) {
setInitializer( aInitializer );
return this;
}
/**
* Builder method for the {@link #setInitializer(Class, String)} method.
*
* @param The type of the {@link Dependency} being claimed by the
* {@link InitializerClaim}.
* @param aInitializer The setup configuring this {@link Dependency}'s
* instances.
* @param aAlias the alias for the {@link InitializerClaim} to be added.
*
* @return This instance as of the builder pattern.
*/
public > DependencyBuilder withInitializer( Class aInitializer, String aAlias ) {
setInitializer( aInitializer, aAlias );
return this;
}
/**
* Builder method for the {@link #setFactory(FactoryClaim)} method.
*
* @param The type of the {@link Dependency} being required by the
* {@link FactoryClaim}.
* @param aFactory The {@link FactoryClaim} instance used for setting up the
* {@link Dependency}.
*
* @return This instance as of the builder pattern.
*/
public DependencyBuilder withFactory( FactoryClaim aFactory ) {
setFactory( aFactory );
return this;
}
/**
* Builder method for the {@link #setFactory(Class, Function)} method.
*
* @param The type of the {@link Dependency} being claimed by the
* {@link FactoryClaim}.
* @param aDependency The according dependency required by the
* {@link FactoryClaim}.
* @param aFactory The factory fabricating (creating) this
* {@link Dependency}'s instances using the provided dependency.
*
* @return This instance as of the builder pattern.
*/
public DependencyBuilder withFactory( Class aDependency, Function aFactory ) {
setFactory( aDependency, aFactory );
return this;
}
/**
* Builder method for the {@link #setFactory(Class, Function, String)}
* method.
*
* @param The type of the {@link Dependency} being claimed by the
* {@link FactoryClaim}.
* @param aDependency The according dependency required by the
* {@link FactoryClaim}.
* @param aFactory The factory fabricating (creating) this
* {@link Dependency}'s instances using the provided dependency.
* @param aAlias the alias for the {@link FactoryClaim} to be added.
*
* @return This instance as of the builder pattern.
*/
public DependencyBuilder withFactory( Class aDependency, Function aFactory, String aAlias ) {
setFactory( aDependency, aFactory, aAlias );
return this;
}
/**
* Builder method for the {@link #setFactory(Class)} method.
*
* @param The type of the {@link Dependency} being claimed by the
* {@link FactoryClaim}.
* @param aFactory The factory setting up this {@link Dependency}'s
* instance.
*
* @return This instance as of the builder pattern.
*/
public > DependencyBuilder withFactory( Class aFactory ) {
setFactory( aFactory );
return this;
}
/**
* Builder method for the {@link #setFactory(Class, String)} method.
*
* @param The type of the {@link Dependency} being claimed by the
* {@link FactoryClaim}.
* @param aFactory The factory setting up this {@link Dependency}'s
* instance.
* @param aAlias the alias for the {@link FactoryClaim} to be added.
*
* @return This instance as of the builder pattern.
*/
public > DependencyBuilder withFactory( Class aFactory, String aAlias ) {
setFactory( aFactory, aAlias );
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy