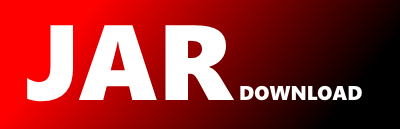
org.refcodes.filesystem.FileHandle Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-filesystem Show documentation
Show all versions of refcodes-filesystem Show documentation
Artifact for the refcodes virtual file system design.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// =============================================================================
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// =============================================================================
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// together with the GPL linking exception applied; as being applied by the GNU
// Classpath ("http://www.gnu.org/software/classpath/license.html")
// =============================================================================
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// =============================================================================
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.filesystem;
import java.util.Date;
import org.refcodes.mixin.CreatedDateAccessor;
import org.refcodes.mixin.ModifiedDateAccessor;
import org.refcodes.mixin.NameAccessor;
import org.refcodes.mixin.PathAccessor;
/**
* A file (handle) (descriptor) describes a file in a file system.
*/
public interface FileHandle extends PathAccessor, NameAccessor, FileSizeAccessor, CreatedDateAccessor, ModifiedDateAccessor {
/**
* The path is the part of the key without the name. The path separator is
* not implementation specific and should be retrieved from the file
* system's {@link FileSystem#PATH_DELIMITER} attribute.
*
* @return The path of the file's key without the name.
*/
@Override
String getPath();
/**
* The name is the part of the key without the path. The path separator is
* not implementation specific and should be retrieved from the file
* system's {@link FileSystem#PATH_DELIMITER} attribute.
*
* @return The name of the file's key without the path.
*/
@Override
String getName();
/**
* The key is the fully qualified name to identify the file. The key usually
* is physically (directory path and filename) or virtually composed of the
* path and the name.
*
* @return The fully qualified key of the file.
*/
String toKey();
/**
* The size of the content of the file.
*
* @return The content size of the file.
*/
@Override
long getFileSize();
/**
* The date when the file was created.
*
* @return The creation date.
*/
@Override
Date getCreatedDate();
/**
* The date when the file was modified.
*
* @return The modification date.
*/
@Override
Date getModifiedDate();
/**
* Converts the give {@link FileHandle} to a {@link MutableFileHandle}. The
* mutable {@link FileHandle} allows the modification of (fiddling around
* with) attributes.
* -------------------------------------------------------------------------
* ATTENTION: Usually fiddling around with attributes is not necessary, as
* the {@link FileSystem} itself provides the sufficient functionality to
* work with files. In some cases though this might be necessary: This
* method is being provided to allow modification of file attributes while
* making sure that the {@link FileHandle} itself creates a copy so that any
* additional attributes provided by extensions of this interface of whom
* the developer does not know (yet) are preserved. So extensions of the
* {@link FileHandle} know how to create a {@link MutableFileHandle} without
* information loss, the business logic does not require to take care of any
* yet unknown extensions.
* -------------------------------------------------------------------------
* CAUTION: Working with modified {@link FileHandle}s on the
* {@link FileSystem} can aCause unexpected (severe) behavior (data loss),
* so we assume that you know what you do when using the
* {@link MutableFileHandle}!
* -------------------------------------------------------------------------
* Use {@link MutableFileHandle#toFileHandle()} to get back to a
* {@link FileHandle} to avoid hassle with collections, the
* {@link Object#hashCode()} and the {@link Object#equals(Object)}
* operations.
*
* @return the mutable file handle
*/
MutableFileHandle toMutableFileHandle();
/**
* The mutable {@link FileHandle} allows the modification of (fiddling
* around with) attributes.
* -------------------------------------------------------------------------
* ATTENTION: Usually fiddling around with attributes is not necessary, as
* the {@link FileSystem} itself provides the sufficient functionality to
* work with files. In some cases though this might be necessary: This class
* is being provided to allow modification of file attributes while making
* sure that the {@link FileHandle} itself creates a copy so that any
* additional attributes provided by extensions of this interface of whom
* the developer does not know (yet) are preserved. So extensions of the
* {@link FileHandle} know how to create a {@link MutableFileHandle} without
* information loss, the business logic does not require to take care of any
* yet unknown extensions.
* -------------------------------------------------------------------------
* CAUTION: Working with modified {@link FileHandle}s on the
* {@link FileSystem} can aCause unexpected (severe) behavior (data loss),
* so we assume that you know what you do when using the
* {@link MutableFileHandle}!
*/
public interface MutableFileHandle extends FileHandle, PathProperty, NameProperty, FileSizeProperty, CreatedDateProperty, ModifiedDateProperty {
/**
* Converts the {@link MutableFileHandle} back to a {@link FileHandle}
* to avoid hassle with collections, the {@link Object#hashCode()} and
* the {@link Object#equals(Object)} operations.
*
* @return An immutable {@link FileHandle} from this
* {@link MutableFileHandle}.
*/
FileHandle toFileHandle();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy