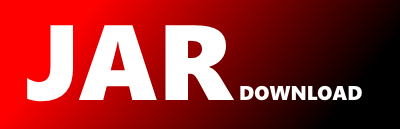
org.refcodes.eventbus.EventBusMatcherByDeclaration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-eventbus Show documentation
Show all versions of refcodes-eventbus Show documentation
Artifact providing observer pattern based event-bus functionality.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// =============================================================================
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// =============================================================================
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// together with the GPL linking exception applied; as being applied by the GNU
// Classpath ("http://www.gnu.org/software/classpath/license.html")
// =============================================================================
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// =============================================================================
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.eventbus;
import org.refcodes.matcher.Matcher;
import org.refcodes.matcher.MatcherDeclaration;
import org.refcodes.observer.ActionEvent;
import org.refcodes.observer.EventMetaData;
import org.refcodes.observer.MetaDataActionEvent;
import org.refcodes.observer.MetaDataEvent;
public class EventBusMatcherByDeclaration {
/**
* Factory method to create an event matcher by event type.
*
* @param aEventType The event type to be matched by this matcher.
*
* @return An event matcher by event type.
*/
public static EventBusMatcher isAssignableFrom( Class> aEventType ) {
Matcher> theAssignableFrom = MatcherDeclaration.isAssignableFrom( aEventType );
return new EventBusMatcherWrapper( theAssignableFrom );
}
/**
* Factory method to create an event matcher by event publisher type.
*
* @param > The type of the event to be matched
* @param The publisher descriptor type
*
* @param aPublisherType The event publisher type to be matched by this
* matcher.
*
* @return An event matcher by event type.
*/
public static , PT extends Object> EventBusMatcher publisherIsAssignableFrom( Class extends PT> aPublisherType ) {
return new PublisherIsAssignableFromMatcherImpl( aPublisherType );
}
/**
* Factory method to create an "OR" matcher for the given matchers.
*
* @param aEventMatchers The matchers to be combined by an "OR".
*
* @return An "OR" matcher.
*/
@SafeVarargs
public static EventBusMatcher or( EventBusMatcher... aEventMatchers ) {
return new EventBusMatcherWrapper( MatcherDeclaration.or( aEventMatchers ) );
}
/**
* Factory method to create an "AND" matcher for the given matchers.
*
* @param > The event type applied to the matcher
*
* @param aEventMatchers The matchers to be combined by an "AND".
*
* @return An "AND" matcher.
*/
@SafeVarargs
public static EventBusMatcher and( EventBusMatcher... aEventMatchers ) {
return new EventBusMatcherWrapper( MatcherDeclaration.and( aEventMatchers ) );
}
/**
* Factory method to create an "EQUAL WITH" matcher for the given name
* compared with the name stored in the {@link EventMetaData}.
*
* @param > The event type applied to the matcher
*
* @param aName The name to be compared with a {@link MetaDataEvent}'s
* {@link EventMetaData}'s name property.
*
* @return An "EQUAL WITH" matcher regarding the {@link MetaDataEvent}'s
* name property.
*/
public static EventBusMatcher nameEqualWith( String aName ) {
return new NameEqualWithMatcherImpl( aName );
}
/**
* Factory method to create an "EQUAL WITH" matcher for the given group
* compared with the group stored in the {@link EventMetaData}.
*
* @param > The event type applied to the matcher
*
* @param aGroup The group to be compared with a {@link MetaDataEvent}'s
* {@link EventMetaData}'s group property.
*
* @return An "EQUAL WITH" matcher regarding the {@link MetaDataEvent}'s
* group property.
*/
public static EventBusMatcher groupEqualWith( String aGroup ) {
return new GroupEqualWithMatcherImpl( aGroup );
}
/**
* Factory method to create an "EQUAL WITH" matcher for the given channel
* compared with the channel stored in the {@link EventMetaData}.
*
* @param > The event type applied to the matcher
*
* @param aChannel The channel to be compared with a {@link MetaDataEvent}'s
* {@link EventMetaData}'s channel property.
*
* @return An "EQUAL WITH" matcher regarding the {@link MetaDataEvent}'s
* channel property.
*/
public static EventBusMatcher channelEqualWith( String aChannel ) {
return new ChannelEqualWithMatcherImpl( aChannel );
}
/**
* Factory method to create an "EQUAL WITH" matcher for the given UID
* compared with the UID stored in the {@link EventMetaData}.
*
* @param > The event type applied to the matcher
*
* @param aUniversalId The UID to be compared with a {@link MetaDataEvent}'s
* {@link EventMetaData}'s UID property.
*
* @return An "EQUAL WITH" matcher regarding the {@link MetaDataEvent}'s UID
* property.
*/
public static EventBusMatcher universalIdEqualWith( String aUniversalId ) {
return new UniversalIdEqualWithMatcherImpl( aUniversalId );
}
/**
* Factory method to create an "EQUAL WITH" matcher for the given action
* compared with the action stored in the {@link EventMetaData}.
*
* @param > The event type applied to the matcher.
*
* @param The type of the action stored in the event. CAUTION: The
* drawback of not using generic generic type declaration on a class
* level is no granted type safety, the advantage is the ease of use:
* Sub-classes can be used out of the box.
*
* @param aAction The action to be compared with a {@link MetaDataEvent}'s
* {@link EventMetaData}'s action property.
*
* @return An "EQUAL WITH" matcher regarding the {@link ActionEvent}'s
* action property.
*/
public static , A> EventBusMatcher actionEqualWith( A aAction ) {
return new ActionEqualWithMatcherImpl( aAction );
}
// -------------------------------------------------------------------------
// INNER CLASSES:
// -------------------------------------------------------------------------
/**
* Wraps an event matcher to by of the correct type.
*/
private static class EventBusMatcherWrapper implements EventBusMatcher {
private Matcher> _eventMatcher;
/**
* Wraps the given event matcher to be of the correct type.
*
* @param aMatcher the event matcher in question.
*/
public EventBusMatcherWrapper( Matcher> aMatcher ) {
assert (aMatcher != null);
_eventMatcher = aMatcher;
}
@Override
public boolean isMatching( MetaDataEvent> aEvent ) {
return _eventMatcher.isMatching( aEvent );
}
}
/**
* A basic implementation of an event matcher.
*
* @param > The event type
*/
private static class PublisherIsAssignableFromMatcherImpl, PT extends Object> implements EventBusMatcher {
private Class extends PT> _eventPublisherType;
public PublisherIsAssignableFromMatcherImpl( Class extends PT> aEventPublisherType ) {
_eventPublisherType = aEventPublisherType;
}
@Override
public boolean isMatching( MetaDataEvent> aEvent ) {
assert (aEvent != null);
if ( _eventPublisherType != null ) {
if ( !aEvent.getMetaData().getPublisherType().isAssignableFrom( _eventPublisherType ) ) { return false; }
}
return true;
}
}
/**
* A basic implementation of an event matcher.
*
* @param > The event type
*/
private static class NameEqualWithMatcherImpl implements EventBusMatcher {
private String _name;
public NameEqualWithMatcherImpl( String aName ) {
_name = aName;
}
@Override
public boolean isMatching( MetaDataEvent> aEvent ) {
assert (aEvent != null);
if ( _name != null ) {
return (_name.equals( aEvent.getMetaData().getName() ));
}
else if ( aEvent.getMetaData().getName() == null ) { return true; }
return false;
}
}
/**
* A basic implementation of an event matcher.
*
* @param > The event type
*/
private static class GroupEqualWithMatcherImpl implements EventBusMatcher {
private String _group;
public GroupEqualWithMatcherImpl( String aGroup ) {
_group = aGroup;
}
@Override
public boolean isMatching( MetaDataEvent> aEvent ) {
assert (aEvent != null);
if ( _group != null ) {
return (_group.equals( aEvent.getMetaData().getGroup() ));
}
else if ( aEvent.getMetaData().getGroup() == null ) { return true; }
return false;
}
}
/**
* A basic implementation of an event matcher.
*
* @param > The event type
*/
private static class ChannelEqualWithMatcherImpl implements EventBusMatcher {
private String _channel;
public ChannelEqualWithMatcherImpl( String aChannel ) {
_channel = aChannel;
}
@Override
public boolean isMatching( MetaDataEvent> aEvent ) {
assert (aEvent != null);
if ( _channel != null ) {
return (_channel.equals( aEvent.getMetaData().getChannel() ));
}
else if ( aEvent.getMetaData().getChannel() == null ) { return true; }
return false;
}
}
/**
* A basic implementation of an event matcher.
*
* @param > The event type
*/
private static class UniversalIdEqualWithMatcherImpl implements EventBusMatcher {
private String _universalId;
public UniversalIdEqualWithMatcherImpl( String aUniversalId ) {
_universalId = aUniversalId;
}
@Override
public boolean isMatching( MetaDataEvent> aEvent ) {
assert (aEvent != null);
if ( _universalId != null ) {
return (_universalId.equals( aEvent.getMetaData().getUniversalId() ));
}
else if ( aEvent.getMetaData().getUniversalId() == null ) { return true; }
return false;
}
}
/**
* A basic implementation of an event matcher.
*
* @param > The event type
*/
private static class ActionEqualWithMatcherImpl implements EventBusMatcher {
private Object _action;
public ActionEqualWithMatcherImpl( A aAction ) {
_action = aAction;
}
@Override
public boolean isMatching( MetaDataEvent> aEvent ) {
assert (aEvent != null);
if ( !(aEvent instanceof MetaDataActionEvent, ?>) ) return false;
MetaDataActionEvent, ?> theEvent = (MetaDataActionEvent, ?>) aEvent;
if ( _action != null ) {
return (_action.equals( theEvent.getAction() ));
}
else if ( theEvent.getAction() == null ) { return true; }
return false;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy