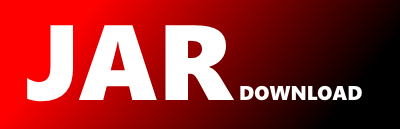
org.refcodes.filesystem.ChangeRootFileSystemWrapperImpl Maven / Gradle / Ivy
Show all versions of refcodes-filesystem Show documentation
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// =============================================================================
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// =============================================================================
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// together with the GPL linking exception applied; as being applied by the GNU
// Classpath ("http://www.gnu.org/software/classpath/license.html")
// =============================================================================
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// =============================================================================
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.filesystem;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.ArrayList;
import java.util.List;
import org.refcodes.filesystem.FileHandle.MutableFileHandle;
/**
* The change root space wrapper for a given {@link FileSystem} relocates the
* paths accessed by an application to the given namespace. This is helpful in
* case an application is to use a {@link FileSystem} sand box. Only the files
* below that name space are visible by the file system.
*
* ATTENTION: Make sure that the {@link FileSystem} cannot jail break its sand
* box by using relative paths with ".." !!!
*/
public class ChangeRootFileSystemWrapperImpl implements FileSystem {
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private String _namespace;
private FileSystem _fileSystem;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Instantiates a new change root file system wrapper impl.
*
* @param aNamespace the namespace
* @param aFileSystem the file system
*/
public ChangeRootFileSystemWrapperImpl( String aNamespace, FileSystem aFileSystem ) {
_namespace = aNamespace;
_fileSystem = aFileSystem;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public boolean hasFile( String aKey ) throws IllegalKeyException, NoListAccessException, UnknownFileSystemException, IOException {
aKey = FileSystemUtility.toNormalizedKey( aKey, this );
aKey = _namespace + PATH_DELIMITER + aKey;
return _fileSystem.hasFile( aKey );
}
/**
* {@inheritDoc}
*/
@Override
public boolean hasFile( String aPath, String aName ) throws IllegalPathException, IllegalNameException, NoListAccessException, UnknownFileSystemException, IOException {
aPath = FileSystemUtility.toNormalizedPath( aPath, this );
aName = FileSystemUtility.toNormalizedName( aName, this );
aPath = _namespace + PATH_DELIMITER + aPath;
return _fileSystem.hasFile( aPath, aName );
}
/**
* {@inheritDoc}
*/
@Override
public boolean hasFile( FileHandle aFileHandle ) throws NoListAccessException, UnknownFileSystemException, IOException, IllegalFileHandleException {
aFileHandle = FileSystemUtility.toNormalizedFileHandle( aFileHandle, this );
MutableFileHandle theMutableFileHandle = aFileHandle.toMutableFileHandle();
theMutableFileHandle.setPath( toRealPath( aFileHandle ) );
return _fileSystem.hasFile( theMutableFileHandle.toFileHandle() );
}
/**
* {@inheritDoc}
*/
@Override
public FileHandle createFile( String aKey ) throws FileAlreadyExistsException, NoCreateAccessException, IllegalKeyException, UnknownFileSystemException, IOException, NoListAccessException {
aKey = FileSystemUtility.toNormalizedKey( aKey, this );
aKey = _namespace + PATH_DELIMITER + aKey;
FileHandle theFileHandle = _fileSystem.createFile( aKey );
MutableFileHandle theMutableFileHandle = theFileHandle.toMutableFileHandle();
theMutableFileHandle.setPath( toVirtualPath( theFileHandle ) );
return theMutableFileHandle.toFileHandle();
}
/**
* {@inheritDoc}
*/
@Override
public FileHandle createFile( String aPath, String aName ) throws FileAlreadyExistsException, NoCreateAccessException, IllegalNameException, IllegalPathException, UnknownFileSystemException, IOException, NoListAccessException {
aPath = FileSystemUtility.toNormalizedPath( aPath, this );
aName = FileSystemUtility.toNormalizedPath( aName, this );
aPath = _namespace + PATH_DELIMITER + aPath;
FileHandle theFileHandle = _fileSystem.createFile( aPath, aName );
MutableFileHandle theMutableFileHandle = theFileHandle.toMutableFileHandle();
theMutableFileHandle.setPath( toVirtualPath( theFileHandle ) );
return theMutableFileHandle.toFileHandle();
}
/**
* {@inheritDoc}
*/
@Override
public FileHandle getFileHandle( String aKey ) throws NoListAccessException, IllegalKeyException, UnknownFileSystemException, IOException, UnknownKeyException {
aKey = FileSystemUtility.toNormalizedKey( aKey, this );
aKey = _namespace + PATH_DELIMITER + aKey;
FileHandle theFileHandle = _fileSystem.getFileHandle( aKey );
MutableFileHandle theMutableFileHandle = theFileHandle.toMutableFileHandle();
theMutableFileHandle.setPath( toVirtualPath( theFileHandle ) );
return theMutableFileHandle.toFileHandle();
}
/**
* {@inheritDoc}
*/
@Override
public FileHandle getFileHandle( String aPath, String aName ) throws NoListAccessException, IllegalNameException, IllegalPathException, UnknownFileSystemException, IOException, UnknownKeyException {
aPath = FileSystemUtility.toNormalizedPath( aPath, this );
aName = FileSystemUtility.toNormalizedName( aName, this );
aPath = _namespace + PATH_DELIMITER + aPath;
FileHandle theFileHandle = _fileSystem.getFileHandle( aPath, aName );
MutableFileHandle theMutableFileHandle = theFileHandle.toMutableFileHandle();
theMutableFileHandle.setPath( toVirtualPath( theFileHandle ) );
return theMutableFileHandle.toFileHandle();
}
/**
* {@inheritDoc}
*/
@Override
public void fromFile( FileHandle aFromFileHandle, OutputStream aOutputStream ) throws ConcurrentAccessException, UnknownFileException, NoReadAccessException, UnknownFileSystemException, IOException, NoListAccessException, IllegalFileHandleException {
aFromFileHandle = FileSystemUtility.toNormalizedFileHandle( aFromFileHandle, this );
MutableFileHandle theMutableFromFileHandle = aFromFileHandle.toMutableFileHandle();
theMutableFromFileHandle.setPath( toRealPath( aFromFileHandle ) );
_fileSystem.fromFile( theMutableFromFileHandle.toFileHandle(), aOutputStream );
}
/**
* {@inheritDoc}
*/
@Override
public void toFile( FileHandle aToFileHandle, InputStream aInputStream ) throws ConcurrentAccessException, UnknownFileException, NoWriteAccessException, UnknownFileSystemException, IOException, NoListAccessException, IllegalFileHandleException {
aToFileHandle = FileSystemUtility.toNormalizedFileHandle( aToFileHandle, this );
MutableFileHandle theMutableToFileHandle = aToFileHandle.toMutableFileHandle();
theMutableToFileHandle.setPath( toRealPath( aToFileHandle ) );
_fileSystem.toFile( theMutableToFileHandle.toFileHandle(), aInputStream );
}
/**
* {@inheritDoc}
*/
@Override
public InputStream fromFile( FileHandle aFromFileHandle ) throws ConcurrentAccessException, UnknownFileException, UnknownFileException, NoReadAccessException, UnknownFileSystemException, IOException, NoListAccessException, IllegalFileHandleException {
aFromFileHandle = FileSystemUtility.toNormalizedFileHandle( aFromFileHandle, this );
MutableFileHandle theMutableFromFileHandle = aFromFileHandle.toMutableFileHandle();
theMutableFromFileHandle.setPath( toRealPath( aFromFileHandle ) );
return _fileSystem.fromFile( theMutableFromFileHandle.toFileHandle() );
}
/**
* {@inheritDoc}
*/
@Override
public OutputStream toFile( FileHandle aToFileHandle ) throws ConcurrentAccessException, UnknownFileException, NoWriteAccessException, UnknownFileSystemException, IOException, IllegalFileHandleException {
aToFileHandle = FileSystemUtility.toNormalizedFileHandle( aToFileHandle, this );
MutableFileHandle theMutableToFileHandle = aToFileHandle.toMutableFileHandle();
theMutableToFileHandle.setPath( toRealPath( aToFileHandle ) );
return _fileSystem.toFile( theMutableToFileHandle.toFileHandle() );
}
/**
* {@inheritDoc}
*/
@Override
public void fromFile( FileHandle aFromFileHandle, File aToFile ) throws ConcurrentAccessException, UnknownFileException, NoReadAccessException, UnknownFileSystemException, IOException, NoListAccessException, IllegalFileHandleException {
aFromFileHandle = FileSystemUtility.toNormalizedFileHandle( aFromFileHandle, this );
MutableFileHandle theMutableFromFileHandle = aFromFileHandle.toMutableFileHandle();
theMutableFromFileHandle.setPath( toRealPath( aFromFileHandle ) );
_fileSystem.fromFile( theMutableFromFileHandle.toFileHandle(), aToFile );
}
/**
* {@inheritDoc}
*/
@Override
public void toFile( FileHandle aToFileHandle, File aFromFile ) throws ConcurrentAccessException, UnknownFileException, NoWriteAccessException, UnknownFileSystemException, IOException, NoListAccessException, IllegalFileHandleException {
aToFileHandle = FileSystemUtility.toNormalizedFileHandle( aToFileHandle, this );
MutableFileHandle theMutableToFileHandle = aToFileHandle.toMutableFileHandle();
theMutableToFileHandle.setPath( toRealPath( aToFileHandle ) );
_fileSystem.toFile( theMutableToFileHandle.toFileHandle(), aFromFile );
}
/**
* {@inheritDoc}
*/
@Override
public void toFile( FileHandle aToFileHandle, byte[] aBuffer ) throws ConcurrentAccessException, UnknownFileException, NoWriteAccessException, UnknownFileSystemException, IOException, NoListAccessException, IllegalFileHandleException {
aToFileHandle = FileSystemUtility.toNormalizedFileHandle( aToFileHandle, this );
MutableFileHandle theMutableToFileHandle = aToFileHandle.toMutableFileHandle();
theMutableToFileHandle.setPath( toRealPath( aToFileHandle ) );
_fileSystem.toFile( theMutableToFileHandle.toFileHandle(), aBuffer );
}
/**
* {@inheritDoc}
*/
@Override
public FileHandle renameFile( FileHandle aFileHandle, String aNewName ) throws UnknownFileException, ConcurrentAccessException, FileAlreadyExistsException, NoCreateAccessException, NoDeleteAccessException, IllegalNameException, UnknownFileSystemException, IOException, NoListAccessException, IllegalFileHandleException {
aFileHandle = FileSystemUtility.toNormalizedFileHandle( aFileHandle, this );
MutableFileHandle theMutableFileHandle = aFileHandle.toMutableFileHandle();
theMutableFileHandle.setPath( toRealPath( aFileHandle ) );
FileHandle theNewFileHandle = _fileSystem.renameFile( theMutableFileHandle.toFileHandle(), aNewName );
MutableFileHandle theMutableNewFileHandle = theNewFileHandle.toMutableFileHandle();
theMutableNewFileHandle.setPath( toVirtualPath( theNewFileHandle ) );
return theMutableNewFileHandle.toFileHandle();
}
/**
* {@inheritDoc}
*/
@Override
public FileHandle moveFile( FileHandle aFileHandle, String aNewKey ) throws UnknownFileException, ConcurrentAccessException, FileAlreadyExistsException, NoCreateAccessException, NoDeleteAccessException, IllegalKeyException, UnknownFileSystemException, IOException, NoListAccessException, IllegalFileHandleException {
aFileHandle = FileSystemUtility.toNormalizedFileHandle( aFileHandle, this );
MutableFileHandle theMutableFileHandle = aFileHandle.toMutableFileHandle();
theMutableFileHandle.setPath( toRealPath( aFileHandle ) );
aNewKey = _namespace + PATH_DELIMITER + aNewKey;
FileHandle theNewFileHandle = _fileSystem.moveFile( theMutableFileHandle.toFileHandle(), aNewKey );
MutableFileHandle theMutableNewFileHandle = theNewFileHandle.toMutableFileHandle();
theMutableNewFileHandle.setPath( toVirtualPath( theNewFileHandle ) );
return theMutableNewFileHandle.toFileHandle();
}
/**
* {@inheritDoc}
*/
@Override
public void deleteFile( FileHandle aFileHandle ) throws ConcurrentAccessException, UnknownFileException, NoDeleteAccessException, UnknownFileSystemException, IOException, NoListAccessException, IllegalFileHandleException {
aFileHandle = FileSystemUtility.toNormalizedFileHandle( aFileHandle, this );
MutableFileHandle theMutableFileHandle = aFileHandle.toMutableFileHandle();
theMutableFileHandle.setPath( toRealPath( aFileHandle ) );
_fileSystem.deleteFile( theMutableFileHandle.toFileHandle() );
}
/**
* {@inheritDoc}
*/
@Override
public boolean hasFiles( String aPath, boolean isRecursively ) throws NoListAccessException, IllegalPathException, UnknownFileSystemException, IOException {
aPath = FileSystemUtility.toNormalizedPath( aPath, this );
aPath = _namespace + PATH_DELIMITER + aPath;
return _fileSystem.hasFiles( aPath, isRecursively );
}
/**
* {@inheritDoc}
*/
@Override
public List getFileHandles( String aPath, boolean isRecursively ) throws NoListAccessException, UnknownPathException, IllegalPathException, UnknownFileSystemException, IOException {
aPath = FileSystemUtility.toNormalizedPath( aPath, this );
aPath = _namespace + PATH_DELIMITER + aPath;
List theFileHandles = _fileSystem.getFileHandles( aPath, isRecursively );
List theFoundFileHandles = new ArrayList();
MutableFileHandle eMutableFileHandle;
for ( FileHandle eFileHandle : theFileHandles ) {
eMutableFileHandle = eFileHandle.toMutableFileHandle();
eMutableFileHandle.setPath( toVirtualPath( eFileHandle ) );
theFoundFileHandles.add( eMutableFileHandle.toFileHandle() );
}
return theFoundFileHandles;
}
/**
* {@inheritDoc}
*/
@Override
public void destroy() {
if ( _fileSystem != null ) {
_fileSystem.destroy();
}
}
// /////////////////////////////////////////////////////////////////////////
// HELPER:
// /////////////////////////////////////////////////////////////////////////
/**
* Removes the namespace from the {@link FileHandle}'s path.
*
* @param aFileHandle The {@link FileHandle} from which to remove the
* namespace. This is the file handle used by the virtual file
* system.
*
* @return The path from the provided {@link FileHandle} without the
* namespace.
*/
private String toVirtualPath( FileHandle aFileHandle ) {
return aFileHandle.getPath().substring( (_namespace + PATH_DELIMITER).length() );
}
/**
* Adds the namespace to the {@link FileHandle}'s path.
*
* @param aFileHandle The {@link FileHandle} to which to add the namespace.
* This is the file handle used by the real file system.
*
* @return The path from the provided {@link FileHandle} with the namespace
* prepended.
*/
private String toRealPath( FileHandle aFileHandle ) {
return _namespace + PATH_DELIMITER + aFileHandle.getPath();
}
}